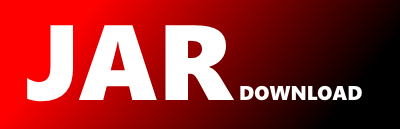
datafu.hourglass.mapreduce.CollapsingReducer Maven / Gradle / Ivy
/**
* Copyright 2013 LinkedIn, Inc
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package datafu.hourglass.mapreduce;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import org.apache.avro.generic.GenericData;
import org.apache.avro.generic.GenericRecord;
import org.apache.avro.generic.GenericData.Record;
import org.apache.avro.mapred.AvroKey;
import org.apache.avro.mapred.AvroValue;
import org.apache.hadoop.mapreduce.ReduceContext;
import datafu.hourglass.fs.DateRange;
import datafu.hourglass.jobs.DateRangeConfigurable;
import datafu.hourglass.model.Accumulator;
import datafu.hourglass.model.Merger;
import datafu.hourglass.schemas.PartitionCollapsingSchemas;
/**
* The reducer used by {@link datafu.hourglass.jobs.AbstractPartitionCollapsingIncrementalJob} and its derived classes.
*
*
* An implementation of {@link datafu.hourglass.model.Accumulator} is used to perform aggregation and produce the
* output value.
*
*
* @author "Matthew Hayes"
*
*/
public class CollapsingReducer extends ObjectReducer implements DateRangeConfigurable, Serializable
{
protected long _beginTime;
protected long _endTime;
private Accumulator _newAccumulator;
private Accumulator _oldAccumulator;
private Merger _merger;
private Merger _oldMerger;
private boolean _reusePreviousOutput;
private PartitionCollapsingSchemas _schemas;
@SuppressWarnings("unchecked")
public void reduce(Object keyObj,
Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy