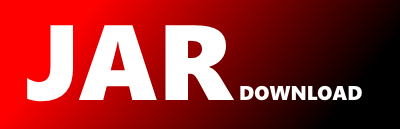
azkaban.trigger.builtin.ExecuteFlowAction Maven / Gradle / Ivy
/*
* Copyright 2012 LinkedIn Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package azkaban.trigger.builtin;
import azkaban.executor.ExecutableFlow;
import azkaban.executor.ExecutionOptions;
import azkaban.executor.ExecutorManagerAdapter;
import azkaban.executor.ExecutorManagerException;
import azkaban.flow.Flow;
import azkaban.flow.FlowUtils;
import azkaban.project.Project;
import azkaban.project.ProjectManager;
import azkaban.sla.SlaOption;
import azkaban.trigger.TriggerAction;
import azkaban.trigger.TriggerManager;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.log4j.Logger;
public class ExecuteFlowAction implements TriggerAction {
public static final String type = "ExecuteFlowAction";
public static final String EXEC_ID = "ExecuteFlowAction.execid";
private static ExecutorManagerAdapter executorManager;
private static TriggerManager triggerManager;
private static ProjectManager projectManager;
private static Logger logger = Logger.getLogger(ExecuteFlowAction.class);
private final String actionId;
private final String projectName;
private int projectId;
private String flowName;
private String submitUser;
private ExecutionOptions executionOptions = new ExecutionOptions();
private List slaOptions;
public ExecuteFlowAction(final String actionId, final int projectId, final String projectName,
final String flowName, final String submitUser, final ExecutionOptions executionOptions,
final List slaOptions) {
this.actionId = actionId;
this.projectId = projectId;
this.projectName = projectName;
this.flowName = flowName;
this.submitUser = submitUser;
this.executionOptions = executionOptions;
this.slaOptions = slaOptions;
}
public static void setLogger(final Logger logger) {
ExecuteFlowAction.logger = logger;
}
public static ExecutorManagerAdapter getExecutorManager() {
return executorManager;
}
public static void setExecutorManager(final ExecutorManagerAdapter executorManager) {
ExecuteFlowAction.executorManager = executorManager;
}
public static TriggerManager getTriggerManager() {
return triggerManager;
}
public static void setTriggerManager(final TriggerManager triggerManager) {
ExecuteFlowAction.triggerManager = triggerManager;
}
public static ProjectManager getProjectManager() {
return projectManager;
}
public static void setProjectManager(final ProjectManager projectManager) {
ExecuteFlowAction.projectManager = projectManager;
}
public static TriggerAction createFromJson(final HashMap obj) {
final Map jsonObj = (HashMap) obj;
final String objType = (String) jsonObj.get("type");
if (!objType.equals(type)) {
throw new RuntimeException("Cannot create action of " + type + " from "
+ objType);
}
final String actionId = (String) jsonObj.get("actionId");
final int projectId = Integer.valueOf((String) jsonObj.get("projectId"));
final String projectName = (String) jsonObj.get("projectName");
final String flowName = (String) jsonObj.get("flowName");
final String submitUser = (String) jsonObj.get("submitUser");
ExecutionOptions executionOptions = null;
if (jsonObj.containsKey("executionOptions")) {
executionOptions =
ExecutionOptions.createFromObject(jsonObj.get("executionOptions"));
}
List slaOptions = null;
if (jsonObj.containsKey("slaOptions")) {
slaOptions = new ArrayList<>();
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy