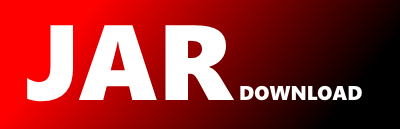
com.lightstep.tracer.shared.SimpleFuture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-common Show documentation
Show all versions of java-common Show documentation
The LightStep OpenTracing Tracer implementation for Java
package com.lightstep.tracer.shared;
/**
* Simple Future/Promise-like class.
*
* Acts as a simple wrapper on Object wait() / notify() - avoiding the
* complex future interfaces in Java 7/8.
*/
public class SimpleFuture {
private boolean resolved;
private T value;
@SuppressWarnings("WeakerAccess")
public SimpleFuture() {
resolved = false;
}
@SuppressWarnings("WeakerAccess")
public SimpleFuture(T value) {
this.value = value;
resolved = true;
}
public void set(T value) {
synchronized (this) {
this.value = value;
resolved = true;
notifyAll();
}
}
public T get() throws InterruptedException {
if (!resolved) {
synchronized (this) {
wait();
}
}
return value;
}
@SuppressWarnings({"unused", "WeakerAccess"})
public T getWithTimeout(long millis) throws InterruptedException {
if (!resolved) {
synchronized (this) {
wait(millis);
}
}
return value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy