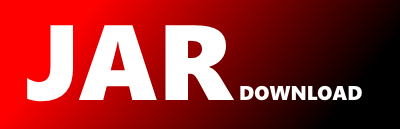
com.linkedin.restli.restspec.CustomAnnotationSchema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restli-common Show documentation
Show all versions of restli-common Show documentation
Pegasus is a framework for building robust, scalable service architectures using dynamic discovery and simple asychronous type-checked REST + JSON APIs.
package com.linkedin.restli.restspec;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.linkedin.data.DataMap;
import com.linkedin.data.schema.PathSpec;
import com.linkedin.data.schema.RecordDataSchema;
import com.linkedin.data.template.DataTemplateUtil;
import com.linkedin.data.template.GetMode;
import com.linkedin.data.template.RecordTemplate;
import com.linkedin.data.template.SetMode;
/**
* Custom annotation for idl
*
*/
@Generated(value = "com.linkedin.pegasus.generator.JavaCodeUtil", comments = "Rest.li Data Template. Generated from /Users/mnchen/dev/pegasus_trunk/pegasus/restli-common/src/main/pegasus/com/linkedin/restli/restspec/CustomAnnotationSchema.pdsc.", date = "Tue Oct 03 15:15:24 PDT 2017")
public class CustomAnnotationSchema
extends RecordTemplate
{
private final static CustomAnnotationSchema.Fields _fields = new CustomAnnotationSchema.Fields();
private final static RecordDataSchema SCHEMA = ((RecordDataSchema) DataTemplateUtil.parseSchema("{\"type\":\"record\",\"name\":\"CustomAnnotationSchema\",\"namespace\":\"com.linkedin.restli.restspec\",\"doc\":\"Custom annotation for idl\",\"fields\":[{\"name\":\"annotations\",\"type\":{\"type\":\"map\",\"values\":{\"type\":\"record\",\"name\":\"CustomAnnotationContentSchema\",\"doc\":\"Unstructured record that represents arbitrary custom annotations for idl. Actual content is always a map with annotation's overridable member name as key and member value as value\",\"fields\":[]}},\"doc\":\"custom annotation data\",\"optional\":true}]}"));
private final static RecordDataSchema.Field FIELD_Annotations = SCHEMA.getField("annotations");
public CustomAnnotationSchema() {
super(new DataMap(), SCHEMA);
}
public CustomAnnotationSchema(DataMap data) {
super(data, SCHEMA);
}
public static CustomAnnotationSchema.Fields fields() {
return _fields;
}
/**
* Existence checker for annotations
*
* @see CustomAnnotationSchema.Fields#annotations
*/
public boolean hasAnnotations() {
return contains(FIELD_Annotations);
}
/**
* Remover for annotations
*
* @see CustomAnnotationSchema.Fields#annotations
*/
public void removeAnnotations() {
remove(FIELD_Annotations);
}
/**
* Getter for annotations
*
* @see CustomAnnotationSchema.Fields#annotations
*/
public CustomAnnotationContentSchemaMap getAnnotations(GetMode mode) {
return obtainWrapped(FIELD_Annotations, CustomAnnotationContentSchemaMap.class, mode);
}
/**
* Getter for annotations
*
* @return
* Optional field. Always check for null.
* @see CustomAnnotationSchema.Fields#annotations
*/
@Nullable
public CustomAnnotationContentSchemaMap getAnnotations() {
return obtainWrapped(FIELD_Annotations, CustomAnnotationContentSchemaMap.class, GetMode.STRICT);
}
/**
* Setter for annotations
*
* @see CustomAnnotationSchema.Fields#annotations
*/
public CustomAnnotationSchema setAnnotations(CustomAnnotationContentSchemaMap value, SetMode mode) {
putWrapped(FIELD_Annotations, CustomAnnotationContentSchemaMap.class, value, mode);
return this;
}
/**
* Setter for annotations
*
* @param value
* Must not be null. For more control, use setters with mode instead.
* @see CustomAnnotationSchema.Fields#annotations
*/
public CustomAnnotationSchema setAnnotations(
@Nonnull
CustomAnnotationContentSchemaMap value) {
putWrapped(FIELD_Annotations, CustomAnnotationContentSchemaMap.class, value, SetMode.DISALLOW_NULL);
return this;
}
@Override
public CustomAnnotationSchema clone()
throws CloneNotSupportedException
{
return ((CustomAnnotationSchema) super.clone());
}
@Override
public CustomAnnotationSchema copy()
throws CloneNotSupportedException
{
return ((CustomAnnotationSchema) super.copy());
}
public static class Fields
extends PathSpec
{
public Fields(List path, String name) {
super(path, name);
}
public Fields() {
super();
}
/**
* custom annotation data
*
*/
public com.linkedin.restli.restspec.CustomAnnotationContentSchemaMap.Fields annotations() {
return new com.linkedin.restli.restspec.CustomAnnotationContentSchemaMap.Fields(getPathComponents(), "annotations");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy