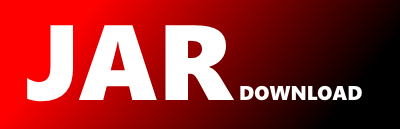
org.apache.calcite.prepare.QueryableRelBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of calcite-core Show documentation
Show all versions of calcite-core Show documentation
Core Calcite APIs and engine.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.calcite.prepare;
import org.apache.calcite.jdbc.CalciteSchema;
import org.apache.calcite.linq4j.Enumerable;
import org.apache.calcite.linq4j.Grouping;
import org.apache.calcite.linq4j.OrderedQueryable;
import org.apache.calcite.linq4j.Queryable;
import org.apache.calcite.linq4j.QueryableDefaults;
import org.apache.calcite.linq4j.QueryableFactory;
import org.apache.calcite.linq4j.function.BigDecimalFunction1;
import org.apache.calcite.linq4j.function.DoubleFunction1;
import org.apache.calcite.linq4j.function.EqualityComparer;
import org.apache.calcite.linq4j.function.FloatFunction1;
import org.apache.calcite.linq4j.function.Function1;
import org.apache.calcite.linq4j.function.Function2;
import org.apache.calcite.linq4j.function.IntegerFunction1;
import org.apache.calcite.linq4j.function.LongFunction1;
import org.apache.calcite.linq4j.function.NullableBigDecimalFunction1;
import org.apache.calcite.linq4j.function.NullableDoubleFunction1;
import org.apache.calcite.linq4j.function.NullableFloatFunction1;
import org.apache.calcite.linq4j.function.NullableIntegerFunction1;
import org.apache.calcite.linq4j.function.NullableLongFunction1;
import org.apache.calcite.linq4j.function.Predicate1;
import org.apache.calcite.linq4j.function.Predicate2;
import org.apache.calcite.linq4j.tree.FunctionExpression;
import org.apache.calcite.rel.RelNode;
import org.apache.calcite.rel.logical.LogicalFilter;
import org.apache.calcite.rel.logical.LogicalProject;
import org.apache.calcite.rel.logical.LogicalTableScan;
import org.apache.calcite.rex.RexNode;
import org.apache.calcite.schema.QueryableTable;
import org.apache.calcite.schema.TranslatableTable;
import org.apache.calcite.schema.impl.AbstractTableQueryable;
import java.math.BigDecimal;
import java.util.Comparator;
import java.util.List;
/**
* Implementation of {@link QueryableFactory}
* that builds a tree of {@link RelNode} planner nodes. Used by
* {@link LixToRelTranslator}.
*
* Each of the methods that implements a {@code Replayer} method creates
* a tree of {@code RelNode}s equivalent to the arguments, and calls
* {@link #setRel} to assign the root of that tree to the {@link #rel} member
* variable.
*
* To comply with the {@link org.apache.calcite.linq4j.QueryableFactory}
* interface, which is after all a factory, each method returns a dummy result
* such as {@code null} or {@code 0}.
* The caller will not use the result.
* The real effect of the method is to
* call {@link #setRel} with a {@code RelNode}.
*
* NOTE: Many methods currently throw {@link UnsupportedOperationException}.
* These method need to be implemented.
*
* @param Element type
*/
class QueryableRelBuilder implements QueryableFactory {
private final LixToRelTranslator translator;
private RelNode rel;
QueryableRelBuilder(LixToRelTranslator translator) {
this.translator = translator;
}
RelNode toRel(Queryable queryable) {
if (queryable instanceof QueryableDefaults.Replayable) {
//noinspection unchecked
((QueryableDefaults.Replayable) queryable).replay(this);
return rel;
}
if (queryable instanceof AbstractTableQueryable) {
final AbstractTableQueryable tableQueryable =
(AbstractTableQueryable) queryable;
final QueryableTable table = tableQueryable.table;
final CalciteSchema.TableEntry tableEntry =
CalciteSchema.from(tableQueryable.schema)
.add(tableQueryable.tableName, tableQueryable.table);
final RelOptTableImpl relOptTable =
RelOptTableImpl.create(null, table.getRowType(translator.typeFactory),
tableEntry, null);
if (table instanceof TranslatableTable) {
return ((TranslatableTable) table).toRel(translator.toRelContext(),
relOptTable);
} else {
return LogicalTableScan.create(translator.cluster, relOptTable);
}
}
return translator.translate(queryable.getExpression());
}
/** Sets the output of this event. */
private void setRel(RelNode rel) {
this.rel = rel;
}
// ~ Methods from QueryableFactory -----------------------------------------
public TResult aggregate(
Queryable source,
TAccumulate seed,
FunctionExpression> func,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public T aggregate(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public TAccumulate aggregate(
Queryable source,
TAccumulate seed,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public boolean all(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public boolean any(Queryable source) {
throw new UnsupportedOperationException();
}
public boolean any(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public BigDecimal averageBigDecimal(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public BigDecimal averageNullableBigDecimal(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public double averageDouble(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Double averageNullableDouble(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public int averageInteger(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Integer averageNullableInteger(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public float averageFloat(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Float averageNullableFloat(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public long averageLong(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Long averageNullableLong(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Queryable concat(
Queryable source, Enumerable source2) {
throw new UnsupportedOperationException();
}
public boolean contains(
Queryable source, T element) {
throw new UnsupportedOperationException();
}
public boolean contains(
Queryable source, T element, EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public int count(Queryable source) {
throw new UnsupportedOperationException();
}
public int count(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public Queryable defaultIfEmpty(Queryable source) {
throw new UnsupportedOperationException();
}
public Queryable defaultIfEmpty(Queryable source, T value) {
throw new UnsupportedOperationException();
}
public Queryable distinct(
Queryable source) {
throw new UnsupportedOperationException();
}
public Queryable distinct(
Queryable source, EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public T elementAt(Queryable source, int index) {
throw new UnsupportedOperationException();
}
public T elementAtOrDefault(Queryable source, int index) {
throw new UnsupportedOperationException();
}
public Queryable except(
Queryable source, Enumerable enumerable) {
throw new UnsupportedOperationException();
}
public Queryable except(
Queryable source,
Enumerable enumerable,
EqualityComparer tEqualityComparer) {
throw new UnsupportedOperationException();
}
public T first(Queryable source) {
throw new UnsupportedOperationException();
}
public T first(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public T firstOrDefault(
Queryable source) {
throw new UnsupportedOperationException();
}
public T firstOrDefault(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public Queryable> groupBy(
Queryable source,
FunctionExpression> keySelector) {
throw new UnsupportedOperationException();
}
public Queryable> groupBy(
Queryable source,
FunctionExpression> keySelector,
EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public Queryable> groupBy(
Queryable source,
FunctionExpression> keySelector,
FunctionExpression> elementSelector) {
throw new UnsupportedOperationException();
}
public Queryable groupByK(
Queryable source,
FunctionExpression> keySelector,
FunctionExpression, TResult>>
resultSelector) {
throw new UnsupportedOperationException();
}
public Queryable> groupBy(
Queryable source,
FunctionExpression> keySelector,
FunctionExpression> elementSelector,
EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public Queryable groupByK(
Queryable source,
FunctionExpression> keySelector,
FunctionExpression, TResult>>
elementSelector,
EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public Queryable groupBy(
Queryable source,
FunctionExpression> keySelector,
FunctionExpression> elementSelector,
FunctionExpression, TResult>>
resultSelector) {
throw new UnsupportedOperationException();
}
public Queryable groupBy(
Queryable source,
FunctionExpression> keySelector,
FunctionExpression> elementSelector,
FunctionExpression, TResult>>
resultSelector,
EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public Queryable groupJoin(
Queryable source,
Enumerable inner,
FunctionExpression> outerKeySelector,
FunctionExpression> innerKeySelector,
FunctionExpression, TResult>>
resultSelector) {
throw new UnsupportedOperationException();
}
public Queryable groupJoin(
Queryable source,
Enumerable inner,
FunctionExpression> outerKeySelector,
FunctionExpression> innerKeySelector,
FunctionExpression, TResult>>
resultSelector,
EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public Queryable intersect(
Queryable source, Enumerable enumerable) {
throw new UnsupportedOperationException();
}
public Queryable intersect(
Queryable source,
Enumerable enumerable,
EqualityComparer tEqualityComparer) {
throw new UnsupportedOperationException();
}
public Queryable join(
Queryable source,
Enumerable inner,
FunctionExpression> outerKeySelector,
FunctionExpression> innerKeySelector,
FunctionExpression> resultSelector) {
throw new UnsupportedOperationException();
}
public Queryable join(
Queryable source,
Enumerable inner,
FunctionExpression> outerKeySelector,
FunctionExpression> innerKeySelector,
FunctionExpression> resultSelector,
EqualityComparer comparer) {
throw new UnsupportedOperationException();
}
public T last(Queryable source) {
throw new UnsupportedOperationException();
}
public T last(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public T lastOrDefault(
Queryable source) {
throw new UnsupportedOperationException();
}
public T lastOrDefault(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public long longCount(Queryable source) {
throw new UnsupportedOperationException();
}
public long longCount(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public T max(Queryable source) {
throw new UnsupportedOperationException();
}
public > TResult max(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public T min(Queryable source) {
throw new UnsupportedOperationException();
}
public > TResult min(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Queryable ofType(
Queryable source, Class clazz) {
throw new UnsupportedOperationException();
}
public Queryable cast(
Queryable source,
Class clazz) {
throw new UnsupportedOperationException();
}
public OrderedQueryable orderBy(
Queryable source,
FunctionExpression> keySelector) {
throw new UnsupportedOperationException();
}
public OrderedQueryable orderBy(
Queryable source,
FunctionExpression> keySelector,
Comparator comparator) {
throw new UnsupportedOperationException();
}
public OrderedQueryable orderByDescending(
Queryable source,
FunctionExpression> keySelector) {
throw new UnsupportedOperationException();
}
public OrderedQueryable orderByDescending(
Queryable source,
FunctionExpression> keySelector,
Comparator comparator) {
throw new UnsupportedOperationException();
}
public Queryable reverse(
Queryable source) {
throw new UnsupportedOperationException();
}
public Queryable select(
Queryable source,
FunctionExpression> selector) {
RelNode child = toRel(source);
List nodes = translator.toRexList(selector, child);
setRel(
LogicalProject.create(child, nodes, (List) null));
return null;
}
public Queryable selectN(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Queryable selectMany(
Queryable source,
FunctionExpression>> selector) {
throw new UnsupportedOperationException();
}
public Queryable selectManyN(
Queryable source,
FunctionExpression>> selector) {
throw new UnsupportedOperationException();
}
public Queryable selectMany(
Queryable source,
FunctionExpression>>
collectionSelector,
FunctionExpression> resultSelector) {
throw new UnsupportedOperationException();
}
public Queryable selectManyN(
Queryable source,
FunctionExpression>>
collectionSelector,
FunctionExpression> resultSelector) {
throw new UnsupportedOperationException();
}
public boolean sequenceEqual(
Queryable source, Enumerable enumerable) {
throw new UnsupportedOperationException();
}
public boolean sequenceEqual(
Queryable source,
Enumerable enumerable,
EqualityComparer tEqualityComparer) {
throw new UnsupportedOperationException();
}
public T single(Queryable source) {
throw new UnsupportedOperationException();
}
public T single(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public T singleOrDefault(Queryable source) {
throw new UnsupportedOperationException();
}
public T singleOrDefault(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public Queryable skip(
Queryable source, int count) {
throw new UnsupportedOperationException();
}
public Queryable skipWhile(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public Queryable skipWhileN(
Queryable source,
FunctionExpression> predicate) {
throw new UnsupportedOperationException();
}
public BigDecimal sumBigDecimal(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public BigDecimal sumNullableBigDecimal(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public double sumDouble(
Queryable source,
FunctionExpression> selector) {
throw new UnsupportedOperationException();
}
public Double sumNullableDouble(
Queryable