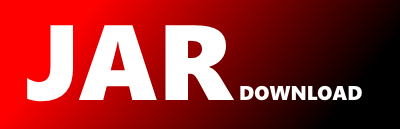
com.linkare.commons.dao.security.impl.UserDAO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpa.security Show documentation
Show all versions of jpa.security Show documentation
Project containing the base domain model for security concerns in JPA projects.
The newest version!
package com.linkare.commons.dao.security.impl;
import static com.linkare.commons.jpa.security.User.FIND_BY_USERNAME_QUERYNAME;
import static com.linkare.commons.jpa.security.User.QUERY_PARAM_USERNAME;
import javax.persistence.EntityManager;
import javax.persistence.NoResultException;
import com.linkare.commons.dao.jpa.GenericDAO;
import com.linkare.commons.dao.security.UserService;
import com.linkare.commons.jpa.security.Login;
import com.linkare.commons.jpa.security.User;
import com.linkare.commons.utils.BooleanResult;
import com.linkare.commons.utils.EqualityUtils;
import com.linkare.commons.utils.PasswordEncryptor;
import com.linkare.commons.utils.StringUtils;
/**
*
* @author Paulo Zenida - Linkare TI
*
*/
public class UserDAO extends GenericDAO implements UserService {
public static final String ERROR_USERNAME_ALREADY_EXISTING = "error.username.already.existing";
public static final String ERROR_USERNAME_CANNOT_BE_EMPTY = "error.username.cannot.be.empty";
private LoginDAO loginDAO;
/**
*
* @param em
* the entity manager to set.
*/
public UserDAO(EntityManager em) {
super(em);
this.loginDAO = new LoginDAO(em);
}
/**
* {@inheritDoc}
*/
@Override
public void create(final User user) {
super.create(user);
if (user.hasLogin()) {
loginDAO.create(user.getLogin());
}
}
/**
* {@inheritDoc}
*
* It checks if the user's username is blank or already existing- In that case, it returns a false result. It returns true, otherwise.
*/
@Override
protected BooleanResult canCreate(final User user) {
if (StringUtils.isBlank(user.getUsername())) {
return new BooleanResult(Boolean.FALSE, ERROR_USERNAME_CANNOT_BE_EMPTY);
}
final User otherUser = findByUsername(user.getUsername());
if (otherUser != null) {
return new BooleanResult(Boolean.FALSE, ERROR_USERNAME_ALREADY_EXISTING);
}
return super.canCreate(user);
}
/**
* {@inheritDoc}
*/
@Override
public User edit(User user) {
if (user.getLogin() != null && !user.getLogin().isPersistent()) {
loginDAO.create(user.getLogin());
} else if (user.getLogin() != null && user.getLogin().isPersistent()) {
loginDAO.edit(user.getLogin());
}
final User updatedUser = super.edit(user);
return updatedUser;
}
/**
* {@inheritDoc} It checks if the user's username already exists. In that case, it returns a false result. It returns true, otherwise.
*/
@Override
protected BooleanResult canEdit(final User user) {
final User otherUser = findByUsername(user.getUsername());
if (otherUser != null && !EqualityUtils.equals(user, otherUser)) {
return new BooleanResult(Boolean.FALSE, ERROR_USERNAME_ALREADY_EXISTING);
}
return super.canEdit(user);
}
/**
* {@inheritDoc}
*/
@Override
public void remove(User user) {
if (user.hasLogin()) {
loginDAO.remove(user.getLogin());
}
super.remove(user);
}
/**
* {@inheritDoc}
*/
@Override
public User findByUsername(final String username) {
try {
return (User) getEntityManager().createNamedQuery(FIND_BY_USERNAME_QUERYNAME).setParameter(QUERY_PARAM_USERNAME, username).getSingleResult();
} catch (NoResultException e) {
return null;
}
}
/**
* {@inheritDoc}
*/
@Override
public User authenticate(User user, String password) {
if (user == null) {
return null;
}
final Login login = user.getLogin();
if (login == null || !PasswordEncryptor.areEquals(login.getPassword(), password)) {
return null;
}
return user;
}
/**
* {@inheritDoc}
*
* @see UserDAO#findByUsername(String)
* @see UserDAO#authenticate(User, String)
*/
@Override
public User authenticate(final String username, final String password) {
User user = findByUsername(username);
return authenticate(user, password);
}
/**
* @return the loginDAO
*/
public LoginDAO getLoginDAO() {
return loginDAO;
}
/**
* @param loginDAO
* the loginDAO to set
*/
public void setLoginDAO(LoginDAO loginDAO) {
this.loginDAO = loginDAO;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy