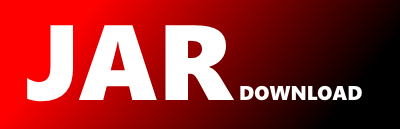
com.linkedin.dagli.dag.AbstractDAGExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
package com.linkedin.dagli.dag;
import com.linkedin.dagli.annotation.Versioned;
import com.linkedin.dagli.objectio.ObjectReader;
import com.linkedin.dagli.preparer.PreparerResult;
import com.linkedin.dagli.util.cloneable.AbstractCloneable;
/**
* DAG executors prepare and apply DAGs. Note that certain DAG executors (e.g. {@link FastPreparedDAGExecutor} may not
* support DAG preparation (training), only application (inference).
*
* @param the type of the derived DAGExecutor
*/
@Versioned
abstract class AbstractDAGExecutor> extends AbstractCloneable
implements PreparedDAGExecutor {
private static final long serialVersionUID = 1L;
@Override
@SuppressWarnings("unchecked") // S is the derived type of this base class
public S internalAPI() {
return (S) this;
}
@Override
public abstract int hashCode(); // force subclasses to override
@Override
public abstract boolean equals(Object obj); // force subclasses to override
protected abstract , T extends PreparableDAGTransformer>
DAGExecutionResult prepareAndApplyUnsafeImpl(T dag, ObjectReader
© 2015 - 2024 Weber Informatics LLC | Privacy Policy