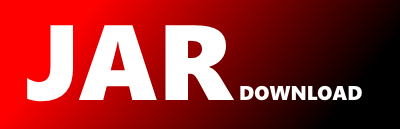
com.lightningkite.rx.okhttp.RxHttpAssist.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okhttp-jackson Show documentation
Show all versions of okhttp-jackson Show documentation
An OkHttp wrapper based on RxJava using Jackson for JSON
The newest version!
package com.lightningkite.rx.okhttp
import com.fasterxml.jackson.core.type.TypeReference
import com.fasterxml.jackson.module.kotlin.jacksonTypeRef
import com.fasterxml.jackson.module.kotlin.readValue
import io.reactivex.rxjava3.core.Single
import okhttp3.Response
import java.lang.reflect.ParameterizedType
/**
* Changes unsuccessful responses into [HttpResponseException].
*/
fun Single.unsuccessfulAsError(): Single {
return this.map { it ->
if (it.isSuccessful) {
return@map it
} else {
throw HttpResponseException(it)
}
}
}
/**
* Properly discards the response.
*/
fun Single.discard(): Single {
return this.flatMap {
if (it.isSuccessful) {
it.discard()
} else {
Single.error(HttpResponseException(it)) as Single
}
}
}
/**
* Reads the response into JSON using the [defaultJsonMapper].
*/
inline fun Single.readJson(): Single {
val type = jacksonTypeRef()
return this.flatMap { it ->
if (it.isSuccessful) {
it.readJson(type)
} else {
Single.error(HttpResponseException(it)) as Single
}
}
}
/**
* Reads the response into JSON using the [defaultJsonMapper], but prints the raw value to [System.out].
*/
inline fun Single.readJsonDebug(): Single {
val type = jacksonTypeRef()
return this.flatMap { it ->
if (it.isSuccessful) {
it.readJsonDebug(type)
} else {
Single.error(HttpResponseException(it)) as Single
}
}
}
/**
* Reads the text from the [Response].
*/
fun Single.readText(): Single {
return this.flatMap { it ->
if (it.isSuccessful) {
it.readText()
} else {
Single.error(HttpResponseException(it))
}
}
}
/**
* Reads the binary data from the [Response].
*/
fun Single.readByteArray(): Single {
return this.flatMap { it ->
if (it.isSuccessful) {
it.readByteArray()
} else {
Single.error(HttpResponseException(it))
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy