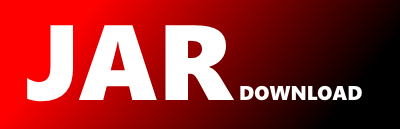
com.linkedin.dagli.preparer.Preparer7 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
// AUTOGENERATED CODE. DO NOT MODIFY DIRECTLY! Instead, please modify the preparer/PreparerX.ftl file.
// See the README in the module's src/template directory for details.
package com.linkedin.dagli.preparer;
import com.linkedin.dagli.objectio.ObjectReader;
import com.linkedin.dagli.transformer.PreparedTransformer7;
import com.linkedin.dagli.util.invariant.Arguments;
import com.linkedin.dagli.tuple.Tuple7;
public interface Preparer7> extends
Preparer {
@Override
default void processUnsafe(Object[] values) {
Arguments.check(values.length == 7, "7 arguments must be provided");
process((A) values[0], (B) values[1], (C) values[2], (D) values[3], (E) values[4], (F) values[5], (G) values[6]);
}
/**
* Processes a single example of preparation data. To prepare a {@link Preparer}, process(...) will be called on
* each and every preparation example before finish(...) is called to complete preparation.
*
* This method is not assumed to be thread-safe and will not be invoked concurrently on the same {@link Preparer}.
*
* @param value1 the first value of the preparation example to be processed
* @param value2 the second value of the preparation example to be processed
* @param value3 the third value of the preparation example to be processed
* @param value4 the fourth value of the preparation example to be processed
* @param value5 the fifth value of the preparation example to be processed
* @param value6 the sixth value of the preparation example to be processed
* @param value7 the seventh value of the preparation example to be processed
*/
void process(A value1, B value2, C value3, D value4, E value5, F value6, G value7);
@Override
default PreparerResultMixed extends PreparedTransformer7 super A, ? super B, ? super C, ? super D, ? super E, ? super F, ? super G, ? extends R>, N> finishUnsafe(
ObjectReader
© 2015 - 2024 Weber Informatics LLC | Privacy Policy