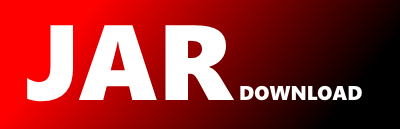
com.lithic.api.models.PrimeRateRetrieveResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lithic-kotlin-core Show documentation
Show all versions of lithic-kotlin-core Show documentation
The Lithic Developer API is designed to provide a predictable programmatic
interface for accessing your Lithic account through an API and transaction
webhooks. Note that your API key is a secret and should be treated as such.
Don't share it with anyone, including us. We will never ask you for it.
The newest version!
// File generated from our OpenAPI spec by Stainless.
package com.lithic.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.lithic.api.core.ExcludeMissing
import com.lithic.api.core.JsonField
import com.lithic.api.core.JsonMissing
import com.lithic.api.core.JsonValue
import com.lithic.api.core.NoAutoDetect
import com.lithic.api.core.toImmutable
import java.time.LocalDate
import java.util.Objects
@JsonDeserialize(builder = PrimeRateRetrieveResponse.Builder::class)
@NoAutoDetect
class PrimeRateRetrieveResponse
private constructor(
private val data: JsonField>,
private val hasMore: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** List of prime rates */
fun data(): List = data.getRequired("data")
/** Whether there are more prime rates */
fun hasMore(): Boolean = hasMore.getRequired("has_more")
/** List of prime rates */
@JsonProperty("data") @ExcludeMissing fun _data() = data
/** Whether there are more prime rates */
@JsonProperty("has_more") @ExcludeMissing fun _hasMore() = hasMore
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): PrimeRateRetrieveResponse = apply {
if (!validated) {
data().forEach { it.validate() }
hasMore()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var data: JsonField> = JsonMissing.of()
private var hasMore: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(primeRateRetrieveResponse: PrimeRateRetrieveResponse) = apply {
this.data = primeRateRetrieveResponse.data
this.hasMore = primeRateRetrieveResponse.hasMore
additionalProperties(primeRateRetrieveResponse.additionalProperties)
}
/** List of prime rates */
fun data(data: List) = data(JsonField.of(data))
/** List of prime rates */
@JsonProperty("data")
@ExcludeMissing
fun data(data: JsonField>) = apply { this.data = data }
/** Whether there are more prime rates */
fun hasMore(hasMore: Boolean) = hasMore(JsonField.of(hasMore))
/** Whether there are more prime rates */
@JsonProperty("has_more")
@ExcludeMissing
fun hasMore(hasMore: JsonField) = apply { this.hasMore = hasMore }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): PrimeRateRetrieveResponse =
PrimeRateRetrieveResponse(
data.map { it.toImmutable() },
hasMore,
additionalProperties.toImmutable(),
)
}
@JsonDeserialize(builder = InterestRate.Builder::class)
@NoAutoDetect
class InterestRate
private constructor(
private val effectiveDate: JsonField,
private val rate: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** Date the rate goes into effect */
fun effectiveDate(): LocalDate = effectiveDate.getRequired("effective_date")
/** The rate in decimal format */
fun rate(): String = rate.getRequired("rate")
/** Date the rate goes into effect */
@JsonProperty("effective_date") @ExcludeMissing fun _effectiveDate() = effectiveDate
/** The rate in decimal format */
@JsonProperty("rate") @ExcludeMissing fun _rate() = rate
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): InterestRate = apply {
if (!validated) {
effectiveDate()
rate()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var effectiveDate: JsonField = JsonMissing.of()
private var rate: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(interestRate: InterestRate) = apply {
this.effectiveDate = interestRate.effectiveDate
this.rate = interestRate.rate
additionalProperties(interestRate.additionalProperties)
}
/** Date the rate goes into effect */
fun effectiveDate(effectiveDate: LocalDate) = effectiveDate(JsonField.of(effectiveDate))
/** Date the rate goes into effect */
@JsonProperty("effective_date")
@ExcludeMissing
fun effectiveDate(effectiveDate: JsonField) = apply {
this.effectiveDate = effectiveDate
}
/** The rate in decimal format */
fun rate(rate: String) = rate(JsonField.of(rate))
/** The rate in decimal format */
@JsonProperty("rate")
@ExcludeMissing
fun rate(rate: JsonField) = apply { this.rate = rate }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): InterestRate =
InterestRate(
effectiveDate,
rate,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is InterestRate && this.effectiveDate == other.effectiveDate && this.rate == other.rate && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(effectiveDate, rate, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"InterestRate{effectiveDate=$effectiveDate, rate=$rate, additionalProperties=$additionalProperties}"
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is PrimeRateRetrieveResponse && this.data == other.data && this.hasMore == other.hasMore && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(data, hasMore, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"PrimeRateRetrieveResponse{data=$data, hasMore=$hasMore, additionalProperties=$additionalProperties}"
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy