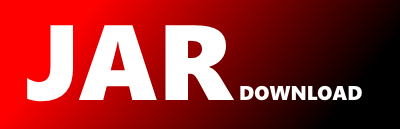
azkaban.executor.ExecutionJobDao Maven / Gradle / Ivy
/*
* Copyright 2017 LinkedIn Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package azkaban.executor;
import azkaban.db.DatabaseOperator;
import azkaban.utils.GZIPUtils;
import azkaban.utils.JSONUtils;
import azkaban.utils.Pair;
import azkaban.utils.Props;
import azkaban.utils.PropsUtils;
import java.io.File;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import javax.inject.Inject;
import javax.inject.Singleton;
import org.apache.commons.dbutils.ResultSetHandler;
import org.apache.commons.io.FileUtils;
import org.apache.log4j.Logger;
@Singleton
public class ExecutionJobDao {
private static final Logger logger = Logger.getLogger(ExecutorDao.class);
private final DatabaseOperator dbOperator;
@Inject
ExecutionJobDao(final DatabaseOperator databaseOperator) {
this.dbOperator = databaseOperator;
}
public void uploadExecutableNode(final ExecutableNode node, final Props inputProps)
throws ExecutorManagerException {
final String INSERT_EXECUTION_NODE = "INSERT INTO execution_jobs "
+ "(exec_id, project_id, version, flow_id, job_id, start_time, "
+ "end_time, status, input_params, attempt) VALUES (?,?,?,?,?,?,?,?,?,?)";
byte[] inputParam = null;
if (inputProps != null) {
try {
final String jsonString =
JSONUtils.toJSON(PropsUtils.toHierarchicalMap(inputProps));
inputParam = GZIPUtils.gzipString(jsonString, "UTF-8");
} catch (final IOException e) {
throw new ExecutorManagerException("Error encoding input params");
}
}
final ExecutableFlow flow = node.getExecutableFlow();
final String flowId = node.getParentFlow().getFlowPath();
logger.info("Uploading flowId " + flowId);
try {
this.dbOperator.update(INSERT_EXECUTION_NODE, flow.getExecutionId(),
flow.getProjectId(), flow.getVersion(), flowId, node.getId(),
node.getStartTime(), node.getEndTime(), node.getStatus().getNumVal(),
inputParam, node.getAttempt());
} catch (final SQLException e) {
throw new ExecutorManagerException("Error writing job " + node.getId(), e);
}
}
public void updateExecutableNode(final ExecutableNode node) throws ExecutorManagerException {
final String UPSERT_EXECUTION_NODE = "UPDATE execution_jobs "
+ "SET start_time=?, end_time=?, status=?, output_params=? "
+ "WHERE exec_id=? AND flow_id=? AND job_id=? AND attempt=?";
byte[] outputParam = null;
final Props outputProps = node.getOutputProps();
if (outputProps != null) {
try {
final String jsonString =
JSONUtils.toJSON(PropsUtils.toHierarchicalMap(outputProps));
outputParam = GZIPUtils.gzipString(jsonString, "UTF-8");
} catch (final IOException e) {
throw new ExecutorManagerException("Error encoding input params");
}
}
try {
this.dbOperator.update(UPSERT_EXECUTION_NODE, node.getStartTime(), node
.getEndTime(), node.getStatus().getNumVal(), outputParam, node
.getExecutableFlow().getExecutionId(), node.getParentFlow()
.getFlowPath(), node.getId(), node.getAttempt());
} catch (final SQLException e) {
throw new ExecutorManagerException("Error updating job " + node.getId(), e);
}
}
public List fetchJobInfoAttempts(final int execId, final String jobId)
throws ExecutorManagerException {
try {
final List info = this.dbOperator.query(
FetchExecutableJobHandler.FETCH_EXECUTABLE_NODE_ATTEMPTS,
new FetchExecutableJobHandler(), execId, jobId);
if (info == null || info.isEmpty()) {
return null;
} else {
return info;
}
} catch (final SQLException e) {
throw new ExecutorManagerException("Error querying job info " + jobId, e);
}
}
public ExecutableJobInfo fetchJobInfo(final int execId, final String jobId, final int attempts)
throws ExecutorManagerException {
try {
final List info =
this.dbOperator.query(FetchExecutableJobHandler.FETCH_EXECUTABLE_NODE,
new FetchExecutableJobHandler(), execId, jobId, attempts);
if (info == null || info.isEmpty()) {
return null;
} else {
return info.get(0);
}
} catch (final SQLException e) {
throw new ExecutorManagerException("Error querying job info " + jobId, e);
}
}
public Props fetchExecutionJobInputProps(final int execId, final String jobId)
throws ExecutorManagerException {
try {
final Pair props = this.dbOperator.query(
FetchExecutableJobPropsHandler.FETCH_INPUT_PARAM_EXECUTABLE_NODE,
new FetchExecutableJobPropsHandler(), execId, jobId);
return props.getFirst();
} catch (final SQLException e) {
throw new ExecutorManagerException("Error querying job params " + execId
+ " " + jobId, e);
}
}
public Props fetchExecutionJobOutputProps(final int execId, final String jobId)
throws ExecutorManagerException {
try {
final Pair props = this.dbOperator.query(
FetchExecutableJobPropsHandler.FETCH_OUTPUT_PARAM_EXECUTABLE_NODE,
new FetchExecutableJobPropsHandler(), execId, jobId);
return props.getFirst();
} catch (final SQLException e) {
throw new ExecutorManagerException("Error querying job params " + execId
+ " " + jobId, e);
}
}
public Pair fetchExecutionJobProps(final int execId, final String jobId)
throws ExecutorManagerException {
try {
return this.dbOperator.query(
FetchExecutableJobPropsHandler.FETCH_INPUT_OUTPUT_PARAM_EXECUTABLE_NODE,
new FetchExecutableJobPropsHandler(), execId, jobId);
} catch (final SQLException e) {
throw new ExecutorManagerException("Error querying job params " + execId
+ " " + jobId, e);
}
}
public List fetchJobHistory(final int projectId,
final String jobId,
final int skip,
final int size) throws ExecutorManagerException {
try {
final List info =
this.dbOperator.query(FetchExecutableJobHandler.FETCH_PROJECT_EXECUTABLE_NODE,
new FetchExecutableJobHandler(), projectId, jobId, skip, size);
if (info == null || info.isEmpty()) {
return null;
} else {
return info;
}
} catch (final SQLException e) {
throw new ExecutorManagerException("Error querying job info " + jobId, e);
}
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy