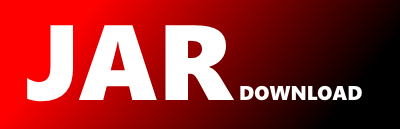
com.liferay.petra.function.transform.TransformUtil Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2000-present Liferay, Inc. All rights reserved.
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
package com.liferay.petra.function.transform;
import com.liferay.petra.function.UnsafeFunction;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
* @author Brian Wing Shun Chan
*/
public class TransformUtil {
public static List transform(
Collection collection, UnsafeFunction unsafeFunction) {
try {
return unsafeTransform(collection, unsafeFunction);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static R[] transform(
T[] array, UnsafeFunction unsafeFunction, Class> clazz) {
try {
return unsafeTransform(array, unsafeFunction, clazz);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static R[] transformToArray(
Collection collection, UnsafeFunction unsafeFunction,
Class> clazz) {
try {
return unsafeTransformToArray(collection, unsafeFunction, clazz);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static int[] transformToIntArray(
Collection collection, UnsafeFunction unsafeFunction) {
try {
return unsafeTransformToIntArray(collection, unsafeFunction);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static List transformToList(
int[] array, UnsafeFunction unsafeFunction) {
try {
return unsafeTransformToList(array, unsafeFunction);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static List transformToList(
long[] array, UnsafeFunction unsafeFunction) {
try {
return unsafeTransformToList(array, unsafeFunction);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static List transformToList(
T[] array, UnsafeFunction unsafeFunction) {
try {
return unsafeTransformToList(array, unsafeFunction);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static long[] transformToLongArray(
Collection collection, UnsafeFunction unsafeFunction) {
try {
return unsafeTransformToLongArray(collection, unsafeFunction);
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
}
public static List unsafeTransform(
Collection collection, UnsafeFunction unsafeFunction)
throws E {
if (collection == null) {
return new ArrayList<>();
}
List list = new ArrayList<>(collection.size());
for (T item : collection) {
R newItem = unsafeFunction.apply(item);
if (newItem != null) {
list.add(newItem);
}
}
return list;
}
public static R[] unsafeTransform(
T[] array, UnsafeFunction unsafeFunction, Class> clazz)
throws E {
List list = unsafeTransformToList(array, unsafeFunction);
return list.toArray((R[])Array.newInstance(clazz, 0));
}
public static R[] unsafeTransformToArray(
Collection collection, UnsafeFunction unsafeFunction,
Class> clazz)
throws E {
List list = unsafeTransform(collection, unsafeFunction);
return list.toArray((R[])Array.newInstance(clazz, 0));
}
public static int[] unsafeTransformToIntArray(
Collection collection, UnsafeFunction unsafeFunction)
throws E {
return (int[])_unsafeTransformToPrimitiveArray(
collection, unsafeFunction, int[].class);
}
public static List unsafeTransformToList(
int[] array, UnsafeFunction unsafeFunction)
throws E {
if (array == null) {
return new ArrayList<>();
}
List list = new ArrayList<>(array.length);
for (Integer item : array) {
R newItem = unsafeFunction.apply(item);
if (newItem != null) {
list.add(newItem);
}
}
return list;
}
public static List unsafeTransformToList(
long[] array, UnsafeFunction unsafeFunction)
throws E {
if (array == null) {
return new ArrayList<>();
}
List list = new ArrayList<>(array.length);
for (Long item : array) {
R newItem = unsafeFunction.apply(item);
if (newItem != null) {
list.add(newItem);
}
}
return list;
}
public static List unsafeTransformToList(
T[] array, UnsafeFunction unsafeFunction)
throws E {
if (array == null) {
return new ArrayList<>();
}
List list = new ArrayList<>(array.length);
for (T item : array) {
R newItem = unsafeFunction.apply(item);
if (newItem != null) {
list.add(newItem);
}
}
return list;
}
public static long[] unsafeTransformToLongArray(
Collection collection, UnsafeFunction unsafeFunction)
throws E {
return (long[])_unsafeTransformToPrimitiveArray(
collection, unsafeFunction, long[].class);
}
private static Object
_unsafeTransformToPrimitiveArray(
Collection collection,
UnsafeFunction unsafeFunction, Class> clazz)
throws E {
List list = unsafeTransform(collection, unsafeFunction);
Object array = clazz.cast(
Array.newInstance(clazz.getComponentType(), list.size()));
for (int i = 0; i < list.size(); i++) {
Array.set(array, i, list.get(i));
}
return array;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy