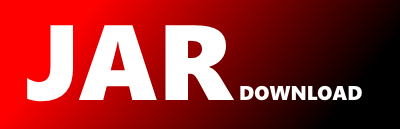
com.linkedin.restli.internal.server.methods.response.AbstractBatchResponseBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restli-server Show documentation
Show all versions of restli-server Show documentation
Pegasus is a framework for building robust, scalable service architectures using dynamic discovery and simple asychronous type-checked REST + JSON APIs.
/*
Copyright (c) 2012 LinkedIn Corp.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
/**
* $Id: $
*/
package com.linkedin.restli.internal.server.methods.response;
import java.io.IOException;
import java.util.Map;
import com.linkedin.data.DataMap;
import com.linkedin.data.template.RecordTemplate;
import com.linkedin.r2.message.rest.RestRequest;
import com.linkedin.restli.common.BatchResponse;
import com.linkedin.restli.common.ErrorResponse;
import com.linkedin.restli.common.RestConstants;
import com.linkedin.restli.internal.server.RoutingResult;
import com.linkedin.restli.internal.server.ServerResourceContext;
import com.linkedin.restli.server.ResourceContext;
import com.linkedin.restli.server.RestLiServiceException;
/**
* @author Josh Walker
* @version $Revision: $
*/
public abstract class AbstractBatchResponseBuilder
{
ErrorResponseBuilder _errorResponseBuilder;
protected AbstractBatchResponseBuilder(ErrorResponseBuilder errorResponseBuilder)
{
_errorResponseBuilder = errorResponseBuilder;
}
protected void populateErrors(final RestRequest request,
final RoutingResult routingResult,
final Map, RestLiServiceException> map,
final Map headers,
final BatchResponse extends RecordTemplate> batchResponse) throws IOException
{
for (Map.Entry, RestLiServiceException> entry : ((ServerResourceContext) routingResult.getContext()).getBatchKeyErrors()
.entrySet())
{
DataMap errorData =
_errorResponseBuilder.buildResponse(request, routingResult, entry.getValue(), headers)
.getDataMap();
batchResponse.getErrors().put(entry.getKey().toString(),
new ErrorResponse(errorData));
}
for (Map.Entry, RestLiServiceException> entry : map.entrySet())
{
DataMap errorData =
_errorResponseBuilder.buildResponse(request, routingResult, entry.getValue(), headers)
.getDataMap();
batchResponse.getErrors().put(entry.getKey().toString(),
new ErrorResponse(errorData));
}
}
protected void populateResults(final BatchResponse> response,
final Map, ? extends V> resultsMap,
final Map headers,
final Class extends RecordTemplate> valueClass,
final ResourceContext resourceContext)
{
DataMap dataMap = (DataMap) response.data().get(BatchResponse.RESULTS);
for (Map.Entry, ? extends V> entry: resultsMap.entrySet())
{
DataMap data = buildResultRecord(entry.getValue(), resourceContext);
dataMap.put(entry.getKey().toString(), data);
}
headers.put(RestConstants.HEADER_LINKEDIN_TYPE, BatchResponse.class.getName());
headers.put(RestConstants.HEADER_LINKEDIN_SUB_TYPE, valueClass.getName());
}
protected BatchResponse createBatchResponse(final Class clazz,
final int resultsCapacity,
final int errorsCapacity)
{
return new BatchResponse(clazz, resultsCapacity, errorsCapacity);
}
/**
* Subclasses must override this method to convert an application result into a
* RecordTemplate representation to be sent in the BatchResponse.
*
* This method is called by populateResults for each value in the resultsMap
*
* @param o - the object to be converted
* @return a RecordTemplate representation of the converted object
*/
protected abstract DataMap buildResultRecord(V o, ResourceContext resourceContext);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy