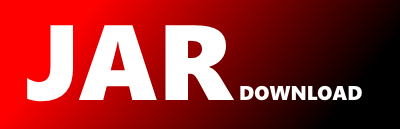
com.linkedin.dagli.util.function.ByteComposedFunction7 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of util-function Show documentation
Show all versions of util-function Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
// AUTOGENERATED CODE. DO NOT MODIFY DIRECTLY! Instead, please modify the util/function/ComposedFunction.ftl file.
// See the README in the module's src/template directory for details.
package com.linkedin.dagli.util.function;
import java.util.Objects;
/**
* A function class implementing {@link ByteFunction7.Serializable} that composes
* {@link ByteFunction7} with a {@link Function1}. The function is only actually serializable
* if its constituent composed functions are serializable, of course.
*/
class ByteComposedFunction7 implements ByteFunction7.Serializable {
private static final long serialVersionUID = 1;
private final Function7 _first;
private final ByteFunction1 super Q> _andThen;
/**
* Creates a new instance that composes two functions, i.e. {@code andThen(first(inputs))}.
*
* @param first the first function to be called in the composition
* @param andThen the second function to be called in the composition, accepting the {@code first} functions result
* as input
*/
ByteComposedFunction7(Function7 first, ByteFunction1 super Q> andThen) {
_first = first;
_andThen = andThen;
}
@Override
@SuppressWarnings("unchecked")
public ByteComposedFunction7 safelySerializable() {
return new ByteComposedFunction7<>(((Function7.Serializable) _first).safelySerializable(),
((ByteFunction1.Serializable super Q>) _andThen).safelySerializable());
}
@Override
public byte apply(A value1, B value2, C value3, D value4, E value5, F value6, G value7) {
return _andThen.apply(_first.apply(value1, value2, value3, value4, value5, value6, value7));
}
@Override
public int hashCode() {
return Objects.hash(ByteComposedFunction7.class, _first, _andThen);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof ByteComposedFunction7) {
return this._first.equals(((ByteComposedFunction7) obj)._first)
&& this._andThen.equals(((ByteComposedFunction7) obj)._andThen);
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy