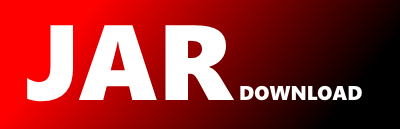
io.opentelemetry.sdk.metrics.export.IntervalMetricReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lightstep-opentelemetry-auto-exporter Show documentation
Show all versions of lightstep-opentelemetry-auto-exporter Show documentation
Lightstep OpenTelemetry Auto Exporter
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy