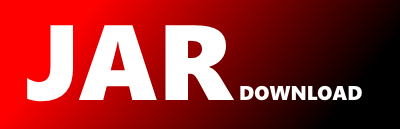
azkaban.executor.ExecutorApiGateway Maven / Gradle / Ivy
/*
* Copyright 2017 LinkedIn Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package azkaban.executor;
import azkaban.utils.JSONUtils;
import azkaban.utils.Pair;
import com.google.inject.Inject;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import javax.inject.Singleton;
import org.codehaus.jackson.map.ObjectMapper;
@Singleton
public class ExecutorApiGateway {
private final ExecutorApiClient apiClient;
@Inject
public ExecutorApiGateway(final ExecutorApiClient apiClient) {
this.apiClient = apiClient;
}
Map callWithExecutable(final ExecutableFlow exflow,
final Executor executor, final String action) throws ExecutorManagerException {
return callWithExecutionId(executor.getHost(), executor.getPort(), action,
exflow.getExecutionId(), null, (Pair[]) null);
}
Map callWithReference(final ExecutionReference ref, final String action,
final Pair... params) throws ExecutorManagerException {
final Executor executor = ref.getExecutor().get();
return callWithExecutionId(executor.getHost(), executor.getPort(), action, ref.getExecId(),
null, params);
}
Map callWithReferenceByUser(final ExecutionReference ref,
final String action, final String user, final Pair... params)
throws ExecutorManagerException {
final Executor executor = ref.getExecutor().get();
return callWithExecutionId(executor.getHost(), executor.getPort(), action,
ref.getExecId(), user, params);
}
Map callWithExecutionId(final String host, final int port,
final String action, final Integer executionId, final String user,
final Pair... params) throws ExecutorManagerException {
try {
final List> paramList = new ArrayList<>();
if (params != null) {
paramList.addAll(Arrays.asList(params));
}
paramList
.add(new Pair<>(ConnectorParams.ACTION_PARAM, action));
paramList.add(new Pair<>(ConnectorParams.EXECID_PARAM, String
.valueOf(executionId)));
paramList.add(new Pair<>(ConnectorParams.USER_PARAM, user));
return callForJsonObjectMap(host, port, "/executor", paramList);
} catch (final IOException e) {
throw new ExecutorManagerException(e);
}
}
/**
* Call executor and parse the JSON response as an instance of the class given as an argument.
*/
T callForJsonType(final String host, final int port, final String path,
final List> paramList, final Class valueType) throws IOException {
final String responseString = callForJsonString(host, port, path, paramList);
if (null == responseString || responseString.length() == 0) {
return null;
}
return new ObjectMapper().readValue(responseString, valueType);
}
/*
* Call executor and return json object map.
*/
Map callForJsonObjectMap(final String host, final int port,
final String path, final List> paramList) throws IOException {
final String responseString =
callForJsonString(host, port, path, paramList);
@SuppressWarnings("unchecked") final Map jsonResponse =
(Map) JSONUtils.parseJSONFromString(responseString);
final String error = (String) jsonResponse.get(ConnectorParams.RESPONSE_ERROR);
if (error != null) {
throw new IOException(error);
}
return jsonResponse;
}
/*
* Call executor and return raw json string.
*/
private String callForJsonString(final String host, final int port, final String path,
List> paramList) throws IOException {
if (paramList == null) {
paramList = new ArrayList<>();
}
@SuppressWarnings("unchecked") final URI uri =
ExecutorApiClient.buildUri(host, port, path, true);
return this.apiClient.httpPost(uri, paramList);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy