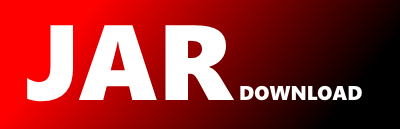
com.linkedin.dagli.util.function.BooleanNegatedFunction9 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of util-function Show documentation
Show all versions of util-function Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
// AUTOGENERATED CODE. DO NOT MODIFY DIRECTLY! Instead, please modify the util/function/BooleanNegatedFunction.ftl file.
// See the README in the module's src/template directory for details.
package com.linkedin.dagli.util.function;
import java.util.Objects;
/**
* Implements the negation of the result of a boolean function.
*
* This class is {@link java.io.Serializable} to allow its subclass (also named Serializable) to be serialized, but
* should not be regarded as "safely serializable" since the function it wraps is not itself guaranteed to be
* serializable.
*/
class BooleanNegatedFunction9 implements BooleanFunction9,
java.io.Serializable {
private static final int CLASS_HASH = BooleanNegatedFunction9.class.hashCode();
private final BooleanFunction9 _function;
// no-arg constructor for Kryo
private BooleanNegatedFunction9() {
_function = null;
}
/**
* Creates a new instance that will negate the result provided by the given, wrapped function.
*
* @param function the function to be wrapped
*/
private BooleanNegatedFunction9(BooleanFunction9 function) {
_function = Objects.requireNonNull(function);
}
/**
* Returns a function that will have a result that is a negation of the function provided. If the passed function
* is itself of this type, its underlying (wrapped) function will be returned; otherwise, a new instance of this class
* will be created to wrap the passed function.
*
* @param function the function whose result will be negated
* @return a function (which may or may not be new) that negates the return value of the provided function
*/
static BooleanFunction9 negate(
BooleanFunction9 function) {
if (function instanceof BooleanNegatedFunction9) {
return ((BooleanNegatedFunction9) function)._function; // negation of a negation is the original function
}
return new BooleanNegatedFunction9<>(function);
}
@Override
public boolean apply(A value1, B value2, C value3, D value4, E value5, F value6, G value7, H value8, I value9) {
return !_function.apply(value1, value2, value3, value4, value5, value6, value7, value8, value9);
}
@Override
public int hashCode() {
return CLASS_HASH + _function.hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj instanceof BooleanNegatedFunction9) {
return this._function.equals(((BooleanNegatedFunction9) obj)._function);
}
return false;
}
static class Serializable extends BooleanNegatedFunction9
implements BooleanFunction9.Serializable {
private static final long serialVersionUID = 1;
// no-arg constructor for Kryo
private Serializable() {
}
/**
* Creates a new instance that will negate the result provided by the given, wrapped function.
*
* @param function the function to be wrapped
*/
private Serializable(BooleanFunction9.Serializable function) {
super(function);
}
/**
* Returns a function that will have a result that is a negation of the function provided. If the passed function
* is itself of this type, its underlying (wrapped) function will be returned; otherwise, a new instance of this class
* will be created to wrap the passed function.
*
* @param function the function whose result will be negated
* @return a function (which may or may not be new) that negates the return value of the provided function
*/
static BooleanFunction9.Serializable negate(
BooleanFunction9.Serializable function) {
if (function instanceof BooleanNegatedFunction9.Serializable) {
// negation of a negation is the original function
return (BooleanFunction9.Serializable) ((BooleanNegatedFunction9) function)._function;
}
return new BooleanNegatedFunction9.Serializable<>(function);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy