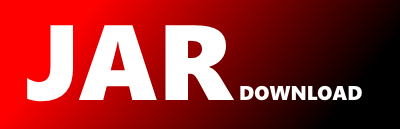
azkaban.executor.ExecutableFlowBase Maven / Gradle / Ivy
/*
* Copyright 2012 LinkedIn, Inc
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package azkaban.executor;
import azkaban.flow.Edge;
import azkaban.flow.Flow;
import azkaban.flow.FlowProps;
import azkaban.flow.Node;
import azkaban.flow.SpecialJobTypes;
import azkaban.project.Project;
import azkaban.utils.TypedMapWrapper;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExecutableFlowBase extends ExecutableNode {
public static final String FLOW_ID_PARAM = "flowId";
public static final String NODES_PARAM = "nodes";
public static final String PROPERTIES_PARAM = "properties";
public static final String SOURCE_PARAM = "source";
public static final String INHERITED_PARAM = "inherited";
private static final Logger logger = LoggerFactory.getLogger(ExecutableFlowBase.class);
private final HashMap executableNodes =
new HashMap<>();
private final HashMap flowProps =
new HashMap<>();
private ArrayList startNodes;
private ArrayList endNodes;
private String flowId;
public ExecutableFlowBase(final Project project, final Node node, final Flow flow,
final ExecutableFlowBase parent) {
super(node, parent);
setFlow(project, flow);
}
public ExecutableFlowBase() {
}
public int getExecutionId() {
if (this.getParentFlow() != null) {
return this.getParentFlow().getExecutionId();
}
return -1;
}
public int getProjectId() {
if (this.getParentFlow() != null) {
return this.getParentFlow().getProjectId();
}
return -1;
}
public String getProjectName() {
if (this.getParentFlow() != null) {
return this.getParentFlow().getProjectName();
}
return null;
}
public int getVersion() {
if (this.getParentFlow() != null) {
return this.getParentFlow().getVersion();
}
return -1;
}
public String getLastModifiedByUser() {
if (this.getParentFlow() != null) {
return this.getParentFlow().getLastModifiedByUser();
}
return null;
}
public long getLastModifiedTimestamp() {
if (this.getParentFlow() != null) {
return this.getParentFlow().getLastModifiedTimestamp();
}
return -1;
}
public Collection getFlowProps() {
return this.flowProps.values();
}
public String getFlowId() {
return this.flowId;
}
protected void setFlow(final Project project, final Flow flow) {
this.flowId = flow.getId();
this.flowProps.putAll(flow.getAllFlowProps());
for (final Node node : flow.getNodes()) {
final String id = node.getId();
if (node.getType().equals(SpecialJobTypes.EMBEDDED_FLOW_TYPE)) {
final String embeddedFlowId = node.getEmbeddedFlowId();
final Flow subFlow = project.getFlow(embeddedFlowId);
final ExecutableFlowBase embeddedFlow =
new ExecutableFlowBase(project, node, subFlow, this);
this.executableNodes.put(id, embeddedFlow);
} else {
final ExecutableNode exNode = new ExecutableNode(node, this);
this.executableNodes.put(id, exNode);
}
}
for (final Edge edge : flow.getEdges()) {
final ExecutableNode sourceNode = this.executableNodes.get(edge.getSourceId());
final ExecutableNode targetNode = this.executableNodes.get(edge.getTargetId());
if (sourceNode == null) {
logger.info("Source node " + edge.getSourceId() + " doesn't exist");
}
sourceNode.addOutNode(edge.getTargetId());
targetNode.addInNode(edge.getSourceId());
}
}
public List getExecutableNodes() {
return new ArrayList<>(this.executableNodes.values());
}
public ExecutableNode getExecutableNode(final String id) {
return this.executableNodes.get(id);
}
public ExecutableNode getExecutableNodePath(final String ids) {
final String[] split = ids.split(":");
return getExecutableNodePath(split);
}
public ExecutableNode getExecutableNodePath(final String... ids) {
return getExecutableNodePath(this, ids, 0);
}
private ExecutableNode getExecutableNodePath(final ExecutableFlowBase flow,
final String[] ids, int currentIdIdx) {
final ExecutableNode node = flow.getExecutableNode(ids[currentIdIdx]);
currentIdIdx++;
if (node == null) {
return null;
}
if (ids.length == currentIdIdx) {
return node;
} else if (node instanceof ExecutableFlowBase) {
return getExecutableNodePath((ExecutableFlowBase) node, ids, currentIdIdx);
} else {
return null;
}
}
public List getStartNodes() {
if (this.startNodes == null) {
this.startNodes = new ArrayList<>();
for (final ExecutableNode node : this.executableNodes.values()) {
if (node.getInNodes().isEmpty()) {
this.startNodes.add(node.getId());
}
}
}
return this.startNodes;
}
public List getEndNodes() {
if (this.endNodes == null) {
this.endNodes = new ArrayList<>();
for (final ExecutableNode node : this.executableNodes.values()) {
if (node.getOutNodes().isEmpty()) {
this.endNodes.add(node.getId());
}
}
}
return this.endNodes;
}
@Override
public Map toObject() {
final Map mapObj = new HashMap<>();
fillMapFromExecutable(mapObj);
return mapObj;
}
@Override
protected void fillMapFromExecutable(final Map flowObjMap) {
super.fillMapFromExecutable(flowObjMap);
flowObjMap.put(FLOW_ID_PARAM, this.flowId);
final ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy