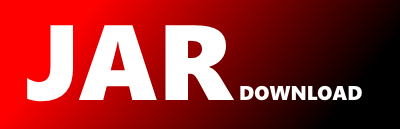
com.linkedin.restli.common.multiplexer.IndividualRequest Maven / Gradle / Ivy
package com.linkedin.restli.common.multiplexer;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.linkedin.data.DataMap;
import com.linkedin.data.schema.PathSpec;
import com.linkedin.data.schema.RecordDataSchema;
import com.linkedin.data.template.DataTemplateUtil;
import com.linkedin.data.template.GetMode;
import com.linkedin.data.template.RecordTemplate;
import com.linkedin.data.template.SetMode;
import com.linkedin.data.template.StringMap;
/**
* Individual HTTP request within a multiplexed request. For security reasons, cookies are not allowed to be specified in the IndividualRequest. Instead, it MUST be specified in the top level envelope request.
*
*/
@Generated(value = "com.linkedin.pegasus.generator.JavaCodeUtil", comments = "Rest.li Data Template. Generated from /Users/mnchen/dev/pegasus_trunk/pegasus/restli-common/src/main/pegasus/com/linkedin/restli/common/multiplexer/IndividualRequest.pdsc.", date = "Tue Oct 03 15:15:24 PDT 2017")
public class IndividualRequest
extends RecordTemplate
{
private final static IndividualRequest.Fields _fields = new IndividualRequest.Fields();
private final static RecordDataSchema SCHEMA = ((RecordDataSchema) DataTemplateUtil.parseSchema("{\"type\":\"record\",\"name\":\"IndividualRequest\",\"namespace\":\"com.linkedin.restli.common.multiplexer\",\"doc\":\"Individual HTTP request within a multiplexed request. For security reasons, cookies are not allowed to be specified in the IndividualRequest. Instead, it MUST be specified in the top level envelope request.\",\"fields\":[{\"name\":\"method\",\"type\":\"string\",\"doc\":\"HTTP method name\"},{\"name\":\"headers\",\"type\":{\"type\":\"map\",\"values\":\"string\"},\"doc\":\"HTTP headers specific to the individual request. All common headers should be specified in the top level envelope request. If IndividualRequest headers contain a header that is also specified in the top level envelope request, the header in the IndividualRequest will be used. In additions, for security reasons, headers in IndividualRequest are whitelisted. Only headers within the whitelist can be specified here.\",\"default\":{}},{\"name\":\"relativeUrl\",\"type\":\"string\",\"doc\":\"Relative URL of the request\"},{\"name\":\"body\",\"type\":{\"type\":\"record\",\"name\":\"IndividualBody\",\"doc\":\"Represents content that may be in the body of an individual request / response\",\"fields\":[]},\"doc\":\"Request body\",\"optional\":true},{\"name\":\"dependentRequests\",\"type\":{\"type\":\"map\",\"values\":\"IndividualRequest\"},\"doc\":\"Requests that should be executed after the current request is processed (sequential ordering). Dependent requests are executed in parallel. Keys of the dependent requests are used to correlate responses with requests. They should be unique within the multiplexed request\",\"default\":{}}]}"));
private final static RecordDataSchema.Field FIELD_Method = SCHEMA.getField("method");
private final static RecordDataSchema.Field FIELD_Headers = SCHEMA.getField("headers");
private final static RecordDataSchema.Field FIELD_RelativeUrl = SCHEMA.getField("relativeUrl");
private final static RecordDataSchema.Field FIELD_Body = SCHEMA.getField("body");
private final static RecordDataSchema.Field FIELD_DependentRequests = SCHEMA.getField("dependentRequests");
public IndividualRequest() {
super(new DataMap(), SCHEMA);
}
public IndividualRequest(DataMap data) {
super(data, SCHEMA);
}
public static IndividualRequest.Fields fields() {
return _fields;
}
/**
* Existence checker for method
*
* @see IndividualRequest.Fields#method
*/
public boolean hasMethod() {
return contains(FIELD_Method);
}
/**
* Remover for method
*
* @see IndividualRequest.Fields#method
*/
public void removeMethod() {
remove(FIELD_Method);
}
/**
* Getter for method
*
* @see IndividualRequest.Fields#method
*/
public String getMethod(GetMode mode) {
return obtainDirect(FIELD_Method, String.class, mode);
}
/**
* Getter for method
*
* @return
* Required field. Could be null for partial record.
* @see IndividualRequest.Fields#method
*/
@Nonnull
public String getMethod() {
return obtainDirect(FIELD_Method, String.class, GetMode.STRICT);
}
/**
* Setter for method
*
* @see IndividualRequest.Fields#method
*/
public IndividualRequest setMethod(String value, SetMode mode) {
putDirect(FIELD_Method, String.class, String.class, value, mode);
return this;
}
/**
* Setter for method
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see IndividualRequest.Fields#method
*/
public IndividualRequest setMethod(
@Nonnull
String value) {
putDirect(FIELD_Method, String.class, String.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for headers
*
* @see IndividualRequest.Fields#headers
*/
public boolean hasHeaders() {
return contains(FIELD_Headers);
}
/**
* Remover for headers
*
* @see IndividualRequest.Fields#headers
*/
public void removeHeaders() {
remove(FIELD_Headers);
}
/**
* Getter for headers
*
* @see IndividualRequest.Fields#headers
*/
public StringMap getHeaders(GetMode mode) {
return obtainWrapped(FIELD_Headers, StringMap.class, mode);
}
/**
* Getter for headers
*
* @return
* Required field. Could be null for partial record.
* @see IndividualRequest.Fields#headers
*/
@Nonnull
public StringMap getHeaders() {
return obtainWrapped(FIELD_Headers, StringMap.class, GetMode.STRICT);
}
/**
* Setter for headers
*
* @see IndividualRequest.Fields#headers
*/
public IndividualRequest setHeaders(StringMap value, SetMode mode) {
putWrapped(FIELD_Headers, StringMap.class, value, mode);
return this;
}
/**
* Setter for headers
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see IndividualRequest.Fields#headers
*/
public IndividualRequest setHeaders(
@Nonnull
StringMap value) {
putWrapped(FIELD_Headers, StringMap.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for relativeUrl
*
* @see IndividualRequest.Fields#relativeUrl
*/
public boolean hasRelativeUrl() {
return contains(FIELD_RelativeUrl);
}
/**
* Remover for relativeUrl
*
* @see IndividualRequest.Fields#relativeUrl
*/
public void removeRelativeUrl() {
remove(FIELD_RelativeUrl);
}
/**
* Getter for relativeUrl
*
* @see IndividualRequest.Fields#relativeUrl
*/
public String getRelativeUrl(GetMode mode) {
return obtainDirect(FIELD_RelativeUrl, String.class, mode);
}
/**
* Getter for relativeUrl
*
* @return
* Required field. Could be null for partial record.
* @see IndividualRequest.Fields#relativeUrl
*/
@Nonnull
public String getRelativeUrl() {
return obtainDirect(FIELD_RelativeUrl, String.class, GetMode.STRICT);
}
/**
* Setter for relativeUrl
*
* @see IndividualRequest.Fields#relativeUrl
*/
public IndividualRequest setRelativeUrl(String value, SetMode mode) {
putDirect(FIELD_RelativeUrl, String.class, String.class, value, mode);
return this;
}
/**
* Setter for relativeUrl
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see IndividualRequest.Fields#relativeUrl
*/
public IndividualRequest setRelativeUrl(
@Nonnull
String value) {
putDirect(FIELD_RelativeUrl, String.class, String.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for body
*
* @see IndividualRequest.Fields#body
*/
public boolean hasBody() {
return contains(FIELD_Body);
}
/**
* Remover for body
*
* @see IndividualRequest.Fields#body
*/
public void removeBody() {
remove(FIELD_Body);
}
/**
* Getter for body
*
* @see IndividualRequest.Fields#body
*/
public IndividualBody getBody(GetMode mode) {
return obtainWrapped(FIELD_Body, IndividualBody.class, mode);
}
/**
* Getter for body
*
* @return
* Optional field. Always check for null.
* @see IndividualRequest.Fields#body
*/
@Nullable
public IndividualBody getBody() {
return obtainWrapped(FIELD_Body, IndividualBody.class, GetMode.STRICT);
}
/**
* Setter for body
*
* @see IndividualRequest.Fields#body
*/
public IndividualRequest setBody(IndividualBody value, SetMode mode) {
putWrapped(FIELD_Body, IndividualBody.class, value, mode);
return this;
}
/**
* Setter for body
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see IndividualRequest.Fields#body
*/
public IndividualRequest setBody(
@Nonnull
IndividualBody value) {
putWrapped(FIELD_Body, IndividualBody.class, value, SetMode.DISALLOW_NULL);
return this;
}
/**
* Existence checker for dependentRequests
*
* @see IndividualRequest.Fields#dependentRequests
*/
public boolean hasDependentRequests() {
return contains(FIELD_DependentRequests);
}
/**
* Remover for dependentRequests
*
* @see IndividualRequest.Fields#dependentRequests
*/
public void removeDependentRequests() {
remove(FIELD_DependentRequests);
}
/**
* Getter for dependentRequests
*
* @see IndividualRequest.Fields#dependentRequests
*/
public IndividualRequestMap getDependentRequests(GetMode mode) {
return obtainWrapped(FIELD_DependentRequests, IndividualRequestMap.class, mode);
}
/**
* Getter for dependentRequests
*
* @return
* Required field. Could be null for partial record.
* @see IndividualRequest.Fields#dependentRequests
*/
@Nonnull
public IndividualRequestMap getDependentRequests() {
return obtainWrapped(FIELD_DependentRequests, IndividualRequestMap.class, GetMode.STRICT);
}
/**
* Setter for dependentRequests
*
* @see IndividualRequest.Fields#dependentRequests
*/
public IndividualRequest setDependentRequests(IndividualRequestMap value, SetMode mode) {
putWrapped(FIELD_DependentRequests, IndividualRequestMap.class, value, mode);
return this;
}
/**
* Setter for dependentRequests
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see IndividualRequest.Fields#dependentRequests
*/
public IndividualRequest setDependentRequests(
@Nonnull
IndividualRequestMap value) {
putWrapped(FIELD_DependentRequests, IndividualRequestMap.class, value, SetMode.DISALLOW_NULL);
return this;
}
@Override
public IndividualRequest clone()
throws CloneNotSupportedException
{
return ((IndividualRequest) super.clone());
}
@Override
public IndividualRequest copy()
throws CloneNotSupportedException
{
return ((IndividualRequest) super.copy());
}
public static class Fields
extends PathSpec
{
public Fields(List path, String name) {
super(path, name);
}
public Fields() {
super();
}
/**
* HTTP method name
*
*/
public PathSpec method() {
return new PathSpec(getPathComponents(), "method");
}
/**
* HTTP headers specific to the individual request. All common headers should be specified in the top level envelope request. If IndividualRequest headers contain a header that is also specified in the top level envelope request, the header in the IndividualRequest will be used. In additions, for security reasons, headers in IndividualRequest are whitelisted. Only headers within the whitelist can be specified here.
*
*/
public PathSpec headers() {
return new PathSpec(getPathComponents(), "headers");
}
/**
* Relative URL of the request
*
*/
public PathSpec relativeUrl() {
return new PathSpec(getPathComponents(), "relativeUrl");
}
/**
* Request body
*
*/
public com.linkedin.restli.common.multiplexer.IndividualBody.Fields body() {
return new com.linkedin.restli.common.multiplexer.IndividualBody.Fields(getPathComponents(), "body");
}
/**
* Requests that should be executed after the current request is processed (sequential ordering). Dependent requests are executed in parallel. Keys of the dependent requests are used to correlate responses with requests. They should be unique within the multiplexed request
*
*/
public com.linkedin.restli.common.multiplexer.IndividualRequestMap.Fields dependentRequests() {
return new com.linkedin.restli.common.multiplexer.IndividualRequestMap.Fields(getPathComponents(), "dependentRequests");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy