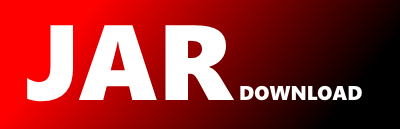
com.linkare.commons.jpa.security.Identification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpa.security Show documentation
Show all versions of jpa.security Show documentation
Project containing the base domain model for security concerns in JPA projects.
The newest version!
package com.linkare.commons.jpa.security;
import javax.persistence.Column;
import javax.persistence.DiscriminatorColumn;
import javax.persistence.Entity;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.NamedQueries;
import javax.persistence.NamedQuery;
import javax.persistence.Table;
import org.hibernate.envers.AuditTable;
import org.hibernate.envers.Audited;
import com.linkare.commons.jpa.DefaultDomainObject;
/**
*
* @author Paulo Zenida - Linkare TI
*
*/
@Entity
@Table(name = "identification")
@DiscriminatorColumn(name = "instance_type")
@NamedQueries({ @NamedQuery(name = Identification.FIND_ALL_QUERYNAME, query = Identification.FIND_ALL_QUERY),
@NamedQuery(name = Identification.COUNT_ALL_QUERYNAME, query = Identification.COUNT_ALL_QUERY) })
@Audited
@AuditTable("identification_audit")
public class Identification extends DefaultDomainObject {
private static final long serialVersionUID = 1L;
public static final String FIND_ALL_QUERYNAME = "Identification.findAll";
public static final String FIND_ALL_QUERY = "Select i from Identification i";
public static final String COUNT_ALL_QUERYNAME = "Identification.countAll";
public static final String COUNT_ALL_QUERY = "Select count(i) from Identification i";
@ManyToOne(optional = true)
@JoinColumn(name = "key_user", nullable = true)
private User user;
@Column(name = "instance_type", insertable = false, updatable = false)
private String instanceType;
/**
* @return the user
*/
public User getUser() {
return user;
}
/**
* @param user
* the user to set
*/
public void setUser(User user) {
if (this.user != null) {
this.user.internalRemoveIdentification(this);
}
this.user = user;
if (user != null) {
user.internalAddIdentification(this);
}
}
/**
* @return the instanceType
*/
public String getInstanceType() {
return instanceType;
}
/**
* @param instanceType
* the instanceType to set
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
public boolean isLogin() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy