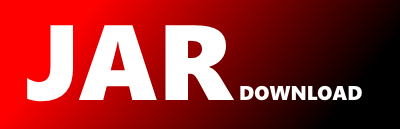
com.linkedin.dagli.tester.AbstractTransformerTestBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
package com.linkedin.dagli.tester;
import com.linkedin.dagli.placeholder.Placeholder;
import com.linkedin.dagli.producer.Producer;
import com.linkedin.dagli.producer.internal.ChildProducerInternalAPI;
import com.linkedin.dagli.transformer.Transformer;
import com.linkedin.dagli.util.array.ArraysEx;
import com.linkedin.dagli.util.closeable.Closeables;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
/**
* Tests a {@link Transformer}.
*
* @param the type of result produced by the {@link Transformer}
*/
abstract class AbstractTransformerTestBuilder, S extends AbstractTransformerTestBuilder>
extends AbstractChildTestBuilder
© 2015 - 2024 Weber Informatics LLC | Privacy Policy