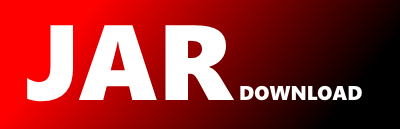
io.opentelemetry.sdk.trace.export.SimpleSpanProcessor Maven / Gradle / Ivy
/*
* Copyright 2019, OpenTelemetry Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.opentelemetry.sdk.trace.export;
import com.google.common.annotations.VisibleForTesting;
import io.opentelemetry.sdk.common.export.ConfigBuilder;
import io.opentelemetry.sdk.trace.ReadableSpan;
import io.opentelemetry.sdk.trace.SpanProcessor;
import io.opentelemetry.sdk.trace.data.SpanData;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* An implementation of the {@link SpanProcessor} that converts the {@link ReadableSpan} to {@link
* SpanData} and passes it to the configured exporter.
*
* Configuration options for {@link SimpleSpanProcessor} can be read from system properties,
* environment variables, or {@link java.util.Properties} objects.
*
*
For system properties and {@link java.util.Properties} objects, {@link SimpleSpanProcessor}
* will look for the following names:
*
*
* - {@code otel.ssp.export.sampled}: sets whether only sampled spans should be exported.
*
*
* For environment variables, {@link SimpleSpanProcessor} will look for the following names:
*
*
* - {@code OTEL_SSP_EXPORT_SAMPLED}: sets whether only sampled spans should be exported.
*
*/
public final class SimpleSpanProcessor implements SpanProcessor {
private static final Logger logger = Logger.getLogger(SimpleSpanProcessor.class.getName());
private final SpanExporter spanExporter;
private final boolean sampled;
private SimpleSpanProcessor(SpanExporter spanExporter, boolean sampled) {
this.spanExporter = Objects.requireNonNull(spanExporter, "spanExporter");
this.sampled = sampled;
}
@Override
public void onStart(ReadableSpan span) {
// Do nothing.
}
@Override
public boolean isStartRequired() {
return false;
}
@Override
public void onEnd(ReadableSpan span) {
if (sampled && !span.getSpanContext().getTraceFlags().isSampled()) {
return;
}
try {
List spans = Collections.singletonList(span.toSpanData());
spanExporter.export(spans);
} catch (Throwable e) {
logger.log(Level.WARNING, "Exception thrown by the export.", e);
}
}
@Override
public boolean isEndRequired() {
return true;
}
@Override
public void shutdown() {
spanExporter.shutdown();
}
@Override
public void forceFlush() {
// Do nothing.
}
/**
* Returns a new Builder for {@link SimpleSpanProcessor}.
*
* @param spanExporter the {@code SpanExporter} to where the Spans are pushed.
* @return a new {@link SimpleSpanProcessor}.
* @throws NullPointerException if the {@code spanExporter} is {@code null}.
*/
public static Builder newBuilder(SpanExporter spanExporter) {
return new Builder(spanExporter);
}
/** Builder class for {@link SimpleSpanProcessor}. */
public static final class Builder extends ConfigBuilder {
private static final String KEY_SAMPLED = "otel.ssp.export.sampled";
@VisibleForTesting static final boolean DEFAULT_EXPORT_ONLY_SAMPLED = true;
private final SpanExporter spanExporter;
private boolean exportOnlySampled = DEFAULT_EXPORT_ONLY_SAMPLED;
private Builder(SpanExporter spanExporter) {
this.spanExporter = Objects.requireNonNull(spanExporter, "spanExporter");
}
/**
* Sets the configuration values from the given configuration map for only the available keys.
* This method looks for the following keys:
*
*
* - {@code otel.ssp.export.sampled}: to set whether only sampled spans should be exported.
*
*
* @param configMap {@link Map} holding the configuration values.
* @return this.
*/
@Override
protected Builder fromConfigMap(
Map configMap, NamingConvention namingConvention) {
configMap = namingConvention.normalize(configMap);
Boolean boolValue = getBooleanProperty(KEY_SAMPLED, configMap);
if (boolValue != null) {
return this.setExportOnlySampled(boolValue);
}
return this;
}
/**
* Set whether only sampled spans should be exported.
*
* Default value is {@code true}.
*
* @param exportOnlySampled if {@code true} report only sampled spans.
* @return this.
*/
public Builder setExportOnlySampled(boolean exportOnlySampled) {
this.exportOnlySampled = exportOnlySampled;
return this;
}
@VisibleForTesting
boolean getExportOnlySampled() {
return exportOnlySampled;
}
// TODO: Add metrics for total exported spans.
// TODO: Consider to add support for constant Attributes and/or Resource.
/**
* Returns a new {@link SimpleSpanProcessor} that converts spans to proto and forwards them to
* the given {@code spanExporter}.
*
* @return a new {@link SimpleSpanProcessor}.
* @throws NullPointerException if the {@code spanExporter} is {@code null}.
*/
public SimpleSpanProcessor build() {
return new SimpleSpanProcessor(spanExporter, exportOnlySampled);
}
}
}