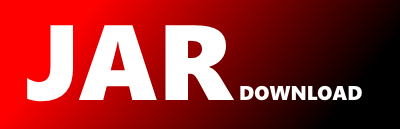
com.linkare.zas.aspectj.utils.ZasUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zas-impl Show documentation
Show all versions of zas-impl Show documentation
The AspectJ based implementation of the Zas project. This is the new version of the implementation of Zas which, initially, started as a M.Sc. thesis project by Paulo Zenida at ISCTE.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy