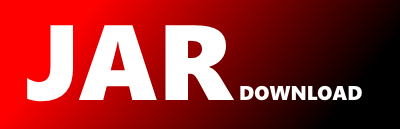
com.linkedin.restli.client.config.ConfigValueCoercers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parseq-restli-client Show documentation
Show all versions of parseq-restli-client Show documentation
Uploads all artifacts belonging to configuration ':parseq-restli-client:archives'
package com.linkedin.restli.client.config;
import java.util.HashSet;
import java.util.Set;
import com.linkedin.parseq.function.Function1;
class ConfigValueCoercers {
public static final Function1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy