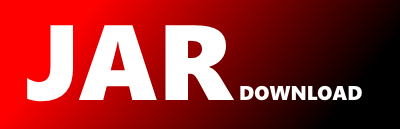
org.apache.calcite.profile.Profiler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of calcite-core Show documentation
Show all versions of calcite-core Show documentation
Core Calcite APIs and engine.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.calcite.profile;
import org.apache.calcite.materialize.Lattice;
import org.apache.calcite.util.ImmutableBitSet;
import org.apache.calcite.util.JsonBuilder;
import org.apache.calcite.util.Util;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSortedSet;
import java.math.BigDecimal;
import java.math.MathContext;
import java.math.RoundingMode;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.NavigableSet;
import java.util.SortedSet;
import javax.annotation.Nonnull;
/**
* Analyzes data sets.
*/
public interface Profiler {
/** Creates a profile of a data set.
*
* @param rows List of rows. Can be iterated over more than once (maybe not
* cheaply)
* @param columns Column definitions
*
* @param initialGroups List of combinations of columns that should be
* profiled early, because they may be interesting
*
* @return A profile describing relationships within the data set
*/
Profile profile(Iterable> rows, List columns,
Collection initialGroups);
/** Column. */
class Column implements Comparable {
public final int ordinal;
public final String name;
/** Creates a Column.
*
* @param ordinal Unique and contiguous within a particular data set
* @param name Name of the column
*/
public Column(int ordinal, String name) {
this.ordinal = ordinal;
this.name = name;
}
static ImmutableBitSet toOrdinals(Iterable columns) {
final ImmutableBitSet.Builder builder = ImmutableBitSet.builder();
for (Column column : columns) {
builder.set(column.ordinal);
}
return builder.build();
}
@Override public int hashCode() {
return ordinal;
}
@Override public boolean equals(Object o) {
return this == o
|| o instanceof Column
&& ordinal == ((Column) o).ordinal;
}
@Override public int compareTo(@Nonnull Column column) {
return Integer.compare(ordinal, column.ordinal);
}
@Override public String toString() {
return name;
}
}
/** Statistic produced by the profiler. */
interface Statistic {
Object toMap(JsonBuilder jsonBuilder);
}
/** Whole data set. */
class RowCount implements Statistic {
final int rowCount;
public RowCount(int rowCount) {
this.rowCount = rowCount;
}
public Object toMap(JsonBuilder jsonBuilder) {
final Map map = jsonBuilder.map();
map.put("type", "rowCount");
map.put("rowCount", rowCount);
return map;
}
}
/** Unique key. */
class Unique implements Statistic {
final NavigableSet columns;
public Unique(SortedSet columns) {
this.columns = ImmutableSortedSet.copyOf(columns);
}
public Object toMap(JsonBuilder jsonBuilder) {
final Map map = jsonBuilder.map();
map.put("type", "unique");
map.put("columns", FunctionalDependency.getObjects(jsonBuilder, columns));
return map;
}
}
/** Functional dependency. */
class FunctionalDependency implements Statistic {
final NavigableSet columns;
final Column dependentColumn;
FunctionalDependency(SortedSet columns, Column dependentColumn) {
this.columns = ImmutableSortedSet.copyOf(columns);
this.dependentColumn = dependentColumn;
}
public Object toMap(JsonBuilder jsonBuilder) {
final Map map = jsonBuilder.map();
map.put("type", "fd");
map.put("columns", getObjects(jsonBuilder, columns));
map.put("dependentColumn", dependentColumn.name);
return map;
}
private static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy