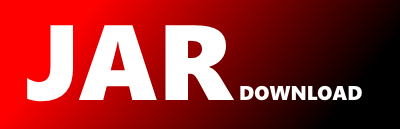
oshi.hardware.platform.mac.MacGlobalMemory Maven / Gradle / Ivy
/**
* MIT License
*
* Copyright (c) 2010 - 2021 The OSHI Project Contributors: https://github.com/oshi/oshi/graphs/contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package oshi.hardware.platform.mac;
import static oshi.util.Memoizer.defaultExpiration;
import static oshi.util.Memoizer.memoize;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Supplier;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.sun.jna.Native; // NOSONAR
import com.sun.jna.platform.mac.SystemB;
import com.sun.jna.platform.mac.SystemB.VMStatistics;
import com.sun.jna.ptr.IntByReference;
import com.sun.jna.ptr.LongByReference;
import oshi.annotation.concurrent.ThreadSafe;
import oshi.hardware.PhysicalMemory;
import oshi.hardware.VirtualMemory;
import oshi.hardware.common.AbstractGlobalMemory;
import oshi.util.Constants;
import oshi.util.ExecutingCommand;
import oshi.util.ParseUtil;
import oshi.util.platform.mac.SysctlUtil;
/**
* Memory obtained by host_statistics (vm_stat) and sysctl.
*/
@ThreadSafe
final class MacGlobalMemory extends AbstractGlobalMemory {
private static final Logger LOG = LoggerFactory.getLogger(MacGlobalMemory.class);
private final Supplier available = memoize(this::queryVmStats, defaultExpiration());
private final Supplier total = memoize(MacGlobalMemory::queryPhysMem);
private final Supplier pageSize = memoize(MacGlobalMemory::queryPageSize);
private final Supplier vm = memoize(this::createVirtualMemory);
@Override
public long getAvailable() {
return available.get();
}
@Override
public long getTotal() {
return total.get();
}
@Override
public long getPageSize() {
return pageSize.get();
}
@Override
public VirtualMemory getVirtualMemory() {
return vm.get();
}
@Override
public List getPhysicalMemory() {
List pmList = new ArrayList<>();
List sp = ExecutingCommand.runNative("system_profiler SPMemoryDataType");
int bank = 0;
String bankLabel = Constants.UNKNOWN;
long capacity = 0L;
long speed = 0L;
String manufacturer = Constants.UNKNOWN;
String memoryType = Constants.UNKNOWN;
for (String line : sp) {
if (line.trim().startsWith("BANK")) {
// Save previous bank
if (bank++ > 0) {
pmList.add(new PhysicalMemory(bankLabel, capacity, speed, manufacturer, memoryType));
}
bankLabel = line.trim();
int colon = bankLabel.lastIndexOf(':');
if (colon > 0) {
bankLabel = bankLabel.substring(0, colon - 1);
}
} else if (bank > 0) {
String[] split = line.trim().split(":");
if (split.length == 2) {
switch (split[0]) {
case "Size":
capacity = ParseUtil.parseDecimalMemorySizeToBinary(split[1].trim());
break;
case "Type":
memoryType = split[1].trim();
break;
case "Speed":
speed = ParseUtil.parseHertz(split[1]);
break;
case "Manufacturer":
manufacturer = split[1].trim();
break;
default:
break;
}
}
}
}
pmList.add(new PhysicalMemory(bankLabel, capacity, speed, manufacturer, memoryType));
return pmList;
}
private long queryVmStats() {
VMStatistics vmStats = new VMStatistics();
if (0 != SystemB.INSTANCE.host_statistics(SystemB.INSTANCE.mach_host_self(), SystemB.HOST_VM_INFO, vmStats,
new IntByReference(vmStats.size() / SystemB.INT_SIZE))) {
LOG.error("Failed to get host VM info. Error code: {}", Native.getLastError());
return 0L;
}
return (vmStats.free_count + vmStats.inactive_count) * getPageSize();
}
private static long queryPhysMem() {
return SysctlUtil.sysctl("hw.memsize", 0L);
}
private static long queryPageSize() {
LongByReference pPageSize = new LongByReference();
if (0 == SystemB.INSTANCE.host_page_size(SystemB.INSTANCE.mach_host_self(), pPageSize)) {
return pPageSize.getValue();
}
LOG.error("Failed to get host page size. Error code: {}", Native.getLastError());
return 4098L;
}
private VirtualMemory createVirtualMemory() {
return new MacVirtualMemory(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy