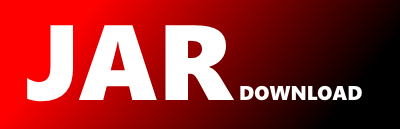
azkaban.executor.ExecutorLoader Maven / Gradle / Ivy
/*
* Copyright 2012 LinkedIn Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package azkaban.executor;
import azkaban.executor.ExecutorLogEvent.EventType;
import azkaban.utils.FileIOUtils.LogData;
import azkaban.utils.Pair;
import azkaban.utils.Props;
import java.io.File;
import java.time.Duration;
import java.util.List;
import java.util.Map;
public interface ExecutorLoader {
void uploadExecutableFlow(ExecutableFlow flow)
throws ExecutorManagerException;
ExecutableFlow fetchExecutableFlow(int execId)
throws ExecutorManagerException;
List fetchRecentlyFinishedFlows(Duration maxAge)
throws ExecutorManagerException;
Map> fetchActiveFlows()
throws ExecutorManagerException;
List fetchFlowHistory(int skip, int num)
throws ExecutorManagerException;
List fetchFlowHistory(int projectId, String flowId,
int skip, int num) throws ExecutorManagerException;
List fetchFlowHistory(int projectId, String flowId,
int skip, int num, Status status) throws ExecutorManagerException;
List fetchFlowHistory(String projContain,
String flowContains, String userNameContains, int status, long startData,
long endData, int skip, int num) throws ExecutorManagerException;
List fetchFlowHistory(final int projectId, final String flowId,
final long startTime) throws ExecutorManagerException;
/**
*
* Fetch all executors from executors table
* Note:-
* 1 throws an Exception in case of a SQL issue
* 2 returns an empty list in case of no executor
*
*
* @return List
*/
List fetchAllExecutors() throws ExecutorManagerException;
/**
*
* Fetch all executors from executors table with active = true
* Note:-
* 1 throws an Exception in case of a SQL issue
* 2 returns an empty list in case of no active executor
*
*
* @return List
*/
List fetchActiveExecutors() throws ExecutorManagerException;
/**
*
* Fetch executor from executors with a given (host, port)
* Note:
* 1. throws an Exception in case of a SQL issue
* 2. return null when no executor is found
* with the given (host,port)
*
*
* @return Executor
*/
Executor fetchExecutor(String host, int port)
throws ExecutorManagerException;
/**
*
* Fetch executor from executors with a given executorId
* Note:
* 1. throws an Exception in case of a SQL issue
* 2. return null when no executor is found with the given executorId
*
*
* @return Executor
*/
Executor fetchExecutor(int executorId) throws ExecutorManagerException;
/**
*
* create an executor and insert in executors table.
* Note:-
* 1. throws an Exception in case of a SQL issue
* 2. throws an Exception if a executor with (host, port) already exist
* 3. return null when no executor is found with the given executorId
*
*
* @return Executor
*/
Executor addExecutor(String host, int port)
throws ExecutorManagerException;
/**
*
* create an executor and insert in executors table.
* Note:-
* 1. throws an Exception in case of a SQL issue
* 2. throws an Exception if there is no executor with the given id
* 3. return null when no executor is found with the given executorId
*
*/
void updateExecutor(Executor executor) throws ExecutorManagerException;
/**
*
* Remove the executor from executors table.
* Note:-
* 1. throws an Exception in case of a SQL issue
* 2. throws an Exception if there is no executor in the table*
*
*/
void removeExecutor(String host, int port) throws ExecutorManagerException;
/**
* * Log an event in executor_event audit table Note:- throws an Exception in * case of a SQL issue * Note: throws an Exception in case of a SQL issue ** * @return isSuccess */ void postExecutorEvent(Executor executor, EventType type, String user, String message) throws ExecutorManagerException; /** *
* This method is to fetch events recorded in executor audit table, inserted * by postExecutorEvents with a given executor, starting from skip * Note:- * 1. throws an Exception in case of a SQL issue * 2. Returns an empty list in case of no events ** * @return List
* Unset executor Id for an execution * Note:- * throws an Exception in case of a SQL issue **/ void unassignExecutor(int executionId) throws ExecutorManagerException; /** *
* Set an executor Id to an execution * Note:- * 1. throws an Exception in case of a SQL issue * 2. throws an Exception in case executionId or executorId do not exist **/ void assignExecutor(int executorId, int execId) throws ExecutorManagerException; /** *
* Fetches an executor corresponding to a given execution * Note:- * 1. throws an Exception in case of a SQL issue * 2. return null when no executor is found with the given executionId ** * @return fetched Executor */ Executor fetchExecutorByExecutionId(int executionId) throws ExecutorManagerException; /** *
* Fetch queued flows which have not yet dispatched * Note: * 1. throws an Exception in case of a SQL issue * 2. return empty list when no queued execution is found ** * @return List of queued flows and corresponding execution reference */ List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy