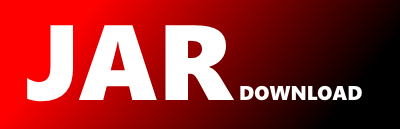
com.linkedin.restli.common.ErrorResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restli-common Show documentation
Show all versions of restli-common Show documentation
Pegasus is a framework for building robust, scalable service architectures using dynamic discovery and simple asychronous type-checked REST + JSON APIs.
package com.linkedin.restli.common;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.linkedin.data.DataMap;
import com.linkedin.data.schema.PathSpec;
import com.linkedin.data.schema.RecordDataSchema;
import com.linkedin.data.template.DataTemplateUtil;
import com.linkedin.data.template.GetMode;
import com.linkedin.data.template.RecordTemplate;
import com.linkedin.data.template.SetMode;
/**
* A generic ErrorResponse
*
*/
@Generated(value = "com.linkedin.pegasus.generator.JavaCodeUtil", comments = "Rest.li Data Template. Generated from /Users/mnchen/dev/pegasus_trunk/pegasus/restli-common/src/main/pegasus/com/linkedin/restli/common/ErrorResponse.pdsc.", date = "Tue Oct 03 15:15:24 PDT 2017")
public class ErrorResponse
extends RecordTemplate
{
private final static ErrorResponse.Fields _fields = new ErrorResponse.Fields();
private final static RecordDataSchema SCHEMA = ((RecordDataSchema) DataTemplateUtil.parseSchema("{\"type\":\"record\",\"name\":\"ErrorResponse\",\"namespace\":\"com.linkedin.restli.common\",\"doc\":\"A generic ErrorResponse\",\"fields\":[{\"name\":\"status\",\"type\":\"int\",\"doc\":\"The HTTP status code\",\"optional\":true},{\"name\":\"serviceErrorCode\",\"type\":\"int\",\"doc\":\"An service-specific error code (documented in prose)\",\"optional\":true},{\"name\":\"message\",\"type\":\"string\",\"doc\":\"A human-readable explanation of the error\",\"optional\":true},{\"name\":\"exceptionClass\",\"type\":\"string\",\"doc\":\"The FQCN of the exception thrown by the server (included the case of a server fault)\",\"optional\":true},{\"name\":\"stackTrace\",\"type\":\"string\",\"doc\":\"The full (??) stack trace (included the case of a server fault)\",\"optional\":true},{\"name\":\"errorDetails\",\"type\":{\"type\":\"record\",\"name\":\"ErrorDetails\",\"fields\":[]},\"optional\":true}]}"));
private final static RecordDataSchema.Field FIELD_Status = SCHEMA.getField("status");
private final static RecordDataSchema.Field FIELD_ServiceErrorCode = SCHEMA.getField("serviceErrorCode");
private final static RecordDataSchema.Field FIELD_Message = SCHEMA.getField("message");
private final static RecordDataSchema.Field FIELD_ExceptionClass = SCHEMA.getField("exceptionClass");
private final static RecordDataSchema.Field FIELD_StackTrace = SCHEMA.getField("stackTrace");
private final static RecordDataSchema.Field FIELD_ErrorDetails = SCHEMA.getField("errorDetails");
public ErrorResponse() {
super(new DataMap(), SCHEMA);
}
public ErrorResponse(DataMap data) {
super(data, SCHEMA);
}
public static ErrorResponse.Fields fields() {
return _fields;
}
/**
* Existence checker for status
*
* @see ErrorResponse.Fields#status
*/
public boolean hasStatus() {
return contains(FIELD_Status);
}
/**
* Remover for status
*
* @see ErrorResponse.Fields#status
*/
public void removeStatus() {
remove(FIELD_Status);
}
/**
* Getter for status
*
* @see ErrorResponse.Fields#status
*/
public Integer getStatus(GetMode mode) {
return obtainDirect(FIELD_Status, Integer.class, mode);
}
/**
* Getter for status
*
* @return
* Optional field. Always check for null.
* @see ErrorResponse.Fields#status
*/
@Nullable
public Integer getStatus() {
return obtainDirect(FIELD_Status, Integer.class, GetMode.STRICT);
}
/**
* Setter for status
*
* @see ErrorResponse.Fields#status
*/
public ErrorResponse setStatus(Integer value, SetMode mode) {
putDirect(FIELD_Status, Integer.class, Integer.class, value, mode);
return this;
}
/**
* Setter for status
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see ErrorResponse.Fields#status
*/
public ErrorResponse setStatus(
@Nonnull
Integer value) {
putDirect(FIELD_Status, Integer.class, Integer.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Setter for status
*
* @see ErrorResponse.Fields#status
*/
public ErrorResponse setStatus(int value) {
putDirect(FIELD_Status, Integer.class, Integer.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for serviceErrorCode
*
* @see ErrorResponse.Fields#serviceErrorCode
*/
public boolean hasServiceErrorCode() {
return contains(FIELD_ServiceErrorCode);
}
/**
* Remover for serviceErrorCode
*
* @see ErrorResponse.Fields#serviceErrorCode
*/
public void removeServiceErrorCode() {
remove(FIELD_ServiceErrorCode);
}
/**
* Getter for serviceErrorCode
*
* @see ErrorResponse.Fields#serviceErrorCode
*/
public Integer getServiceErrorCode(GetMode mode) {
return obtainDirect(FIELD_ServiceErrorCode, Integer.class, mode);
}
/**
* Getter for serviceErrorCode
*
* @return
* Optional field. Always check for null.
* @see ErrorResponse.Fields#serviceErrorCode
*/
@Nullable
public Integer getServiceErrorCode() {
return obtainDirect(FIELD_ServiceErrorCode, Integer.class, GetMode.STRICT);
}
/**
* Setter for serviceErrorCode
*
* @see ErrorResponse.Fields#serviceErrorCode
*/
public ErrorResponse setServiceErrorCode(Integer value, SetMode mode) {
putDirect(FIELD_ServiceErrorCode, Integer.class, Integer.class, value, mode);
return this;
}
/**
* Setter for serviceErrorCode
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see ErrorResponse.Fields#serviceErrorCode
*/
public ErrorResponse setServiceErrorCode(
@Nonnull
Integer value) {
putDirect(FIELD_ServiceErrorCode, Integer.class, Integer.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Setter for serviceErrorCode
*
* @see ErrorResponse.Fields#serviceErrorCode
*/
public ErrorResponse setServiceErrorCode(int value) {
putDirect(FIELD_ServiceErrorCode, Integer.class, Integer.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for message
*
* @see ErrorResponse.Fields#message
*/
public boolean hasMessage() {
return contains(FIELD_Message);
}
/**
* Remover for message
*
* @see ErrorResponse.Fields#message
*/
public void removeMessage() {
remove(FIELD_Message);
}
/**
* Getter for message
*
* @see ErrorResponse.Fields#message
*/
public String getMessage(GetMode mode) {
return obtainDirect(FIELD_Message, String.class, mode);
}
/**
* Getter for message
*
* @return
* Optional field. Always check for null.
* @see ErrorResponse.Fields#message
*/
@Nullable
public String getMessage() {
return obtainDirect(FIELD_Message, String.class, GetMode.STRICT);
}
/**
* Setter for message
*
* @see ErrorResponse.Fields#message
*/
public ErrorResponse setMessage(String value, SetMode mode) {
putDirect(FIELD_Message, String.class, String.class, value, mode);
return this;
}
/**
* Setter for message
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see ErrorResponse.Fields#message
*/
public ErrorResponse setMessage(
@Nonnull
String value) {
putDirect(FIELD_Message, String.class, String.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for exceptionClass
*
* @see ErrorResponse.Fields#exceptionClass
*/
public boolean hasExceptionClass() {
return contains(FIELD_ExceptionClass);
}
/**
* Remover for exceptionClass
*
* @see ErrorResponse.Fields#exceptionClass
*/
public void removeExceptionClass() {
remove(FIELD_ExceptionClass);
}
/**
* Getter for exceptionClass
*
* @see ErrorResponse.Fields#exceptionClass
*/
public String getExceptionClass(GetMode mode) {
return obtainDirect(FIELD_ExceptionClass, String.class, mode);
}
/**
* Getter for exceptionClass
*
* @return
* Optional field. Always check for null.
* @see ErrorResponse.Fields#exceptionClass
*/
@Nullable
public String getExceptionClass() {
return obtainDirect(FIELD_ExceptionClass, String.class, GetMode.STRICT);
}
/**
* Setter for exceptionClass
*
* @see ErrorResponse.Fields#exceptionClass
*/
public ErrorResponse setExceptionClass(String value, SetMode mode) {
putDirect(FIELD_ExceptionClass, String.class, String.class, value, mode);
return this;
}
/**
* Setter for exceptionClass
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see ErrorResponse.Fields#exceptionClass
*/
public ErrorResponse setExceptionClass(
@Nonnull
String value) {
putDirect(FIELD_ExceptionClass, String.class, String.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for stackTrace
*
* @see ErrorResponse.Fields#stackTrace
*/
public boolean hasStackTrace() {
return contains(FIELD_StackTrace);
}
/**
* Remover for stackTrace
*
* @see ErrorResponse.Fields#stackTrace
*/
public void removeStackTrace() {
remove(FIELD_StackTrace);
}
/**
* Getter for stackTrace
*
* @see ErrorResponse.Fields#stackTrace
*/
public String getStackTrace(GetMode mode) {
return obtainDirect(FIELD_StackTrace, String.class, mode);
}
/**
* Getter for stackTrace
*
* @return
* Optional field. Always check for null.
* @see ErrorResponse.Fields#stackTrace
*/
@Nullable
public String getStackTrace() {
return obtainDirect(FIELD_StackTrace, String.class, GetMode.STRICT);
}
/**
* Setter for stackTrace
*
* @see ErrorResponse.Fields#stackTrace
*/
public ErrorResponse setStackTrace(String value, SetMode mode) {
putDirect(FIELD_StackTrace, String.class, String.class, value, mode);
return this;
}
/**
* Setter for stackTrace
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see ErrorResponse.Fields#stackTrace
*/
public ErrorResponse setStackTrace(
@Nonnull
String value) {
putDirect(FIELD_StackTrace, String.class, String.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for errorDetails
*
* @see ErrorResponse.Fields#errorDetails
*/
public boolean hasErrorDetails() {
return contains(FIELD_ErrorDetails);
}
/**
* Remover for errorDetails
*
* @see ErrorResponse.Fields#errorDetails
*/
public void removeErrorDetails() {
remove(FIELD_ErrorDetails);
}
/**
* Getter for errorDetails
*
* @see ErrorResponse.Fields#errorDetails
*/
public ErrorDetails getErrorDetails(GetMode mode) {
return obtainWrapped(FIELD_ErrorDetails, ErrorDetails.class, mode);
}
/**
* Getter for errorDetails
*
* @return
* Optional field. Always check for null.
* @see ErrorResponse.Fields#errorDetails
*/
@Nullable
public ErrorDetails getErrorDetails() {
return obtainWrapped(FIELD_ErrorDetails, ErrorDetails.class, GetMode.STRICT);
}
/**
* Setter for errorDetails
*
* @see ErrorResponse.Fields#errorDetails
*/
public ErrorResponse setErrorDetails(ErrorDetails value, SetMode mode) {
putWrapped(FIELD_ErrorDetails, ErrorDetails.class, value, mode);
return this;
}
/**
* Setter for errorDetails
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see ErrorResponse.Fields#errorDetails
*/
public ErrorResponse setErrorDetails(
@Nonnull
ErrorDetails value) {
putWrapped(FIELD_ErrorDetails, ErrorDetails.class, value, SetMode.DISALLOW_NULL);
return this;
}
@Override
public ErrorResponse clone()
throws CloneNotSupportedException
{
return ((ErrorResponse) super.clone());
}
@Override
public ErrorResponse copy()
throws CloneNotSupportedException
{
return ((ErrorResponse) super.copy());
}
public static class Fields
extends PathSpec
{
public Fields(List path, String name) {
super(path, name);
}
public Fields() {
super();
}
/**
* The HTTP status code
*
*/
public PathSpec status() {
return new PathSpec(getPathComponents(), "status");
}
/**
* An service-specific error code (documented in prose)
*
*/
public PathSpec serviceErrorCode() {
return new PathSpec(getPathComponents(), "serviceErrorCode");
}
/**
* A human-readable explanation of the error
*
*/
public PathSpec message() {
return new PathSpec(getPathComponents(), "message");
}
/**
* The FQCN of the exception thrown by the server (included the case of a server fault)
*
*/
public PathSpec exceptionClass() {
return new PathSpec(getPathComponents(), "exceptionClass");
}
/**
* The full (??) stack trace (included the case of a server fault)
*
*/
public PathSpec stackTrace() {
return new PathSpec(getPathComponents(), "stackTrace");
}
public com.linkedin.restli.common.ErrorDetails.Fields errorDetails() {
return new com.linkedin.restli.common.ErrorDetails.Fields(getPathComponents(), "errorDetails");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy