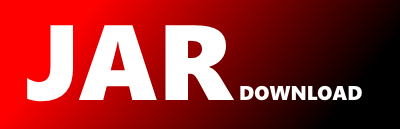
proj.zoie.hourglass.impl.Box Maven / Gradle / Ivy
package proj.zoie.hourglass.impl;
import java.io.IOException;
import java.util.LinkedList;
import java.util.List;
import org.apache.log4j.Logger;
import org.apache.lucene.index.IndexReader;
import proj.zoie.api.ZoieException;
import proj.zoie.api.ZoieIndexReader;
import proj.zoie.api.indexing.IndexReaderDecorator;
import proj.zoie.impl.indexing.ZoieSystem;
public class Box
{
public static final Logger log = Logger.getLogger(Box.class.getName());
List> _archives;
List> _archiveZoies;
List> _retiree;
List> _actives;
IndexReaderDecorator _decorator;
/**
* Copy the given lists to have immutable behavior.
*
* @param archives
* @param retiree
* @param actives
* @param decorator
*/
public Box(List> archives, List> archiveZoies, List> retiree, List> actives, IndexReaderDecorator decorator)
{
_archives = new LinkedList>(archives);
_archiveZoies = new LinkedList>(archiveZoies);
_retiree = new LinkedList>(retiree);
_actives = new LinkedList>(actives);
_decorator = decorator;
if (log.isDebugEnabled())
{
for (ZoieIndexReader r : _archives)
{
log.debug("archive " + r.directory() + " refCount: " + r.getRefCount());
}
}
}
public void shutdown()
{
for (ZoieIndexReader r : _archives)
{
r.decZoieRef();
log.info("refCount at shutdown: " + r.getRefCount() + " " + r.directory());
}
for (ZoieSystem zoie : _archiveZoies)
{
zoie.shutdown();
}
for (ZoieSystem zoie : _retiree)
{
zoie.shutdown();
}
// add the active index readers
for (ZoieSystem zoie : _actives)
{
while (true)
{
long flushwait = 200000L;
try
{
zoie.flushEvents(flushwait);
zoie.getAdminMBean().setUseCompoundFile(true);
zoie.getAdminMBean().optimize(1);
break;
} catch (IOException e)
{
log.error("pre-shutdown optimization " + zoie.getAdminMBean().getIndexDir() + " Should investigate. But move on now.", e);
break;
} catch (ZoieException e)
{
if (e.getMessage().indexOf("timed out") < 0)
{
break;
} else
{
log.info("pre-shutdown optimization " + zoie.getAdminMBean().getIndexDir() + " flushing processing " + flushwait + "ms elapsed");
}
}
}
zoie.shutdown();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy