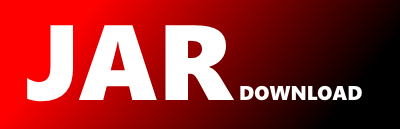
com.lightningkite.lightningdb.LargeTestModelFields.kt Maven / Gradle / Ivy
@file:UseContextualSerialization(UUID::class, Instant::class)
@file:OptIn(ExperimentalSerializationApi::class, InternalSerializationApi::class)
package com.lightningkite.lightningdb
import com.lightningkite.khrysalis.SharedCode
import kotlinx.serialization.Contextual
import kotlinx.serialization.Serializable
import kotlinx.serialization.UseContextualSerialization
import java.time.Instant
import java.util.*
import com.lightningkite.lightningdb.*
import kotlin.reflect.*
import kotlinx.serialization.*
import kotlinx.serialization.builtins.*
import kotlinx.serialization.internal.GeneratedSerializer
import java.time.*
fun prepareLargeTestModelFields() {
LargeTestModel::_id.setCopyImplementation { original, value -> original.copy(_id = value) }
LargeTestModel::boolean.setCopyImplementation { original, value -> original.copy(boolean = value) }
LargeTestModel::byte.setCopyImplementation { original, value -> original.copy(byte = value) }
LargeTestModel::short.setCopyImplementation { original, value -> original.copy(short = value) }
LargeTestModel::int.setCopyImplementation { original, value -> original.copy(int = value) }
LargeTestModel::long.setCopyImplementation { original, value -> original.copy(long = value) }
LargeTestModel::float.setCopyImplementation { original, value -> original.copy(float = value) }
LargeTestModel::double.setCopyImplementation { original, value -> original.copy(double = value) }
LargeTestModel::char.setCopyImplementation { original, value -> original.copy(char = value) }
LargeTestModel::string.setCopyImplementation { original, value -> original.copy(string = value) }
LargeTestModel::instant.setCopyImplementation { original, value -> original.copy(instant = value) }
LargeTestModel::list.setCopyImplementation { original, value -> original.copy(list = value) }
LargeTestModel::listEmbedded.setCopyImplementation { original, value -> original.copy(listEmbedded = value) }
LargeTestModel::set.setCopyImplementation { original, value -> original.copy(set = value) }
LargeTestModel::setEmbedded.setCopyImplementation { original, value -> original.copy(setEmbedded = value) }
LargeTestModel::map.setCopyImplementation { original, value -> original.copy(map = value) }
LargeTestModel::embedded.setCopyImplementation { original, value -> original.copy(embedded = value) }
LargeTestModel::booleanNullable.setCopyImplementation { original, value -> original.copy(booleanNullable = value) }
LargeTestModel::byteNullable.setCopyImplementation { original, value -> original.copy(byteNullable = value) }
LargeTestModel::shortNullable.setCopyImplementation { original, value -> original.copy(shortNullable = value) }
LargeTestModel::intNullable.setCopyImplementation { original, value -> original.copy(intNullable = value) }
LargeTestModel::longNullable.setCopyImplementation { original, value -> original.copy(longNullable = value) }
LargeTestModel::floatNullable.setCopyImplementation { original, value -> original.copy(floatNullable = value) }
LargeTestModel::doubleNullable.setCopyImplementation { original, value -> original.copy(doubleNullable = value) }
LargeTestModel::charNullable.setCopyImplementation { original, value -> original.copy(charNullable = value) }
LargeTestModel::stringNullable.setCopyImplementation { original, value -> original.copy(stringNullable = value) }
LargeTestModel::instantNullable.setCopyImplementation { original, value -> original.copy(instantNullable = value) }
LargeTestModel::listNullable.setCopyImplementation { original, value -> original.copy(listNullable = value) }
LargeTestModel::mapNullable.setCopyImplementation { original, value -> original.copy(mapNullable = value) }
LargeTestModel::embeddedNullable.setCopyImplementation { original, value -> original.copy(embeddedNullable = value) }
}
val DataClassPath._id: DataClassPath get() = this[LargeTestModel::_id]
val DataClassPath.boolean: DataClassPath get() = this[LargeTestModel::boolean]
val DataClassPath.byte: DataClassPath get() = this[LargeTestModel::byte]
val DataClassPath.short: DataClassPath get() = this[LargeTestModel::short]
val DataClassPath.int: DataClassPath get() = this[LargeTestModel::int]
val DataClassPath.long: DataClassPath get() = this[LargeTestModel::long]
val DataClassPath.float: DataClassPath get() = this[LargeTestModel::float]
val DataClassPath.double: DataClassPath get() = this[LargeTestModel::double]
val DataClassPath.char: DataClassPath get() = this[LargeTestModel::char]
val DataClassPath.string: DataClassPath get() = this[LargeTestModel::string]
val DataClassPath.instant: DataClassPath get() = this[LargeTestModel::instant]
val DataClassPath.list: DataClassPath> get() = this[LargeTestModel::list]
val DataClassPath.listEmbedded: DataClassPath> get() = this[LargeTestModel::listEmbedded]
val DataClassPath.set: DataClassPath> get() = this[LargeTestModel::set]
val DataClassPath.setEmbedded: DataClassPath> get() = this[LargeTestModel::setEmbedded]
val DataClassPath.map: DataClassPath> get() = this[LargeTestModel::map]
val DataClassPath.embedded: DataClassPath get() = this[LargeTestModel::embedded]
val DataClassPath.booleanNullable: DataClassPath get() = this[LargeTestModel::booleanNullable]
val DataClassPath.byteNullable: DataClassPath get() = this[LargeTestModel::byteNullable]
val DataClassPath.shortNullable: DataClassPath get() = this[LargeTestModel::shortNullable]
val DataClassPath.intNullable: DataClassPath get() = this[LargeTestModel::intNullable]
val DataClassPath.longNullable: DataClassPath get() = this[LargeTestModel::longNullable]
val DataClassPath.floatNullable: DataClassPath get() = this[LargeTestModel::floatNullable]
val DataClassPath.doubleNullable: DataClassPath get() = this[LargeTestModel::doubleNullable]
val DataClassPath.charNullable: DataClassPath get() = this[LargeTestModel::charNullable]
val DataClassPath.stringNullable: DataClassPath get() = this[LargeTestModel::stringNullable]
val DataClassPath.instantNullable: DataClassPath get() = this[LargeTestModel::instantNullable]
val DataClassPath.listNullable: DataClassPath?> get() = this[LargeTestModel::listNullable]
val DataClassPath.mapNullable: DataClassPath?> get() = this[LargeTestModel::mapNullable]
val DataClassPath.embeddedNullable: DataClassPath get() = this[LargeTestModel::embeddedNullable]
inline val LargeTestModel.Companion.path: DataClassPath get() = path()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy