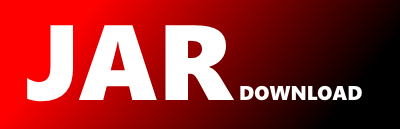
com.linkedin.dagli.util.function.CharacterMethodReference0 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of util-function Show documentation
Show all versions of util-function Show documentation
DAG-oriented machine learning framework for bug-resistant, readable, efficient, maintainable and trivially deployable models in Java and other JVM languages
// AUTOGENERATED CODE. DO NOT MODIFY DIRECTLY! Instead, please modify the util/function/MethodReference.ftl file.
// See the README in the module's src/template directory for details.
package com.linkedin.dagli.util.function;
import java.io.IOException;
import java.lang.invoke.LambdaMetafactory;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodType;
/**
* Represents a safely serializable method reference (assuming the JVM supports {@link MethodReference}, which is not
* guaranteed). If the JVM does not support {@link MethodReference}, an exception may be thrown when creating the
* method reference; fortunately, however, deserialization will not be affected: if you can serialize it, you'll be able
* to deserialize later on any JVM.
*/
class CharacterMethodReference0 implements CharacterFunction0.Serializable {
private static final long serialVersionUID = 1;
// hash and equality distinguish between different types of MethodReferenceX classes even when the underlying method
// references are identical
private static final int CLASS_HASH = CharacterMethodReference0.class.hashCode();
private final MethodReference _methodReference;
private transient CharacterFunction0 _cachedFunction = null;
CharacterMethodReference0(MethodReference mr) {
_methodReference = mr;
initCachedFunction();
}
/**
* Creates a new instance.
*
* The passed func parameter must be a method reference, such as Object::toString or String::length. A runtime
* exception will be thrown if func is a function object or an anonymous lambda (e.g. "a -> a + 5"). An exception
* may also be thrown if your JVM implementation does not support the library's internal method for retrieving
* information about the passed method reference.
*
* @param func a method reference lambda to wrap. If this function is not safely serializable, a runtime exception
* will be thrown.
*/
public CharacterMethodReference0(Serializable func) {
if (func instanceof CharacterMethodReference0) {
// multiple applications of this constructor are idempotent:
_methodReference = ((CharacterMethodReference0) func)._methodReference;
} else {
// this line will thrown an exception if func is not a method reference:
_methodReference = new MethodReference(func);
}
initCachedFunction();
}
// deserialization hook to ensure _cachedFunction is always set
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
initCachedFunction();
}
private void initCachedFunction() {
if (_cachedFunction == null) {
_cachedFunction = fromMethodReference();
}
}
private CharacterFunction0 fromMethodReference() {
if (_methodReference.isBound()) {
CharacterMethodReference1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy