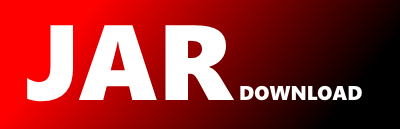
com.lithic.api.core.ClientOptions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lithic-kotlin-core Show documentation
Show all versions of lithic-kotlin-core Show documentation
The Lithic Developer API is designed to provide a predictable programmatic
interface for accessing your Lithic account through an API and transaction
webhooks. Note that your API key is a secret and should be treated as such.
Don't share it with anyone, including us. We will never ask you for it.
The newest version!
// File generated from our OpenAPI spec by Stainless.
package com.lithic.api.core
import com.fasterxml.jackson.databind.json.JsonMapper
import com.lithic.api.core.http.Headers
import com.lithic.api.core.http.HttpClient
import com.lithic.api.core.http.PhantomReachableClosingHttpClient
import com.lithic.api.core.http.QueryParams
import com.lithic.api.core.http.RetryingHttpClient
import java.time.Clock
class ClientOptions
private constructor(
private val originalHttpClient: HttpClient,
val httpClient: HttpClient,
val jsonMapper: JsonMapper,
val clock: Clock,
val baseUrl: String,
val headers: Headers,
val queryParams: QueryParams,
val responseValidation: Boolean,
val maxRetries: Int,
val apiKey: String,
val webhookSecret: String?,
) {
fun toBuilder() = Builder().from(this)
companion object {
const val PRODUCTION_URL = "https://api.lithic.com"
const val SANDBOX_URL = "https://sandbox.lithic.com"
fun builder() = Builder()
fun fromEnv(): ClientOptions = builder().fromEnv().build()
}
class Builder {
private var httpClient: HttpClient? = null
private var jsonMapper: JsonMapper = jsonMapper()
private var clock: Clock = Clock.systemUTC()
private var baseUrl: String = PRODUCTION_URL
private var headers: Headers.Builder = Headers.builder()
private var queryParams: QueryParams.Builder = QueryParams.builder()
private var responseValidation: Boolean = false
private var maxRetries: Int = 2
private var apiKey: String? = null
private var webhookSecret: String? = null
internal fun from(clientOptions: ClientOptions) = apply {
httpClient = clientOptions.originalHttpClient
jsonMapper = clientOptions.jsonMapper
clock = clientOptions.clock
baseUrl = clientOptions.baseUrl
headers = clientOptions.headers.toBuilder()
queryParams = clientOptions.queryParams.toBuilder()
responseValidation = clientOptions.responseValidation
maxRetries = clientOptions.maxRetries
apiKey = clientOptions.apiKey
webhookSecret = clientOptions.webhookSecret
}
fun httpClient(httpClient: HttpClient) = apply { this.httpClient = httpClient }
fun jsonMapper(jsonMapper: JsonMapper) = apply { this.jsonMapper = jsonMapper }
fun clock(clock: Clock) = apply { this.clock = clock }
fun baseUrl(baseUrl: String) = apply { this.baseUrl = baseUrl }
fun headers(headers: Headers) = apply {
this.headers.clear()
putAllHeaders(headers)
}
fun headers(headers: Map>) = apply {
this.headers.clear()
putAllHeaders(headers)
}
fun putHeader(name: String, value: String) = apply { headers.put(name, value) }
fun putHeaders(name: String, values: Iterable) = apply { headers.put(name, values) }
fun putAllHeaders(headers: Headers) = apply { this.headers.putAll(headers) }
fun putAllHeaders(headers: Map>) = apply {
this.headers.putAll(headers)
}
fun replaceHeaders(name: String, value: String) = apply { headers.replace(name, value) }
fun replaceHeaders(name: String, values: Iterable) = apply {
headers.replace(name, values)
}
fun replaceAllHeaders(headers: Headers) = apply { this.headers.replaceAll(headers) }
fun replaceAllHeaders(headers: Map>) = apply {
this.headers.replaceAll(headers)
}
fun removeHeaders(name: String) = apply { headers.remove(name) }
fun removeAllHeaders(names: Set) = apply { headers.removeAll(names) }
fun queryParams(queryParams: QueryParams) = apply {
this.queryParams.clear()
putAllQueryParams(queryParams)
}
fun queryParams(queryParams: Map>) = apply {
this.queryParams.clear()
putAllQueryParams(queryParams)
}
fun putQueryParam(key: String, value: String) = apply { queryParams.put(key, value) }
fun putQueryParams(key: String, values: Iterable) = apply {
queryParams.put(key, values)
}
fun putAllQueryParams(queryParams: QueryParams) = apply {
this.queryParams.putAll(queryParams)
}
fun putAllQueryParams(queryParams: Map>) = apply {
this.queryParams.putAll(queryParams)
}
fun replaceQueryParams(key: String, value: String) = apply {
queryParams.replace(key, value)
}
fun replaceQueryParams(key: String, values: Iterable) = apply {
queryParams.replace(key, values)
}
fun replaceAllQueryParams(queryParams: QueryParams) = apply {
this.queryParams.replaceAll(queryParams)
}
fun replaceAllQueryParams(queryParams: Map>) = apply {
this.queryParams.replaceAll(queryParams)
}
fun removeQueryParams(key: String) = apply { queryParams.remove(key) }
fun removeAllQueryParams(keys: Set) = apply { queryParams.removeAll(keys) }
fun responseValidation(responseValidation: Boolean) = apply {
this.responseValidation = responseValidation
}
fun maxRetries(maxRetries: Int) = apply { this.maxRetries = maxRetries }
fun apiKey(apiKey: String) = apply { this.apiKey = apiKey }
fun webhookSecret(webhookSecret: String?) = apply { this.webhookSecret = webhookSecret }
fun fromEnv() = apply {
System.getenv("LITHIC_API_KEY")?.let { apiKey(it) }
System.getenv("LITHIC_WEBHOOK_SECRET")?.let { webhookSecret(it) }
}
fun build(): ClientOptions {
checkNotNull(httpClient) { "`httpClient` is required but was not set" }
checkNotNull(apiKey) { "`apiKey` is required but was not set" }
val headers = Headers.builder()
val queryParams = QueryParams.builder()
headers.put("X-Stainless-Lang", "kotlin")
headers.put("X-Stainless-Arch", getOsArch())
headers.put("X-Stainless-OS", getOsName())
headers.put("X-Stainless-OS-Version", getOsVersion())
headers.put("X-Stainless-Package-Version", getPackageVersion())
headers.put("X-Stainless-Runtime", "JRE")
headers.put("X-Stainless-Runtime-Version", getJavaVersion())
headers.put("X-Lithic-Pagination", "cursor")
apiKey?.let {
if (!it.isEmpty()) {
headers.put("Authorization", it)
}
}
headers.replaceAll(this.headers.build())
queryParams.replaceAll(this.queryParams.build())
return ClientOptions(
httpClient!!,
PhantomReachableClosingHttpClient(
RetryingHttpClient.builder()
.httpClient(httpClient!!)
.clock(clock)
.maxRetries(maxRetries)
.build()
),
jsonMapper,
clock,
baseUrl,
headers.build(),
queryParams.build(),
responseValidation,
maxRetries,
apiKey!!,
webhookSecret,
)
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy