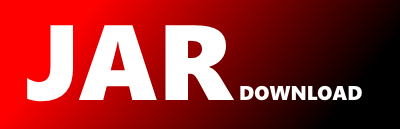
com.lightningkite.rx.okhttp.HttpResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okhttp-jackson Show documentation
Show all versions of okhttp-jackson Show documentation
An OkHttp wrapper based on RxJava using Jackson for JSON
The newest version!
package com.lightningkite.rx.okhttp
import com.fasterxml.jackson.core.type.TypeReference
import com.fasterxml.jackson.module.kotlin.jacksonTypeRef
import com.fasterxml.jackson.module.kotlin.readValue
import io.reactivex.rxjava3.core.Single
import io.reactivex.rxjava3.core.SingleEmitter
import okhttp3.Response
import java.lang.reflect.ParameterizedType
import java.lang.reflect.Type
/**
* Closes the body of the response as a [Single].
*/
fun Response.discard(): Single = Single.create { em ->
body!!.close()
em.onSuccess(Unit)
}.let { HttpClient.threadCorrectly(it) }
/**
* Reads the body of the response as a [Single].
*/
fun Response.readText(): Single =
Single.create { em -> em.onSuccess(body!!.use { it.string() }) }.let { HttpClient.threadCorrectly(it) }
/**
* Reads the body of the response as a [Single].
*/
fun Response.readByteArray(): Single =
Single.create { em -> em.onSuccess(body!!.use { it.bytes() }) }.let { HttpClient.threadCorrectly(it) }
/**
* Reads the body of the response as a [Single], using [defaultJsonMapper] to parse the JSON.
*/
inline fun Response.readJson(): Single = readJson(jacksonTypeRef())
/**
* Reads the body of the response as a [Single], using [defaultJsonMapper] to parse the JSON.
*/
fun Response.readJson(typeToken: TypeReference): Single = Single.create { em: SingleEmitter ->
try {
val result: T = body!!.use {
defaultJsonMapper.readValue(it.byteStream(), typeToken)
}
em.onSuccess(result)
} catch (e: Throwable) {
em.tryOnError(e)
}
}.let { HttpClient.threadCorrectly(it) }
/**
* Reads the body of the response as a [Single], using [defaultJsonMapper] to parse the JSON.
* Dumps the raw response to [System.out].
*/
inline fun Response.readJsonDebug(): Single = readJsonDebug(jacksonTypeRef())
/**
* Reads the body of the response as a [Single], using [defaultJsonMapper] to parse the JSON.
* Dumps the raw response to [System.out].
*/
fun Response.readJsonDebug(typeToken: TypeReference): Single = readText().map { println("HttpResponse got $it"); it.fromJsonString(typeToken) }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy