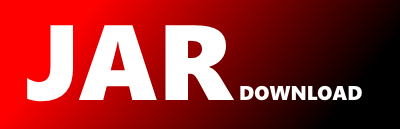
com.linecorp.armeria.spring.AnnotatedServiceRegistrationBean Maven / Gradle / Ivy
/*
* Copyright 2017 LINE Corporation
*
* LINE Corporation licenses this file to you under the Apache License,
* version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package com.linecorp.armeria.spring;
import java.util.ArrayList;
import java.util.Collection;
import javax.validation.constraints.NotNull;
import com.google.common.collect.ImmutableList;
import com.linecorp.armeria.common.HttpHeaders;
import com.linecorp.armeria.server.annotation.ExceptionHandlerFunction;
import com.linecorp.armeria.server.annotation.RequestConverterFunction;
import com.linecorp.armeria.server.annotation.ResponseConverterFunction;
/**
* A bean with information for registering an annotated service object.
* It enables Micrometer metric collection of the service automatically.
* {@code
* > @Bean
* > public AnnotatedServiceRegistrationBean okService() {
* > return new AnnotatedServiceRegistrationBean()
* > .setServiceName("myAnnotatedService")
* > .setPathPrefix("/my_service")
* > .setService(new MyAnnotatedService())
* > .setDecorators(LoggingService.newDecorator())
* > .setExceptionHandlers(new MyExceptionHandler())
* > .setRequestConverters(new MyRequestConverter())
* > .setResponseConverters(new MyResponseConverter())
* > .addExampleRequests(AnnotatedExampleRequest.of("myMethod", "{\"foo\":\"bar\"}"))
* > .addExampleHeaders(ExampleHeaders.of("my-header", "headerVal"));
* > }
* }
*/
public class AnnotatedServiceRegistrationBean
extends AbstractServiceRegistrationBean
© 2015 - 2025 Weber Informatics LLC | Privacy Policy