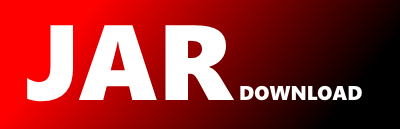
azkaban.flow.Flow Maven / Gradle / Ivy
/*
* Copyright 2012 LinkedIn Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package azkaban.flow;
import azkaban.Constants;
import azkaban.executor.mail.DefaultMailCreator;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class Flow {
private final String id;
private final HashMap nodes = new HashMap<>();
private final HashMap edges = new HashMap<>();
private final HashMap> outEdges =
new HashMap<>();
private final HashMap> inEdges = new HashMap<>();
private final HashMap flowProps =
new HashMap<>();
private int projectId;
private ArrayList startNodes = null;
private ArrayList endNodes = null;
private int numLevels = -1;
private List failureEmail = new ArrayList<>();
private List successEmail = new ArrayList<>();
private String mailCreator = DefaultMailCreator.DEFAULT_MAIL_CREATOR;
private ArrayList errors;
private int version = -1;
private Map metadata = new HashMap<>();
private boolean isLayedOut = false;
private boolean isEmbeddedFlow = false;
private double azkabanFlowVersion = Constants.DEFAULT_AZKABAN_FLOW_VERSION;
private String condition = null;
public Flow(final String id) {
this.id = id;
}
public static Flow flowFromObject(final Object object) {
final Map flowObject = (Map) object;
final String id = (String) flowObject.get("id");
final Boolean layedout = (Boolean) flowObject.get("layedout");
final Boolean isEmbeddedFlow = (Boolean) flowObject.get("embeddedFlow");
final Double azkabanFlowVersion = (Double) flowObject.get("azkabanFlowVersion");
final String condition = (String) flowObject.get("condition");
final Flow flow = new Flow(id);
if (layedout != null) {
flow.setLayedOut(layedout);
}
if (isEmbeddedFlow != null) {
flow.setEmbeddedFlow(isEmbeddedFlow);
}
if (azkabanFlowVersion != null) {
flow.setAzkabanFlowVersion(azkabanFlowVersion);
}
if (condition != null) {
flow.setCondition(condition);
}
final int projId = (Integer) flowObject.get("project.id");
flow.setProjectId(projId);
final int version = (Integer) flowObject.get("version");
flow.setVersion(version);
// Loading projects
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy