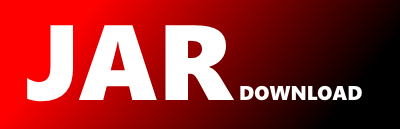
com.linkedin.dagli.producer.AbstractChildProducer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
package com.linkedin.dagli.producer;
import com.linkedin.dagli.producer.internal.ChildProducerInternalAPI;
import java.util.List;
/**
* The base class for child (non-root) producers.
*
* @param the type of result produced by the associated producer
* @param the type of the internal API provided by this producer
* @param the ultimate derived type of this producer
*/
public abstract class AbstractChildProducer, S extends AbstractChildProducer>
extends AbstractProducer
implements ChildProducer {
private static final long serialVersionUID = 1;
protected abstract class InternalAPI extends AbstractProducer.InternalAPI
implements ChildProducerInternalAPI { }
@Override
public void validate() {
super.validate();
List extends Producer>> parents = this.internalAPI().getInputList();
if (parents.isEmpty()) {
throw new IllegalStateException(this.getName() + " is a child producer with no parents");
}
for (int i = 0; i < parents.size(); i++) {
Producer> parent = parents.get(i);
if (parent == null) {
throw new IllegalStateException(this.getName() + " has null parent producer as input #" + i
+ ". This probably means you forgot to set an input on this transformer, e.g. using withInput(...).");
} else if (parent == MissingInput.get()) {
throw new IllegalStateException(this.getName() + " has a MissingInput parent producer as input #" + i
+ ". This probably means you forgot to set an input on this transformer, e.g. using withInput(...).");
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy