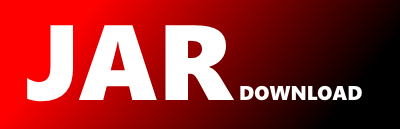
com.liferay.wiki.navigation.web.internal.util.MenuItem Maven / Gradle / Ivy
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
package com.liferay.wiki.navigation.web.internal.util;
import com.liferay.petra.string.StringPool;
import com.liferay.portal.kernel.log.Log;
import com.liferay.portal.kernel.log.LogFactoryUtil;
import com.liferay.portal.kernel.util.GetterUtil;
import com.liferay.portal.kernel.util.Http;
import com.liferay.wiki.model.WikiNode;
import com.liferay.wiki.model.WikiPage;
import com.liferay.wiki.navigation.web.internal.util.constants.WikiNavigationConstants;
import com.liferay.wiki.service.WikiPageServiceUtil;
import java.io.Serializable;
import java.util.LinkedList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.portlet.PortletURL;
/**
* @author Thiago Moreira
* @author Peter Shin
*/
public class MenuItem implements Serializable {
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy