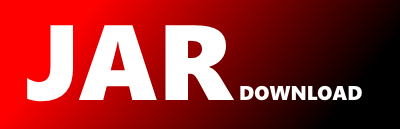
com.linkedin.dagli.dag.DAG Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
// AUTOGENERATED CODE. DO NOT MODIFY DIRECTLY! Instead, please modify the dag/DAG.ftl file.
// See the README in the module's src/template directory for details.
package com.linkedin.dagli.dag;
import com.linkedin.dagli.placeholder.Placeholder;
import com.linkedin.dagli.producer.Producer;
/**
* Static methods for creating preparable DAGs. Use {@link DAG.Prepared} to create prepared DAGs (note that your graph
* must contain no {@link com.linkedin.dagli.transformer.PreparableTransformer}s to be created as a prepared DAG).
*/
public final class DAG {
private DAG() {
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders1 withPlaceholders(Placeholder extends A> placeholder1) {
return new PartialDAG.WithPlaceholders1(placeholder1);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders1 withPlaceholder(Placeholder extends A> placeholder1) {
return new PartialDAG.WithPlaceholders1(placeholder1);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders2 withPlaceholders(Placeholder extends A> placeholder1,
Placeholder extends B> placeholder2) {
return new PartialDAG.WithPlaceholders2(placeholder1, placeholder2);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders3 withPlaceholders(Placeholder extends A> placeholder1,
Placeholder extends B> placeholder2, Placeholder extends C> placeholder3) {
return new PartialDAG.WithPlaceholders3(placeholder1, placeholder2, placeholder3);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders4 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4) {
return new PartialDAG.WithPlaceholders4(placeholder1, placeholder2, placeholder3, placeholder4);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders5 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5) {
return new PartialDAG.WithPlaceholders5(placeholder1, placeholder2, placeholder3, placeholder4,
placeholder5);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders6 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6) {
return new PartialDAG.WithPlaceholders6(placeholder1, placeholder2, placeholder3, placeholder4,
placeholder5, placeholder6);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders7 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7) {
return new PartialDAG.WithPlaceholders7(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5, placeholder6, placeholder7);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @param placeholder8 placeholder 8 of the DAG
* @param the type of placeholder 8
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders8 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7, Placeholder extends H> placeholder8) {
return new PartialDAG.WithPlaceholders8(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5, placeholder6, placeholder7, placeholder8);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @param placeholder8 placeholder 8 of the DAG
* @param the type of placeholder 8
* @param placeholder9 placeholder 9 of the DAG
* @param the type of placeholder 9
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders9 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7, Placeholder extends H> placeholder8,
Placeholder extends I> placeholder9) {
return new PartialDAG.WithPlaceholders9(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5, placeholder6, placeholder7, placeholder8, placeholder9);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @param placeholder8 placeholder 8 of the DAG
* @param the type of placeholder 8
* @param placeholder9 placeholder 9 of the DAG
* @param the type of placeholder 9
* @param placeholder10 placeholder 10 of the DAG
* @param the type of placeholder 10
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.WithPlaceholders10 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7, Placeholder extends H> placeholder8,
Placeholder extends I> placeholder9, Placeholder extends J> placeholder10) {
return new PartialDAG.WithPlaceholders10(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5, placeholder6, placeholder7, placeholder8, placeholder9, placeholder10);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders1 withInputs(Producer extends A> input1) {
return new PartialDAG.WithPlaceholders1(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input1);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders1 withInput(Producer extends A> input1) {
return new PartialDAG.WithPlaceholders1(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input1);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders2 withInputs(Producer extends A> input1,
Producer extends B> input2) {
return new PartialDAG.WithPlaceholders2(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(), input1,
input2);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders3 withInputs(Producer extends A> input1,
Producer extends B> input2, Producer extends C> input3) {
return new PartialDAG.WithPlaceholders3(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input1, input2, input3);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders4 withInputs(Producer extends A> input1,
Producer extends B> input2, Producer extends C> input3, Producer extends D> input4) {
return new PartialDAG.WithPlaceholders4(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input4 instanceof Placeholder
? (Placeholder) input4 : new Placeholder<>(), input1, input2, input3, input4);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders5 withInputs(Producer extends A> input1,
Producer extends B> input2, Producer extends C> input3, Producer extends D> input4,
Producer extends E> input5) {
return new PartialDAG.WithPlaceholders5(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input4 instanceof Placeholder
? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder ? (Placeholder) input5
: new Placeholder<>(), input1, input2, input3, input4, input5);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders6 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6) {
return new PartialDAG.WithPlaceholders6(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input4 instanceof Placeholder
? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder ? (Placeholder) input5
: new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6 : new Placeholder<>(), input1,
input2, input3, input4, input5, input6);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders7 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7) {
return new PartialDAG.WithPlaceholders7(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input4 instanceof Placeholder
? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder ? (Placeholder) input5
: new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6 : new Placeholder<>(),
input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(), input1, input2, input3, input4,
input5, input6, input7);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input8 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders8 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7, Producer extends H> input8) {
return new PartialDAG.WithPlaceholders8(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input8 instanceof Placeholder ? (Placeholder) input8 : new Placeholder<>(), input1, input2, input3, input4,
input5, input6, input7, input8);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input8 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input9 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders9 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7, Producer extends H> input8, Producer extends I> input9) {
return new PartialDAG.WithPlaceholders9(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input8 instanceof Placeholder ? (Placeholder) input8 : new Placeholder<>(), input9 instanceof Placeholder
? (Placeholder) input9 : new Placeholder<>(), input1, input2, input3, input4, input5, input6, input7,
input8, input9);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input8 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input9 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input10 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.WithPlaceholders10 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7, Producer extends H> input8, Producer extends I> input9,
Producer extends J> input10) {
return new PartialDAG.WithPlaceholders10(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input8 instanceof Placeholder ? (Placeholder) input8 : new Placeholder<>(), input9 instanceof Placeholder
? (Placeholder) input9 : new Placeholder<>(), input10 instanceof Placeholder ? (Placeholder) input10
: new Placeholder<>(), input1, input2, input3, input4, input5, input6, input7, input8, input9, input10);
}
/**
* The {@link Prepared} class is analogous to the enclosing {@link DAG} class, but is used to create prepared rather
* than preparable DAGs. Prepared DAGs must contain no {@link com.linkedin.dagli.transformer.PreparableTransformer}s;
* attempting to create a prepared DAG containing a preparable transformer will result in an exception.
*/
public static class Prepared {
private Prepared() {
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders1 withPlaceholders(Placeholder extends A> placeholder1) {
return new PartialDAG.Prepared.WithPlaceholders1(placeholder1);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders1 withPlaceholder(Placeholder extends A> placeholder1) {
return new PartialDAG.Prepared.WithPlaceholders1(placeholder1);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders2 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2) {
return new PartialDAG.Prepared.WithPlaceholders2(placeholder1, placeholder2);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders3 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3) {
return new PartialDAG.Prepared.WithPlaceholders3(placeholder1, placeholder2, placeholder3);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders4 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4) {
return new PartialDAG.Prepared.WithPlaceholders4(placeholder1, placeholder2, placeholder3,
placeholder4);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders5 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5) {
return new PartialDAG.Prepared.WithPlaceholders5(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders6 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6) {
return new PartialDAG.Prepared.WithPlaceholders6(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5, placeholder6);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders7 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7) {
return new PartialDAG.Prepared.WithPlaceholders7(placeholder1, placeholder2, placeholder3,
placeholder4, placeholder5, placeholder6, placeholder7);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @param placeholder8 placeholder 8 of the DAG
* @param the type of placeholder 8
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders8 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7, Placeholder extends H> placeholder8) {
return new PartialDAG.Prepared.WithPlaceholders8(placeholder1, placeholder2,
placeholder3, placeholder4, placeholder5, placeholder6, placeholder7, placeholder8);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @param placeholder8 placeholder 8 of the DAG
* @param the type of placeholder 8
* @param placeholder9 placeholder 9 of the DAG
* @param the type of placeholder 9
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders9 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7, Placeholder extends H> placeholder8,
Placeholder extends I> placeholder9) {
return new PartialDAG.Prepared.WithPlaceholders9(placeholder1, placeholder2,
placeholder3, placeholder4, placeholder5, placeholder6, placeholder7, placeholder8, placeholder9);
}
/**
* Creates a (partial) DAG with the provided {@link Placeholder}s. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* The ancestry of your output nodes must descend (only) from the {@link Placeholder}s provided to this method and from
* Generators. If any output node has another {@link Placeholder} node as an ancestor, an exception will be thrown.
*
* As an alternative to this method, you can instead use withInputs(...) to create a DAG object that captures a
* "subgraph" that is not necessarily rooted on your graph's {@link Placeholder}s.
*
* @param placeholder1 placeholder 1 of the DAG
* @param the type of placeholder 1
* @param placeholder2 placeholder 2 of the DAG
* @param the type of placeholder 2
* @param placeholder3 placeholder 3 of the DAG
* @param the type of placeholder 3
* @param placeholder4 placeholder 4 of the DAG
* @param the type of placeholder 4
* @param placeholder5 placeholder 5 of the DAG
* @param the type of placeholder 5
* @param placeholder6 placeholder 6 of the DAG
* @param the type of placeholder 6
* @param placeholder7 placeholder 7 of the DAG
* @param the type of placeholder 7
* @param placeholder8 placeholder 8 of the DAG
* @param the type of placeholder 8
* @param placeholder9 placeholder 9 of the DAG
* @param the type of placeholder 9
* @param placeholder10 placeholder 10 of the DAG
* @param the type of placeholder 10
* @return a partial DAG rooted at the provided placeholders
*/
public static PartialDAG.Prepared.WithPlaceholders10 withPlaceholders(
Placeholder extends A> placeholder1, Placeholder extends B> placeholder2,
Placeholder extends C> placeholder3, Placeholder extends D> placeholder4,
Placeholder extends E> placeholder5, Placeholder extends F> placeholder6,
Placeholder extends G> placeholder7, Placeholder extends H> placeholder8,
Placeholder extends I> placeholder9, Placeholder extends J> placeholder10) {
return new PartialDAG.Prepared.WithPlaceholders10(placeholder1, placeholder2,
placeholder3, placeholder4, placeholder5, placeholder6, placeholder7, placeholder8, placeholder9,
placeholder10);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders1 withInputs(Producer extends A> input1) {
return new PartialDAG.Prepared.WithPlaceholders1(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input1);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders1 withInput(Producer extends A> input1) {
return new PartialDAG.Prepared.WithPlaceholders1(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input1);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders2 withInputs(Producer extends A> input1,
Producer extends B> input2) {
return new PartialDAG.Prepared.WithPlaceholders2(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(), input1,
input2);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders3 withInputs(Producer extends A> input1,
Producer extends B> input2, Producer extends C> input3) {
return new PartialDAG.Prepared.WithPlaceholders3(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input1, input2, input3);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders4 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4) {
return new PartialDAG.Prepared.WithPlaceholders4(input1 instanceof Placeholder ? (Placeholder) input1
: new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2 : new Placeholder<>(),
input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(), input4 instanceof Placeholder
? (Placeholder) input4 : new Placeholder<>(), input1, input2, input3, input4);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders5 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5) {
return new PartialDAG.Prepared.WithPlaceholders5(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input1, input2, input3, input4, input5);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders6 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6) {
return new PartialDAG.Prepared.WithPlaceholders6(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input1, input2, input3, input4, input5, input6);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders7 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7) {
return new PartialDAG.Prepared.WithPlaceholders7(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input1, input2, input3, input4, input5, input6, input7);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input8 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders8 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7, Producer extends H> input8) {
return new PartialDAG.Prepared.WithPlaceholders8(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input8 instanceof Placeholder ? (Placeholder) input8 : new Placeholder<>(), input1, input2, input3, input4,
input5, input6, input7, input8);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input8 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input9 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders9 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7, Producer extends H> input8, Producer extends I> input9) {
return new PartialDAG.Prepared.WithPlaceholders9(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input8 instanceof Placeholder ? (Placeholder) input8 : new Placeholder<>(), input9 instanceof Placeholder
? (Placeholder) input9 : new Placeholder<>(), input1, input2, input3, input4, input5, input6, input7,
input8, input9);
}
/**
* Creates a (partial) DAG that will have the specified inputs. The partial DAG will become a completed DAG once the
* withOutputs(...) method is then called on it to specify the outputs.
*
* Note that this method will accept all types of {@link Producer}s, not just {@link Placeholder} objects, allowing a DAG
* to be created from a "subgraph" that is not necessarily rooted at its original {@link Placeholder}s. It does this by
* effectively replacing any non-{@link Placeholder} arguments with new {@link Placeholder} objects in the
* created DAG.
*
* For example, let's say we define the following graph:
* {@code
* PlaceholderA -> Transformer1
* PlaceholderB -> Transformer1
* GeneratorC -> Transformer1
* Transformer1 -> Transformer2
* PlaceholderB -> Transformer2
* GeneratorC -> Transformer2
* }
*
* This is a graph where Transformer1 receives inputs from PlaceholderA, PlaceholderB, and GeneratorC, and the
* downstream Transformer2 receives the output of Transformer1 and the inputs from PlaceholderB and GeneratorC.
*
* If we create a DAG with DAG.withInputs(PlaceholderA, PlaceholderB).withOutput(Transformer2), this will be a DAG with
* two inputs and one output, where the DAG's inputs correspond to PlaceholderA and PlaceholderB as normal.
*
* However, let's say that we want to instead create a subgraph, where we provide the values that would
* otherwise be generated by Transformer1 and GeneratorC ourselves. We can just call:
* DAG.withInputs(PlaceholderB, Transformer1, GeneratorC).withOutput(Transformer2)
* In this DAG, Transformer1 and GeneratorC will never run and are instead replaced by new {@link Placeholder}s, such that
* the values provided to their child nodes are those provided to the DAG as input values.
*
* The ancestry of your output nodes must descend (only) from the nodes provided to withInputs(...) and from
* Generators. If any output node has a path to an ancestor {@link Placeholder} node that excludes the nodes passed to
* withInputs(...), an exception will be thrown.
*
* withInputs(...) is intended to help you create DAGs from sub-graphs of a larger DAG. If you just want to create an
* "normal" DAG instance from an entire graph, use withPlaceholders(...) instead. Using withInputs(...) works just as
* well, but withPlaceholders(...) makes your intent to capture the entire graph obvious and is thus easier to read.
* @param input1 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input2 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input3 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input4 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input5 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input6 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input7 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input8 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input9 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @param input10 a producer that will root the subgraph, and whose values will be provided as inputs to the DAG.
* @return a partial DAG configured to use the provided producers as roots
*/
public static PartialDAG.Prepared.WithPlaceholders10 withInputs(
Producer extends A> input1, Producer extends B> input2, Producer extends C> input3,
Producer extends D> input4, Producer extends E> input5, Producer extends F> input6,
Producer extends G> input7, Producer extends H> input8, Producer extends I> input9,
Producer extends J> input10) {
return new PartialDAG.Prepared.WithPlaceholders10(input1 instanceof Placeholder
? (Placeholder) input1 : new Placeholder<>(), input2 instanceof Placeholder ? (Placeholder) input2
: new Placeholder<>(), input3 instanceof Placeholder ? (Placeholder) input3 : new Placeholder<>(),
input4 instanceof Placeholder ? (Placeholder) input4 : new Placeholder<>(), input5 instanceof Placeholder
? (Placeholder) input5 : new Placeholder<>(), input6 instanceof Placeholder ? (Placeholder) input6
: new Placeholder<>(), input7 instanceof Placeholder ? (Placeholder) input7 : new Placeholder<>(),
input8 instanceof Placeholder ? (Placeholder) input8 : new Placeholder<>(), input9 instanceof Placeholder
? (Placeholder) input9 : new Placeholder<>(), input10 instanceof Placeholder ? (Placeholder) input10
: new Placeholder<>(), input1, input2, input3, input4, input5, input6, input7, input8, input9, input10);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy