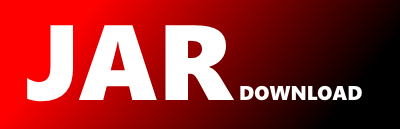
lightstep.com.google.api.ResourceDescriptor Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/api/resource.proto
package lightstep.lightstep.lightstep.com.google.api;
/**
*
* A simple descriptor of a resource type.
* ResourceDescriptor annotates a resource message (either by means of a
* protobuf annotation or use in the service config), and associates the
* resource's schema, the resource type, and the pattern of the resource name.
* Example:
* message Topic {
* // Indicates this message defines a resource schema.
* // Declares the resource type in the format of {service}/{kind}.
* // For Kubernetes resources, the format is {api group}/{kind}.
* option (google.api.resource) = {
* type: "pubsub.googleapis.com/Topic"
* name_descriptor: {
* pattern: "projects/{project}/topics/{topic}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* }
* };
* }
* The ResourceDescriptor Yaml config will look like:
* resources:
* - type: "pubsub.googleapis.com/Topic"
* name_descriptor:
* - pattern: "projects/{project}/topics/{topic}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* Sometimes, resources have multiple patterns, typically because they can
* live under multiple parents.
* Example:
* message LogEntry {
* option (google.api.resource) = {
* type: "logging.googleapis.com/LogEntry"
* name_descriptor: {
* pattern: "projects/{project}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* }
* name_descriptor: {
* pattern: "folders/{folder}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
* parent_name_extractor: "folders/{folder}"
* }
* name_descriptor: {
* pattern: "organizations/{organization}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Organization"
* parent_name_extractor: "organizations/{organization}"
* }
* name_descriptor: {
* pattern: "billingAccounts/{billing_account}/logs/{log}"
* parent_type: "billing.googleapis.com/BillingAccount"
* parent_name_extractor: "billingAccounts/{billing_account}"
* }
* };
* }
* The ResourceDescriptor Yaml config will look like:
* resources:
* - type: 'logging.googleapis.com/LogEntry'
* name_descriptor:
* - pattern: "projects/{project}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* - pattern: "folders/{folder}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
* parent_name_extractor: "folders/{folder}"
* - pattern: "organizations/{organization}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Organization"
* parent_name_extractor: "organizations/{organization}"
* - pattern: "billingAccounts/{billing_account}/logs/{log}"
* parent_type: "billing.googleapis.com/BillingAccount"
* parent_name_extractor: "billingAccounts/{billing_account}"
* For flexible resources, the resource name doesn't contain parent names, but
* the resource itself has parents for policy evaluation.
* Example:
* message Shelf {
* option (google.api.resource) = {
* type: "library.googleapis.com/Shelf"
* name_descriptor: {
* pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* }
* name_descriptor: {
* pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
* }
* };
* }
* The ResourceDescriptor Yaml config will look like:
* resources:
* - type: 'library.googleapis.com/Shelf'
* name_descriptor:
* - pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* - pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
*
*
* Protobuf type {@code google.api.ResourceDescriptor}
*/
public final class ResourceDescriptor extends
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.api.ResourceDescriptor)
ResourceDescriptorOrBuilder {
private static final long serialVersionUID = 0L;
// Use ResourceDescriptor.newBuilder() to construct.
private ResourceDescriptor(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ResourceDescriptor() {
type_ = "";
pattern_ = lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringArrayList.EMPTY;
nameField_ = "";
history_ = 0;
plural_ = "";
singular_ = "";
}
@Override
public final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResourceDescriptor(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedInputStream input,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new NullPointerException();
}
int mutable_bitField0_ = 0;
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.UnknownFieldSet.Builder unknownFields =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
String s = input.readStringRequireUtf8();
type_ = s;
break;
}
case 18: {
String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
pattern_ = new lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
pattern_.add(s);
break;
}
case 26: {
String s = input.readStringRequireUtf8();
nameField_ = s;
break;
}
case 32: {
int rawValue = input.readEnum();
history_ = rawValue;
break;
}
case 42: {
String s = input.readStringRequireUtf8();
plural_ = s;
break;
}
case 50: {
String s = input.readStringRequireUtf8();
singular_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
pattern_ = pattern_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ResourceProto.internal_static_google_api_ResourceDescriptor_descriptor;
}
@Override
protected FieldAccessorTable
internalGetFieldAccessorTable() {
return ResourceProto.internal_static_google_api_ResourceDescriptor_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ResourceDescriptor.class, Builder.class);
}
/**
*
* A description of the historical or future-looking state of the
* resource pattern.
*
*
* Protobuf enum {@code google.api.ResourceDescriptor.History}
*/
public enum History
implements lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ProtocolMessageEnum {
/**
*
* The "unset" value.
*
*
* HISTORY_UNSPECIFIED = 0;
*/
HISTORY_UNSPECIFIED(0),
/**
*
* The resource originally had one pattern and launched as such, and
* additional patterns were added later.
*
*
* ORIGINALLY_SINGLE_PATTERN = 1;
*/
ORIGINALLY_SINGLE_PATTERN(1),
/**
*
* The resource has one pattern, but the API owner expects to add more
* later. (This is the inverse of ORIGINALLY_SINGLE_PATTERN, and prevents
* that from being necessary once there are multiple patterns.)
*
*
* FUTURE_MULTI_PATTERN = 2;
*/
FUTURE_MULTI_PATTERN(2),
UNRECOGNIZED(-1),
;
/**
*
* The "unset" value.
*
*
* HISTORY_UNSPECIFIED = 0;
*/
public static final int HISTORY_UNSPECIFIED_VALUE = 0;
/**
*
* The resource originally had one pattern and launched as such, and
* additional patterns were added later.
*
*
* ORIGINALLY_SINGLE_PATTERN = 1;
*/
public static final int ORIGINALLY_SINGLE_PATTERN_VALUE = 1;
/**
*
* The resource has one pattern, but the API owner expects to add more
* later. (This is the inverse of ORIGINALLY_SINGLE_PATTERN, and prevents
* that from being necessary once there are multiple patterns.)
*
*
* FUTURE_MULTI_PATTERN = 2;
*/
public static final int FUTURE_MULTI_PATTERN_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@Deprecated
public static History valueOf(int value) {
return forNumber(value);
}
public static History forNumber(int value) {
switch (value) {
case 0: return HISTORY_UNSPECIFIED;
case 1: return ORIGINALLY_SINGLE_PATTERN;
case 2: return FUTURE_MULTI_PATTERN;
default: return null;
}
}
public static lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Internal.EnumLiteMap<
History> internalValueMap =
new lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Internal.EnumLiteMap() {
public History findValueByNumber(int number) {
return History.forNumber(number);
}
};
public final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return ResourceDescriptor.getDescriptor().getEnumTypes().get(0);
}
private static final History[] VALUES = values();
public static History valueOf(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private History(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.api.ResourceDescriptor.History)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private volatile Object type_;
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public String getType() {
Object ref = type_;
if (ref instanceof String) {
return (String) ref;
} else {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getTypeBytes() {
Object ref = type_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
type_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
public static final int PATTERN_FIELD_NUMBER = 2;
private lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringList pattern_;
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ProtocolStringList
getPatternList() {
return pattern_;
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public int getPatternCount() {
return pattern_.size();
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public String getPattern(int index) {
return pattern_.get(index);
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getPatternBytes(int index) {
return pattern_.getByteString(index);
}
public static final int NAME_FIELD_FIELD_NUMBER = 3;
private volatile Object nameField_;
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public String getNameField() {
Object ref = nameField_;
if (ref instanceof String) {
return (String) ref;
} else {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
nameField_ = s;
return s;
}
}
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getNameFieldBytes() {
Object ref = nameField_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
nameField_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
public static final int HISTORY_FIELD_NUMBER = 4;
private int history_;
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public int getHistoryValue() {
return history_;
}
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public History getHistory() {
@SuppressWarnings("deprecation")
History result = History.valueOf(history_);
return result == null ? History.UNRECOGNIZED : result;
}
public static final int PLURAL_FIELD_NUMBER = 5;
private volatile Object plural_;
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public String getPlural() {
Object ref = plural_;
if (ref instanceof String) {
return (String) ref;
} else {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
plural_ = s;
return s;
}
}
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getPluralBytes() {
Object ref = plural_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
plural_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
public static final int SINGULAR_FIELD_NUMBER = 6;
private volatile Object singular_;
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public String getSingular() {
Object ref = singular_;
if (ref instanceof String) {
return (String) ref;
} else {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
singular_ = s;
return s;
}
}
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getSingularBytes() {
Object ref = singular_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
singular_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@Override
public void writeTo(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTypeBytes().isEmpty()) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.writeString(output, 1, type_);
}
for (int i = 0; i < pattern_.size(); i++) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.writeString(output, 2, pattern_.getRaw(i));
}
if (!getNameFieldBytes().isEmpty()) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.writeString(output, 3, nameField_);
}
if (history_ != History.HISTORY_UNSPECIFIED.getNumber()) {
output.writeEnum(4, history_);
}
if (!getPluralBytes().isEmpty()) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.writeString(output, 5, plural_);
}
if (!getSingularBytes().isEmpty()) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.writeString(output, 6, singular_);
}
unknownFields.writeTo(output);
}
@Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTypeBytes().isEmpty()) {
size += lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.computeStringSize(1, type_);
}
{
int dataSize = 0;
for (int i = 0; i < pattern_.size(); i++) {
dataSize += computeStringSizeNoTag(pattern_.getRaw(i));
}
size += dataSize;
size += 1 * getPatternList().size();
}
if (!getNameFieldBytes().isEmpty()) {
size += lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.computeStringSize(3, nameField_);
}
if (history_ != History.HISTORY_UNSPECIFIED.getNumber()) {
size += lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedOutputStream
.computeEnumSize(4, history_);
}
if (!getPluralBytes().isEmpty()) {
size += lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.computeStringSize(5, plural_);
}
if (!getSingularBytes().isEmpty()) {
size += lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.computeStringSize(6, singular_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@Override
public boolean equals(final Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ResourceDescriptor)) {
return super.equals(obj);
}
ResourceDescriptor other = (ResourceDescriptor) obj;
if (!getType()
.equals(other.getType())) return false;
if (!getPatternList()
.equals(other.getPatternList())) return false;
if (!getNameField()
.equals(other.getNameField())) return false;
if (history_ != other.history_) return false;
if (!getPlural()
.equals(other.getPlural())) return false;
if (!getSingular()
.equals(other.getSingular())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
if (getPatternCount() > 0) {
hash = (37 * hash) + PATTERN_FIELD_NUMBER;
hash = (53 * hash) + getPatternList().hashCode();
}
hash = (37 * hash) + NAME_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getNameField().hashCode();
hash = (37 * hash) + HISTORY_FIELD_NUMBER;
hash = (53 * hash) + history_;
hash = (37 * hash) + PLURAL_FIELD_NUMBER;
hash = (53 * hash) + getPlural().hashCode();
hash = (37 * hash) + SINGULAR_FIELD_NUMBER;
hash = (53 * hash) + getSingular().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ResourceDescriptor parseFrom(
java.nio.ByteBuffer data)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ResourceDescriptor parseFrom(
java.nio.ByteBuffer data,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ResourceDescriptor parseFrom(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString data)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ResourceDescriptor parseFrom(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString data,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ResourceDescriptor parseFrom(byte[] data)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ResourceDescriptor parseFrom(
byte[] data,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ResourceDescriptor parseFrom(java.io.InputStream input)
throws java.io.IOException {
return lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ResourceDescriptor parseFrom(
java.io.InputStream input,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ResourceDescriptor parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ResourceDescriptor parseDelimitedFrom(
java.io.InputStream input,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ResourceDescriptor parseFrom(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ResourceDescriptor parseFrom(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedInputStream input,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ResourceDescriptor prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@Override
protected Builder newBuilderForType(
BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A simple descriptor of a resource type.
* ResourceDescriptor annotates a resource message (either by means of a
* protobuf annotation or use in the service config), and associates the
* resource's schema, the resource type, and the pattern of the resource name.
* Example:
* message Topic {
* // Indicates this message defines a resource schema.
* // Declares the resource type in the format of {service}/{kind}.
* // For Kubernetes resources, the format is {api group}/{kind}.
* option (google.api.resource) = {
* type: "pubsub.googleapis.com/Topic"
* name_descriptor: {
* pattern: "projects/{project}/topics/{topic}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* }
* };
* }
* The ResourceDescriptor Yaml config will look like:
* resources:
* - type: "pubsub.googleapis.com/Topic"
* name_descriptor:
* - pattern: "projects/{project}/topics/{topic}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* Sometimes, resources have multiple patterns, typically because they can
* live under multiple parents.
* Example:
* message LogEntry {
* option (google.api.resource) = {
* type: "logging.googleapis.com/LogEntry"
* name_descriptor: {
* pattern: "projects/{project}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* }
* name_descriptor: {
* pattern: "folders/{folder}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
* parent_name_extractor: "folders/{folder}"
* }
* name_descriptor: {
* pattern: "organizations/{organization}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Organization"
* parent_name_extractor: "organizations/{organization}"
* }
* name_descriptor: {
* pattern: "billingAccounts/{billing_account}/logs/{log}"
* parent_type: "billing.googleapis.com/BillingAccount"
* parent_name_extractor: "billingAccounts/{billing_account}"
* }
* };
* }
* The ResourceDescriptor Yaml config will look like:
* resources:
* - type: 'logging.googleapis.com/LogEntry'
* name_descriptor:
* - pattern: "projects/{project}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* parent_name_extractor: "projects/{project}"
* - pattern: "folders/{folder}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
* parent_name_extractor: "folders/{folder}"
* - pattern: "organizations/{organization}/logs/{log}"
* parent_type: "cloudresourcemanager.googleapis.com/Organization"
* parent_name_extractor: "organizations/{organization}"
* - pattern: "billingAccounts/{billing_account}/logs/{log}"
* parent_type: "billing.googleapis.com/BillingAccount"
* parent_name_extractor: "billingAccounts/{billing_account}"
* For flexible resources, the resource name doesn't contain parent names, but
* the resource itself has parents for policy evaluation.
* Example:
* message Shelf {
* option (google.api.resource) = {
* type: "library.googleapis.com/Shelf"
* name_descriptor: {
* pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* }
* name_descriptor: {
* pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
* }
* };
* }
* The ResourceDescriptor Yaml config will look like:
* resources:
* - type: 'library.googleapis.com/Shelf'
* name_descriptor:
* - pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Project"
* - pattern: "shelves/{shelf}"
* parent_type: "cloudresourcemanager.googleapis.com/Folder"
*
*
* Protobuf type {@code google.api.ResourceDescriptor}
*/
public static final class Builder extends
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.api.ResourceDescriptor)
lightstep.lightstep.lightstep.com.google.api.ResourceDescriptorOrBuilder {
public static final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ResourceProto.internal_static_google_api_ResourceDescriptor_descriptor;
}
@Override
protected FieldAccessorTable
internalGetFieldAccessorTable() {
return ResourceProto.internal_static_google_api_ResourceDescriptor_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ResourceDescriptor.class, Builder.class);
}
// Construct using lightstep.lightstep.lightstep.com.google.api.ResourceDescriptor.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@Override
public Builder clear() {
super.clear();
type_ = "";
pattern_ = lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
nameField_ = "";
history_ = 0;
plural_ = "";
singular_ = "";
return this;
}
@Override
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ResourceProto.internal_static_google_api_ResourceDescriptor_descriptor;
}
@Override
public ResourceDescriptor getDefaultInstanceForType() {
return ResourceDescriptor.getDefaultInstance();
}
@Override
public ResourceDescriptor build() {
ResourceDescriptor result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@Override
public ResourceDescriptor buildPartial() {
ResourceDescriptor result = new ResourceDescriptor(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.type_ = type_;
if (((bitField0_ & 0x00000002) != 0)) {
pattern_ = pattern_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.pattern_ = pattern_;
result.nameField_ = nameField_;
result.history_ = history_;
result.plural_ = plural_;
result.singular_ = singular_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@Override
public Builder clone() {
return super.clone();
}
@Override
public Builder setField(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return super.setField(field, value);
}
@Override
public Builder clearField(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@Override
public Builder clearOneof(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@Override
public Builder setRepeatedField(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return super.setRepeatedField(field, index, value);
}
@Override
public Builder addRepeatedField(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return super.addRepeatedField(field, value);
}
@Override
public Builder mergeFrom(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Message other) {
if (other instanceof ResourceDescriptor) {
return mergeFrom((ResourceDescriptor)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ResourceDescriptor other) {
if (other == ResourceDescriptor.getDefaultInstance()) return this;
if (!other.getType().isEmpty()) {
type_ = other.type_;
onChanged();
}
if (!other.pattern_.isEmpty()) {
if (pattern_.isEmpty()) {
pattern_ = other.pattern_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensurePatternIsMutable();
pattern_.addAll(other.pattern_);
}
onChanged();
}
if (!other.getNameField().isEmpty()) {
nameField_ = other.nameField_;
onChanged();
}
if (other.history_ != 0) {
setHistoryValue(other.getHistoryValue());
}
if (!other.getPlural().isEmpty()) {
plural_ = other.plural_;
onChanged();
}
if (!other.getSingular().isEmpty()) {
singular_ = other.singular_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@Override
public final boolean isInitialized() {
return true;
}
@Override
public Builder mergeFrom(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedInputStream input,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ResourceDescriptor parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ResourceDescriptor) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private Object type_ = "";
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public String getType() {
Object ref = type_;
if (!(ref instanceof String)) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getTypeBytes() {
Object ref = type_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
type_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public Builder setType(
String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
return this;
}
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
*
* The resource type. It must be in the format of
* {service_name}/{resource_type_kind}. The `resource_type_kind` must be
* singular and must not include version numbers.
* Example: `storage.googleapis.com/Bucket`
* The value of the resource_type_kind must follow the regular expression
* /[A-Za-z][a-zA-Z0-9]+/. It should start with an upper case character and
* should use PascalCase (UpperCamelCase). The maximum number of
* characters allowed for the `resource_type_kind` is 100.
*
*
* string type = 1;
*/
public Builder setTypeBytes(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
onChanged();
return this;
}
private lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringList pattern_ = lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringArrayList.EMPTY;
private void ensurePatternIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
pattern_ = new lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringArrayList(pattern_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ProtocolStringList
getPatternList() {
return pattern_.getUnmodifiableView();
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public int getPatternCount() {
return pattern_.size();
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public String getPattern(int index) {
return pattern_.get(index);
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getPatternBytes(int index) {
return pattern_.getByteString(index);
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public Builder setPattern(
int index, String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePatternIsMutable();
pattern_.set(index, value);
onChanged();
return this;
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public Builder addPattern(
String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePatternIsMutable();
pattern_.add(value);
onChanged();
return this;
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public Builder addAllPattern(
Iterable values) {
ensurePatternIsMutable();
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.AbstractMessageLite.Builder.addAll(
values, pattern_);
onChanged();
return this;
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public Builder clearPattern() {
pattern_ = lightstep.com.lightstep.lightstep.lightstep.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Optional. The relative resource name pattern associated with this resource
* type. The DNS prefix of the full resource name shouldn't be specified here.
* The path pattern must follow the syntax, which aligns with HTTP binding
* syntax:
* Template = Segment { "/" Segment } ;
* Segment = LITERAL | Variable ;
* Variable = "{" LITERAL "}" ;
* Examples:
* - "projects/{project}/topics/{topic}"
* - "projects/{project}/knowledgeBases/{knowledge_base}"
* The components in braces correspond to the IDs for each resource in the
* hierarchy. It is expected that, if multiple patterns are provided,
* the same component name (e.g. "project") refers to IDs of the same
* type of resource.
*
*
* repeated string pattern = 2;
*/
public Builder addPatternBytes(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensurePatternIsMutable();
pattern_.add(value);
onChanged();
return this;
}
private Object nameField_ = "";
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public String getNameField() {
Object ref = nameField_;
if (!(ref instanceof String)) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
nameField_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getNameFieldBytes() {
Object ref = nameField_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
nameField_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public Builder setNameField(
String value) {
if (value == null) {
throw new NullPointerException();
}
nameField_ = value;
onChanged();
return this;
}
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public Builder clearNameField() {
nameField_ = getDefaultInstance().getNameField();
onChanged();
return this;
}
/**
*
* Optional. The field on the resource that designates the resource name
* field. If omitted, this is assumed to be "name".
*
*
* string name_field = 3;
*/
public Builder setNameFieldBytes(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nameField_ = value;
onChanged();
return this;
}
private int history_ = 0;
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public int getHistoryValue() {
return history_;
}
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public Builder setHistoryValue(int value) {
history_ = value;
onChanged();
return this;
}
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public History getHistory() {
@SuppressWarnings("deprecation")
History result = History.valueOf(history_);
return result == null ? History.UNRECOGNIZED : result;
}
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public Builder setHistory(History value) {
if (value == null) {
throw new NullPointerException();
}
history_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Optional. The historical or future-looking state of the resource pattern.
* Example:
* // The InspectTemplate message originally only supported resource
* // names with organization, and project was added later.
* message InspectTemplate {
* option (google.api.resource) = {
* type: "dlp.googleapis.com/InspectTemplate"
* pattern:
* "organizations/{organization}/inspectTemplates/{inspect_template}"
* pattern: "projects/{project}/inspectTemplates/{inspect_template}"
* history: ORIGINALLY_SINGLE_PATTERN
* };
* }
*
*
* .google.api.ResourceDescriptor.History history = 4;
*/
public Builder clearHistory() {
history_ = 0;
onChanged();
return this;
}
private Object plural_ = "";
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public String getPlural() {
Object ref = plural_;
if (!(ref instanceof String)) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
plural_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getPluralBytes() {
Object ref = plural_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
plural_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public Builder setPlural(
String value) {
if (value == null) {
throw new NullPointerException();
}
plural_ = value;
onChanged();
return this;
}
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public Builder clearPlural() {
plural_ = getDefaultInstance().getPlural();
onChanged();
return this;
}
/**
*
* The plural name used in the resource name, such as 'projects' for
* the name of 'projects/{project}'. It is the same concept of the `plural`
* field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
*
*
* string plural = 5;
*/
public Builder setPluralBytes(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
plural_ = value;
onChanged();
return this;
}
private Object singular_ = "";
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public String getSingular() {
Object ref = singular_;
if (!(ref instanceof String)) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString bs =
(lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
singular_ = s;
return s;
} else {
return (String) ref;
}
}
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString
getSingularBytes() {
Object ref = singular_;
if (ref instanceof String) {
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString b =
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString.copyFromUtf8(
(String) ref);
singular_ = b;
return b;
} else {
return (lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString) ref;
}
}
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public Builder setSingular(
String value) {
if (value == null) {
throw new NullPointerException();
}
singular_ = value;
onChanged();
return this;
}
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public Builder clearSingular() {
singular_ = getDefaultInstance().getSingular();
onChanged();
return this;
}
/**
*
* The same concept of the `singular` field in k8s CRD spec
* https://kubernetes.io/docs/tasks/access-kubernetes-api/custom-resources/custom-resource-definitions/
* Such as "project" for the `resourcemanager.googleapis.com/Project` type.
*
*
* string singular = 6;
*/
public Builder setSingularBytes(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
singular_ = value;
onChanged();
return this;
}
@Override
public final Builder setUnknownFields(
final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@Override
public final Builder mergeUnknownFields(
final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.api.ResourceDescriptor)
}
// @@protoc_insertion_point(class_scope:google.api.ResourceDescriptor)
private static final ResourceDescriptor DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ResourceDescriptor();
}
public static ResourceDescriptor getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Parser
PARSER = new lightstep.com.lightstep.lightstep.lightstep.google.protobuf.AbstractParser() {
@Override
public ResourceDescriptor parsePartialFrom(
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.CodedInputStream input,
lightstep.com.lightstep.lightstep.lightstep.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws lightstep.com.lightstep.lightstep.lightstep.google.protobuf.InvalidProtocolBufferException {
return new ResourceDescriptor(input, extensionRegistry);
}
};
public static lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Parser parser() {
return PARSER;
}
@Override
public lightstep.com.lightstep.lightstep.lightstep.google.protobuf.Parser getParserForType() {
return PARSER;
}
@Override
public ResourceDescriptor getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy