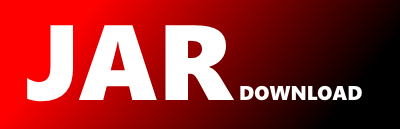
com.lithic.api.models.Transaction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lithic-kotlin-core Show documentation
Show all versions of lithic-kotlin-core Show documentation
The Lithic Developer API is designed to provide a predictable programmatic
interface for accessing your Lithic account through an API and transaction
webhooks. Note that your API key is a secret and should be treated as such.
Don't share it with anyone, including us. We will never ask you for it.
The newest version!
// File generated from our OpenAPI spec by Stainless.
package com.lithic.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonCreator
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.lithic.api.core.Enum
import com.lithic.api.core.ExcludeMissing
import com.lithic.api.core.JsonField
import com.lithic.api.core.JsonMissing
import com.lithic.api.core.JsonValue
import com.lithic.api.core.NoAutoDetect
import com.lithic.api.core.toImmutable
import com.lithic.api.errors.LithicInvalidDataException
import java.time.OffsetDateTime
import java.util.Objects
@JsonDeserialize(builder = Transaction.Builder::class)
@NoAutoDetect
class Transaction
private constructor(
private val acquirerFee: JsonField,
private val acquirerReferenceNumber: JsonField,
private val accountToken: JsonField,
private val amount: JsonField,
private val amounts: JsonField,
private val authorizationAmount: JsonField,
private val authorizationCode: JsonField,
private val avs: JsonField,
private val cardToken: JsonField,
private val cardholderAuthentication: JsonField,
private val created: JsonField,
private val events: JsonField>,
private val merchant: JsonField,
private val merchantAmount: JsonField,
private val merchantAuthorizationAmount: JsonField,
private val merchantCurrency: JsonField,
private val network: JsonField,
private val networkRiskScore: JsonField,
private val result: JsonField,
private val pos: JsonField,
private val settledAmount: JsonField,
private val status: JsonField,
private val token: JsonField,
private val tokenInfo: JsonField,
private val updated: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/**
* Fee assessed by the merchant and paid for by the cardholder in the smallest unit of the
* currency. Will be zero if no fee is assessed. Rebates may be transmitted as a negative value
* to indicate credited fees.
*/
fun acquirerFee(): Long? = acquirerFee.getNullable("acquirer_fee")
/**
* Unique identifier assigned to a transaction by the acquirer that can be used in dispute and
* chargeback filing.
*/
fun acquirerReferenceNumber(): String? =
acquirerReferenceNumber.getNullable("acquirer_reference_number")
/** The token for the account associated with this transaction. */
fun accountToken(): String = accountToken.getRequired("account_token")
/**
* When the transaction is pending, this represents the authorization amount of the transaction
* in the anticipated settlement currency. Once the transaction has settled, this field
* represents the settled amount in the settlement currency.
*/
fun amount(): Long = amount.getRequired("amount")
fun amounts(): TransactionAmounts = amounts.getRequired("amounts")
/** The authorization amount of the transaction in the anticipated settlement currency. */
fun authorizationAmount(): Long? = authorizationAmount.getNullable("authorization_amount")
/**
* A fixed-width 6-digit numeric identifier that can be used to identify a transaction with
* networks.
*/
fun authorizationCode(): String? = authorizationCode.getNullable("authorization_code")
fun avs(): Avs? = avs.getNullable("avs")
/** Token for the card used in this transaction. */
fun cardToken(): String = cardToken.getRequired("card_token")
fun cardholderAuthentication(): CardholderAuthentication? =
cardholderAuthentication.getNullable("cardholder_authentication")
/** Date and time when the transaction first occurred. UTC time zone. */
fun created(): OffsetDateTime = created.getRequired("created")
fun events(): List? = events.getNullable("events")
fun merchant(): Merchant = merchant.getRequired("merchant")
/** Analogous to the 'amount', but in the merchant currency. */
fun merchantAmount(): Long? = merchantAmount.getNullable("merchant_amount")
/** Analogous to the 'authorization_amount', but in the merchant currency. */
fun merchantAuthorizationAmount(): Long? =
merchantAuthorizationAmount.getNullable("merchant_authorization_amount")
/** 3-digit alphabetic ISO 4217 code for the local currency of the transaction. */
fun merchantCurrency(): String = merchantCurrency.getRequired("merchant_currency")
/**
* Card network of the authorization. Can be `INTERLINK`, `MAESTRO`, `MASTERCARD`, `VISA`, or
* `UNKNOWN`. Value is `UNKNOWN` when Lithic cannot determine the network code from the upstream
* provider.
*/
fun network(): Network? = network.getNullable("network")
/**
* Network-provided score assessing risk level associated with a given authorization. Scores are
* on a range of 0-999, with 0 representing the lowest risk and 999 representing the highest
* risk. For Visa transactions, where the raw score has a range of 0-99, Lithic will normalize
* the score by multiplying the raw score by 10x.
*/
fun networkRiskScore(): Long? = networkRiskScore.getNullable("network_risk_score")
fun result(): DeclineResult = result.getRequired("result")
fun pos(): Pos = pos.getRequired("pos")
/** The settled amount of the transaction in the settlement currency. */
fun settledAmount(): Long = settledAmount.getRequired("settled_amount")
/** Status of the transaction. */
fun status(): Status = status.getRequired("status")
/** Globally unique identifier. */
fun token(): String = token.getRequired("token")
fun tokenInfo(): TokenInfo? = tokenInfo.getNullable("token_info")
/** Date and time when the transaction last updated. UTC time zone. */
fun updated(): OffsetDateTime = updated.getRequired("updated")
/**
* Fee assessed by the merchant and paid for by the cardholder in the smallest unit of the
* currency. Will be zero if no fee is assessed. Rebates may be transmitted as a negative value
* to indicate credited fees.
*/
@JsonProperty("acquirer_fee") @ExcludeMissing fun _acquirerFee() = acquirerFee
/**
* Unique identifier assigned to a transaction by the acquirer that can be used in dispute and
* chargeback filing.
*/
@JsonProperty("acquirer_reference_number")
@ExcludeMissing
fun _acquirerReferenceNumber() = acquirerReferenceNumber
/** The token for the account associated with this transaction. */
@JsonProperty("account_token") @ExcludeMissing fun _accountToken() = accountToken
/**
* When the transaction is pending, this represents the authorization amount of the transaction
* in the anticipated settlement currency. Once the transaction has settled, this field
* represents the settled amount in the settlement currency.
*/
@JsonProperty("amount") @ExcludeMissing fun _amount() = amount
@JsonProperty("amounts") @ExcludeMissing fun _amounts() = amounts
/** The authorization amount of the transaction in the anticipated settlement currency. */
@JsonProperty("authorization_amount")
@ExcludeMissing
fun _authorizationAmount() = authorizationAmount
/**
* A fixed-width 6-digit numeric identifier that can be used to identify a transaction with
* networks.
*/
@JsonProperty("authorization_code") @ExcludeMissing fun _authorizationCode() = authorizationCode
@JsonProperty("avs") @ExcludeMissing fun _avs() = avs
/** Token for the card used in this transaction. */
@JsonProperty("card_token") @ExcludeMissing fun _cardToken() = cardToken
@JsonProperty("cardholder_authentication")
@ExcludeMissing
fun _cardholderAuthentication() = cardholderAuthentication
/** Date and time when the transaction first occurred. UTC time zone. */
@JsonProperty("created") @ExcludeMissing fun _created() = created
@JsonProperty("events") @ExcludeMissing fun _events() = events
@JsonProperty("merchant") @ExcludeMissing fun _merchant() = merchant
/** Analogous to the 'amount', but in the merchant currency. */
@JsonProperty("merchant_amount") @ExcludeMissing fun _merchantAmount() = merchantAmount
/** Analogous to the 'authorization_amount', but in the merchant currency. */
@JsonProperty("merchant_authorization_amount")
@ExcludeMissing
fun _merchantAuthorizationAmount() = merchantAuthorizationAmount
/** 3-digit alphabetic ISO 4217 code for the local currency of the transaction. */
@JsonProperty("merchant_currency") @ExcludeMissing fun _merchantCurrency() = merchantCurrency
/**
* Card network of the authorization. Can be `INTERLINK`, `MAESTRO`, `MASTERCARD`, `VISA`, or
* `UNKNOWN`. Value is `UNKNOWN` when Lithic cannot determine the network code from the upstream
* provider.
*/
@JsonProperty("network") @ExcludeMissing fun _network() = network
/**
* Network-provided score assessing risk level associated with a given authorization. Scores are
* on a range of 0-999, with 0 representing the lowest risk and 999 representing the highest
* risk. For Visa transactions, where the raw score has a range of 0-99, Lithic will normalize
* the score by multiplying the raw score by 10x.
*/
@JsonProperty("network_risk_score") @ExcludeMissing fun _networkRiskScore() = networkRiskScore
@JsonProperty("result") @ExcludeMissing fun _result() = result
@JsonProperty("pos") @ExcludeMissing fun _pos() = pos
/** The settled amount of the transaction in the settlement currency. */
@JsonProperty("settled_amount") @ExcludeMissing fun _settledAmount() = settledAmount
/** Status of the transaction. */
@JsonProperty("status") @ExcludeMissing fun _status() = status
/** Globally unique identifier. */
@JsonProperty("token") @ExcludeMissing fun _token() = token
@JsonProperty("token_info") @ExcludeMissing fun _tokenInfo() = tokenInfo
/** Date and time when the transaction last updated. UTC time zone. */
@JsonProperty("updated") @ExcludeMissing fun _updated() = updated
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Transaction = apply {
if (!validated) {
acquirerFee()
acquirerReferenceNumber()
accountToken()
amount()
amounts().validate()
authorizationAmount()
authorizationCode()
avs()?.validate()
cardToken()
cardholderAuthentication()?.validate()
created()
events()?.forEach { it.validate() }
merchant().validate()
merchantAmount()
merchantAuthorizationAmount()
merchantCurrency()
network()
networkRiskScore()
result()
pos().validate()
settledAmount()
status()
token()
tokenInfo()?.validate()
updated()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var acquirerFee: JsonField = JsonMissing.of()
private var acquirerReferenceNumber: JsonField = JsonMissing.of()
private var accountToken: JsonField = JsonMissing.of()
private var amount: JsonField = JsonMissing.of()
private var amounts: JsonField = JsonMissing.of()
private var authorizationAmount: JsonField = JsonMissing.of()
private var authorizationCode: JsonField = JsonMissing.of()
private var avs: JsonField = JsonMissing.of()
private var cardToken: JsonField = JsonMissing.of()
private var cardholderAuthentication: JsonField = JsonMissing.of()
private var created: JsonField = JsonMissing.of()
private var events: JsonField> = JsonMissing.of()
private var merchant: JsonField = JsonMissing.of()
private var merchantAmount: JsonField = JsonMissing.of()
private var merchantAuthorizationAmount: JsonField = JsonMissing.of()
private var merchantCurrency: JsonField = JsonMissing.of()
private var network: JsonField = JsonMissing.of()
private var networkRiskScore: JsonField = JsonMissing.of()
private var result: JsonField = JsonMissing.of()
private var pos: JsonField = JsonMissing.of()
private var settledAmount: JsonField = JsonMissing.of()
private var status: JsonField = JsonMissing.of()
private var token: JsonField = JsonMissing.of()
private var tokenInfo: JsonField = JsonMissing.of()
private var updated: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(transaction: Transaction) = apply {
this.acquirerFee = transaction.acquirerFee
this.acquirerReferenceNumber = transaction.acquirerReferenceNumber
this.accountToken = transaction.accountToken
this.amount = transaction.amount
this.amounts = transaction.amounts
this.authorizationAmount = transaction.authorizationAmount
this.authorizationCode = transaction.authorizationCode
this.avs = transaction.avs
this.cardToken = transaction.cardToken
this.cardholderAuthentication = transaction.cardholderAuthentication
this.created = transaction.created
this.events = transaction.events
this.merchant = transaction.merchant
this.merchantAmount = transaction.merchantAmount
this.merchantAuthorizationAmount = transaction.merchantAuthorizationAmount
this.merchantCurrency = transaction.merchantCurrency
this.network = transaction.network
this.networkRiskScore = transaction.networkRiskScore
this.result = transaction.result
this.pos = transaction.pos
this.settledAmount = transaction.settledAmount
this.status = transaction.status
this.token = transaction.token
this.tokenInfo = transaction.tokenInfo
this.updated = transaction.updated
additionalProperties(transaction.additionalProperties)
}
/**
* Fee assessed by the merchant and paid for by the cardholder in the smallest unit of the
* currency. Will be zero if no fee is assessed. Rebates may be transmitted as a negative
* value to indicate credited fees.
*/
fun acquirerFee(acquirerFee: Long) = acquirerFee(JsonField.of(acquirerFee))
/**
* Fee assessed by the merchant and paid for by the cardholder in the smallest unit of the
* currency. Will be zero if no fee is assessed. Rebates may be transmitted as a negative
* value to indicate credited fees.
*/
@JsonProperty("acquirer_fee")
@ExcludeMissing
fun acquirerFee(acquirerFee: JsonField) = apply { this.acquirerFee = acquirerFee }
/**
* Unique identifier assigned to a transaction by the acquirer that can be used in dispute
* and chargeback filing.
*/
fun acquirerReferenceNumber(acquirerReferenceNumber: String) =
acquirerReferenceNumber(JsonField.of(acquirerReferenceNumber))
/**
* Unique identifier assigned to a transaction by the acquirer that can be used in dispute
* and chargeback filing.
*/
@JsonProperty("acquirer_reference_number")
@ExcludeMissing
fun acquirerReferenceNumber(acquirerReferenceNumber: JsonField) = apply {
this.acquirerReferenceNumber = acquirerReferenceNumber
}
/** The token for the account associated with this transaction. */
fun accountToken(accountToken: String) = accountToken(JsonField.of(accountToken))
/** The token for the account associated with this transaction. */
@JsonProperty("account_token")
@ExcludeMissing
fun accountToken(accountToken: JsonField) = apply {
this.accountToken = accountToken
}
/**
* When the transaction is pending, this represents the authorization amount of the
* transaction in the anticipated settlement currency. Once the transaction has settled,
* this field represents the settled amount in the settlement currency.
*/
fun amount(amount: Long) = amount(JsonField.of(amount))
/**
* When the transaction is pending, this represents the authorization amount of the
* transaction in the anticipated settlement currency. Once the transaction has settled,
* this field represents the settled amount in the settlement currency.
*/
@JsonProperty("amount")
@ExcludeMissing
fun amount(amount: JsonField) = apply { this.amount = amount }
fun amounts(amounts: TransactionAmounts) = amounts(JsonField.of(amounts))
@JsonProperty("amounts")
@ExcludeMissing
fun amounts(amounts: JsonField) = apply { this.amounts = amounts }
/** The authorization amount of the transaction in the anticipated settlement currency. */
fun authorizationAmount(authorizationAmount: Long) =
authorizationAmount(JsonField.of(authorizationAmount))
/** The authorization amount of the transaction in the anticipated settlement currency. */
@JsonProperty("authorization_amount")
@ExcludeMissing
fun authorizationAmount(authorizationAmount: JsonField) = apply {
this.authorizationAmount = authorizationAmount
}
/**
* A fixed-width 6-digit numeric identifier that can be used to identify a transaction with
* networks.
*/
fun authorizationCode(authorizationCode: String) =
authorizationCode(JsonField.of(authorizationCode))
/**
* A fixed-width 6-digit numeric identifier that can be used to identify a transaction with
* networks.
*/
@JsonProperty("authorization_code")
@ExcludeMissing
fun authorizationCode(authorizationCode: JsonField) = apply {
this.authorizationCode = authorizationCode
}
fun avs(avs: Avs) = avs(JsonField.of(avs))
@JsonProperty("avs") @ExcludeMissing fun avs(avs: JsonField) = apply { this.avs = avs }
/** Token for the card used in this transaction. */
fun cardToken(cardToken: String) = cardToken(JsonField.of(cardToken))
/** Token for the card used in this transaction. */
@JsonProperty("card_token")
@ExcludeMissing
fun cardToken(cardToken: JsonField) = apply { this.cardToken = cardToken }
fun cardholderAuthentication(cardholderAuthentication: CardholderAuthentication) =
cardholderAuthentication(JsonField.of(cardholderAuthentication))
@JsonProperty("cardholder_authentication")
@ExcludeMissing
fun cardholderAuthentication(
cardholderAuthentication: JsonField
) = apply { this.cardholderAuthentication = cardholderAuthentication }
/** Date and time when the transaction first occurred. UTC time zone. */
fun created(created: OffsetDateTime) = created(JsonField.of(created))
/** Date and time when the transaction first occurred. UTC time zone. */
@JsonProperty("created")
@ExcludeMissing
fun created(created: JsonField) = apply { this.created = created }
fun events(events: List) = events(JsonField.of(events))
@JsonProperty("events")
@ExcludeMissing
fun events(events: JsonField>) = apply { this.events = events }
fun merchant(merchant: Merchant) = merchant(JsonField.of(merchant))
@JsonProperty("merchant")
@ExcludeMissing
fun merchant(merchant: JsonField) = apply { this.merchant = merchant }
/** Analogous to the 'amount', but in the merchant currency. */
fun merchantAmount(merchantAmount: Long) = merchantAmount(JsonField.of(merchantAmount))
/** Analogous to the 'amount', but in the merchant currency. */
@JsonProperty("merchant_amount")
@ExcludeMissing
fun merchantAmount(merchantAmount: JsonField) = apply {
this.merchantAmount = merchantAmount
}
/** Analogous to the 'authorization_amount', but in the merchant currency. */
fun merchantAuthorizationAmount(merchantAuthorizationAmount: Long) =
merchantAuthorizationAmount(JsonField.of(merchantAuthorizationAmount))
/** Analogous to the 'authorization_amount', but in the merchant currency. */
@JsonProperty("merchant_authorization_amount")
@ExcludeMissing
fun merchantAuthorizationAmount(merchantAuthorizationAmount: JsonField) = apply {
this.merchantAuthorizationAmount = merchantAuthorizationAmount
}
/** 3-digit alphabetic ISO 4217 code for the local currency of the transaction. */
fun merchantCurrency(merchantCurrency: String) =
merchantCurrency(JsonField.of(merchantCurrency))
/** 3-digit alphabetic ISO 4217 code for the local currency of the transaction. */
@JsonProperty("merchant_currency")
@ExcludeMissing
fun merchantCurrency(merchantCurrency: JsonField) = apply {
this.merchantCurrency = merchantCurrency
}
/**
* Card network of the authorization. Can be `INTERLINK`, `MAESTRO`, `MASTERCARD`, `VISA`,
* or `UNKNOWN`. Value is `UNKNOWN` when Lithic cannot determine the network code from the
* upstream provider.
*/
fun network(network: Network) = network(JsonField.of(network))
/**
* Card network of the authorization. Can be `INTERLINK`, `MAESTRO`, `MASTERCARD`, `VISA`,
* or `UNKNOWN`. Value is `UNKNOWN` when Lithic cannot determine the network code from the
* upstream provider.
*/
@JsonProperty("network")
@ExcludeMissing
fun network(network: JsonField) = apply { this.network = network }
/**
* Network-provided score assessing risk level associated with a given authorization. Scores
* are on a range of 0-999, with 0 representing the lowest risk and 999 representing the
* highest risk. For Visa transactions, where the raw score has a range of 0-99, Lithic will
* normalize the score by multiplying the raw score by 10x.
*/
fun networkRiskScore(networkRiskScore: Long) =
networkRiskScore(JsonField.of(networkRiskScore))
/**
* Network-provided score assessing risk level associated with a given authorization. Scores
* are on a range of 0-999, with 0 representing the lowest risk and 999 representing the
* highest risk. For Visa transactions, where the raw score has a range of 0-99, Lithic will
* normalize the score by multiplying the raw score by 10x.
*/
@JsonProperty("network_risk_score")
@ExcludeMissing
fun networkRiskScore(networkRiskScore: JsonField) = apply {
this.networkRiskScore = networkRiskScore
}
fun result(result: DeclineResult) = result(JsonField.of(result))
@JsonProperty("result")
@ExcludeMissing
fun result(result: JsonField) = apply { this.result = result }
fun pos(pos: Pos) = pos(JsonField.of(pos))
@JsonProperty("pos") @ExcludeMissing fun pos(pos: JsonField) = apply { this.pos = pos }
/** The settled amount of the transaction in the settlement currency. */
fun settledAmount(settledAmount: Long) = settledAmount(JsonField.of(settledAmount))
/** The settled amount of the transaction in the settlement currency. */
@JsonProperty("settled_amount")
@ExcludeMissing
fun settledAmount(settledAmount: JsonField) = apply {
this.settledAmount = settledAmount
}
/** Status of the transaction. */
fun status(status: Status) = status(JsonField.of(status))
/** Status of the transaction. */
@JsonProperty("status")
@ExcludeMissing
fun status(status: JsonField) = apply { this.status = status }
/** Globally unique identifier. */
fun token(token: String) = token(JsonField.of(token))
/** Globally unique identifier. */
@JsonProperty("token")
@ExcludeMissing
fun token(token: JsonField) = apply { this.token = token }
fun tokenInfo(tokenInfo: TokenInfo) = tokenInfo(JsonField.of(tokenInfo))
@JsonProperty("token_info")
@ExcludeMissing
fun tokenInfo(tokenInfo: JsonField) = apply { this.tokenInfo = tokenInfo }
/** Date and time when the transaction last updated. UTC time zone. */
fun updated(updated: OffsetDateTime) = updated(JsonField.of(updated))
/** Date and time when the transaction last updated. UTC time zone. */
@JsonProperty("updated")
@ExcludeMissing
fun updated(updated: JsonField) = apply { this.updated = updated }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Transaction =
Transaction(
acquirerFee,
acquirerReferenceNumber,
accountToken,
amount,
amounts,
authorizationAmount,
authorizationCode,
avs,
cardToken,
cardholderAuthentication,
created,
events.map { it.toImmutable() },
merchant,
merchantAmount,
merchantAuthorizationAmount,
merchantCurrency,
network,
networkRiskScore,
result,
pos,
settledAmount,
status,
token,
tokenInfo,
updated,
additionalProperties.toImmutable(),
)
}
@JsonDeserialize(builder = TransactionAmounts.Builder::class)
@NoAutoDetect
class TransactionAmounts
private constructor(
private val cardholder: JsonField,
private val hold: JsonField,
private val merchant: JsonField,
private val settlement: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
fun cardholder(): Cardholder = cardholder.getRequired("cardholder")
fun hold(): Hold = hold.getRequired("hold")
fun merchant(): Merchant = merchant.getRequired("merchant")
fun settlement(): Settlement = settlement.getRequired("settlement")
@JsonProperty("cardholder") @ExcludeMissing fun _cardholder() = cardholder
@JsonProperty("hold") @ExcludeMissing fun _hold() = hold
@JsonProperty("merchant") @ExcludeMissing fun _merchant() = merchant
@JsonProperty("settlement") @ExcludeMissing fun _settlement() = settlement
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): TransactionAmounts = apply {
if (!validated) {
cardholder().validate()
hold().validate()
merchant().validate()
settlement().validate()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var cardholder: JsonField = JsonMissing.of()
private var hold: JsonField = JsonMissing.of()
private var merchant: JsonField = JsonMissing.of()
private var settlement: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(transactionAmounts: TransactionAmounts) = apply {
this.cardholder = transactionAmounts.cardholder
this.hold = transactionAmounts.hold
this.merchant = transactionAmounts.merchant
this.settlement = transactionAmounts.settlement
additionalProperties(transactionAmounts.additionalProperties)
}
fun cardholder(cardholder: Cardholder) = cardholder(JsonField.of(cardholder))
@JsonProperty("cardholder")
@ExcludeMissing
fun cardholder(cardholder: JsonField) = apply {
this.cardholder = cardholder
}
fun hold(hold: Hold) = hold(JsonField.of(hold))
@JsonProperty("hold")
@ExcludeMissing
fun hold(hold: JsonField) = apply { this.hold = hold }
fun merchant(merchant: Merchant) = merchant(JsonField.of(merchant))
@JsonProperty("merchant")
@ExcludeMissing
fun merchant(merchant: JsonField) = apply { this.merchant = merchant }
fun settlement(settlement: Settlement) = settlement(JsonField.of(settlement))
@JsonProperty("settlement")
@ExcludeMissing
fun settlement(settlement: JsonField) = apply {
this.settlement = settlement
}
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): TransactionAmounts =
TransactionAmounts(
cardholder,
hold,
merchant,
settlement,
additionalProperties.toImmutable(),
)
}
@JsonDeserialize(builder = Cardholder.Builder::class)
@NoAutoDetect
class Cardholder
private constructor(
private val amount: JsonField,
private val conversionRate: JsonField,
private val currency: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** The aggregate settled amount in the cardholder billing currency. */
fun amount(): Long = amount.getRequired("amount")
/**
* The conversion rate used to convert the merchant amount to the cardholder billing
* amount.
*/
fun conversionRate(): String = conversionRate.getRequired("conversion_rate")
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(): Currency = currency.getRequired("currency")
/** The aggregate settled amount in the cardholder billing currency. */
@JsonProperty("amount") @ExcludeMissing fun _amount() = amount
/**
* The conversion rate used to convert the merchant amount to the cardholder billing
* amount.
*/
@JsonProperty("conversion_rate") @ExcludeMissing fun _conversionRate() = conversionRate
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency") @ExcludeMissing fun _currency() = currency
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Cardholder = apply {
if (!validated) {
amount()
conversionRate()
currency()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var amount: JsonField = JsonMissing.of()
private var conversionRate: JsonField = JsonMissing.of()
private var currency: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(cardholder: Cardholder) = apply {
this.amount = cardholder.amount
this.conversionRate = cardholder.conversionRate
this.currency = cardholder.currency
additionalProperties(cardholder.additionalProperties)
}
/** The aggregate settled amount in the cardholder billing currency. */
fun amount(amount: Long) = amount(JsonField.of(amount))
/** The aggregate settled amount in the cardholder billing currency. */
@JsonProperty("amount")
@ExcludeMissing
fun amount(amount: JsonField) = apply { this.amount = amount }
/**
* The conversion rate used to convert the merchant amount to the cardholder billing
* amount.
*/
fun conversionRate(conversionRate: String) =
conversionRate(JsonField.of(conversionRate))
/**
* The conversion rate used to convert the merchant amount to the cardholder billing
* amount.
*/
@JsonProperty("conversion_rate")
@ExcludeMissing
fun conversionRate(conversionRate: JsonField) = apply {
this.conversionRate = conversionRate
}
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(currency: Currency) = currency(JsonField.of(currency))
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency")
@ExcludeMissing
fun currency(currency: JsonField) = apply { this.currency = currency }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) =
apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Cardholder =
Cardholder(
amount,
conversionRate,
currency,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Cardholder && this.amount == other.amount && this.conversionRate == other.conversionRate && this.currency == other.currency && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(amount, conversionRate, currency, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"Cardholder{amount=$amount, conversionRate=$conversionRate, currency=$currency, additionalProperties=$additionalProperties}"
}
@JsonDeserialize(builder = Hold.Builder::class)
@NoAutoDetect
class Hold
private constructor(
private val amount: JsonField,
private val currency: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/**
* The aggregate authorization amount of the transaction in the anticipated settlement
* currency.
*/
fun amount(): Long = amount.getRequired("amount")
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(): Currency = currency.getRequired("currency")
/**
* The aggregate authorization amount of the transaction in the anticipated settlement
* currency.
*/
@JsonProperty("amount") @ExcludeMissing fun _amount() = amount
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency") @ExcludeMissing fun _currency() = currency
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Hold = apply {
if (!validated) {
amount()
currency()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var amount: JsonField = JsonMissing.of()
private var currency: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(hold: Hold) = apply {
this.amount = hold.amount
this.currency = hold.currency
additionalProperties(hold.additionalProperties)
}
/**
* The aggregate authorization amount of the transaction in the anticipated
* settlement currency.
*/
fun amount(amount: Long) = amount(JsonField.of(amount))
/**
* The aggregate authorization amount of the transaction in the anticipated
* settlement currency.
*/
@JsonProperty("amount")
@ExcludeMissing
fun amount(amount: JsonField) = apply { this.amount = amount }
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(currency: Currency) = currency(JsonField.of(currency))
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency")
@ExcludeMissing
fun currency(currency: JsonField) = apply { this.currency = currency }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) =
apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Hold =
Hold(
amount,
currency,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Hold && this.amount == other.amount && this.currency == other.currency && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(amount, currency, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"Hold{amount=$amount, currency=$currency, additionalProperties=$additionalProperties}"
}
@JsonDeserialize(builder = Merchant.Builder::class)
@NoAutoDetect
class Merchant
private constructor(
private val amount: JsonField,
private val currency: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** The aggregate settled amount in the merchant currency. */
fun amount(): Long = amount.getRequired("amount")
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(): Currency = currency.getRequired("currency")
/** The aggregate settled amount in the merchant currency. */
@JsonProperty("amount") @ExcludeMissing fun _amount() = amount
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency") @ExcludeMissing fun _currency() = currency
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Merchant = apply {
if (!validated) {
amount()
currency()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var amount: JsonField = JsonMissing.of()
private var currency: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(merchant: Merchant) = apply {
this.amount = merchant.amount
this.currency = merchant.currency
additionalProperties(merchant.additionalProperties)
}
/** The aggregate settled amount in the merchant currency. */
fun amount(amount: Long) = amount(JsonField.of(amount))
/** The aggregate settled amount in the merchant currency. */
@JsonProperty("amount")
@ExcludeMissing
fun amount(amount: JsonField) = apply { this.amount = amount }
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(currency: Currency) = currency(JsonField.of(currency))
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency")
@ExcludeMissing
fun currency(currency: JsonField) = apply { this.currency = currency }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) =
apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Merchant =
Merchant(
amount,
currency,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Merchant && this.amount == other.amount && this.currency == other.currency && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(amount, currency, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"Merchant{amount=$amount, currency=$currency, additionalProperties=$additionalProperties}"
}
@JsonDeserialize(builder = Settlement.Builder::class)
@NoAutoDetect
class Settlement
private constructor(
private val amount: JsonField,
private val currency: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** The aggregate settled amount in the settlement currency. */
fun amount(): Long = amount.getRequired("amount")
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(): Currency = currency.getRequired("currency")
/** The aggregate settled amount in the settlement currency. */
@JsonProperty("amount") @ExcludeMissing fun _amount() = amount
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency") @ExcludeMissing fun _currency() = currency
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Settlement = apply {
if (!validated) {
amount()
currency()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var amount: JsonField = JsonMissing.of()
private var currency: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(settlement: Settlement) = apply {
this.amount = settlement.amount
this.currency = settlement.currency
additionalProperties(settlement.additionalProperties)
}
/** The aggregate settled amount in the settlement currency. */
fun amount(amount: Long) = amount(JsonField.of(amount))
/** The aggregate settled amount in the settlement currency. */
@JsonProperty("amount")
@ExcludeMissing
fun amount(amount: JsonField) = apply { this.amount = amount }
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
fun currency(currency: Currency) = currency(JsonField.of(currency))
/**
* ISO 4217 currency. Its enumerants are ISO 4217 currencies except for some special
* currencies like `XXX`. Enumerants names are lowercase currency code e.g. `EUR`,
* `USD`.
*/
@JsonProperty("currency")
@ExcludeMissing
fun currency(currency: JsonField) = apply { this.currency = currency }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) =
apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Settlement =
Settlement(
amount,
currency,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Settlement && this.amount == other.amount && this.currency == other.currency && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(amount, currency, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"Settlement{amount=$amount, currency=$currency, additionalProperties=$additionalProperties}"
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is TransactionAmounts && this.cardholder == other.cardholder && this.hold == other.hold && this.merchant == other.merchant && this.settlement == other.settlement && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(cardholder, hold, merchant, settlement, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"TransactionAmounts{cardholder=$cardholder, hold=$hold, merchant=$merchant, settlement=$settlement, additionalProperties=$additionalProperties}"
}
@JsonDeserialize(builder = Avs.Builder::class)
@NoAutoDetect
class Avs
private constructor(
private val address: JsonField,
private val zipcode: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** Cardholder address */
fun address(): String = address.getRequired("address")
/** Cardholder ZIP code */
fun zipcode(): String = zipcode.getRequired("zipcode")
/** Cardholder address */
@JsonProperty("address") @ExcludeMissing fun _address() = address
/** Cardholder ZIP code */
@JsonProperty("zipcode") @ExcludeMissing fun _zipcode() = zipcode
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Avs = apply {
if (!validated) {
address()
zipcode()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var address: JsonField = JsonMissing.of()
private var zipcode: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(avs: Avs) = apply {
this.address = avs.address
this.zipcode = avs.zipcode
additionalProperties(avs.additionalProperties)
}
/** Cardholder address */
fun address(address: String) = address(JsonField.of(address))
/** Cardholder address */
@JsonProperty("address")
@ExcludeMissing
fun address(address: JsonField) = apply { this.address = address }
/** Cardholder ZIP code */
fun zipcode(zipcode: String) = zipcode(JsonField.of(zipcode))
/** Cardholder ZIP code */
@JsonProperty("zipcode")
@ExcludeMissing
fun zipcode(zipcode: JsonField) = apply { this.zipcode = zipcode }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Avs =
Avs(
address,
zipcode,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Avs && this.address == other.address && this.zipcode == other.zipcode && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(address, zipcode, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"Avs{address=$address, zipcode=$zipcode, additionalProperties=$additionalProperties}"
}
@JsonDeserialize(builder = CardholderAuthentication.Builder::class)
@NoAutoDetect
class CardholderAuthentication
private constructor(
private val _3dsVersion: JsonField,
private val acquirerExemption: JsonField,
private val authenticationResult: JsonField,
private val decisionMadeBy: JsonField,
private val liabilityShift: JsonField,
private val threeDSAuthenticationToken: JsonField,
private val verificationAttempted: JsonField,
private val verificationResult: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** The 3DS version used for the authentication */
fun _3dsVersion(): String? = _3dsVersion.getNullable("3ds_version")
/** Whether an acquirer exemption applied to the transaction. */
fun acquirerExemption(): AcquirerExemption =
acquirerExemption.getRequired("acquirer_exemption")
/** Indicates what the outcome of the 3DS authentication process is. */
fun authenticationResult(): AuthenticationResult =
authenticationResult.getRequired("authentication_result")
/** Indicates which party made the 3DS authentication decision. */
fun decisionMadeBy(): DecisionMadeBy = decisionMadeBy.getRequired("decision_made_by")
/**
* Indicates whether chargeback liability shift applies to the transaction. Possible enum
* values:
*
* * `3DS_AUTHENTICATED`: The transaction was fully authenticated through a 3-D Secure flow, chargeback liability shift applies.
* * `ACQUIRER_EXEMPTION`: The acquirer utilised an exemption to bypass Strong Customer Authentication (`transStatus = N`, or `transStatus = I`). Liability remains with the acquirer and in this case the `acquirer_exemption` field is expected to be not `NONE`.
* * `NONE`: Chargeback liability shift has not shifted to the issuer, i.e. the merchant is liable.
* - `TOKEN_AUTHENTICATED`: The transaction was a tokenized payment with validated
* cryptography, possibly recurring. Chargeback liability shift to the issuer applies.
*/
fun liabilityShift(): LiabilityShift = liabilityShift.getRequired("liability_shift")
/**
* Unique identifier you can use to match a given 3DS authentication (available via the
* three_ds_authentication.created event webhook) and the transaction. Note that in cases
* where liability shift does not occur, this token is matched to the transaction on a
* best-effort basis.
*/
fun threeDSAuthenticationToken(): String? =
threeDSAuthenticationToken.getNullable("three_ds_authentication_token")
/**
* Indicates whether a 3DS challenge flow was used, and if so, what the verification method
* was. (deprecated, use `authentication_result`)
*/
fun verificationAttempted(): VerificationAttempted =
verificationAttempted.getRequired("verification_attempted")
/**
* Indicates whether a transaction is considered 3DS authenticated. (deprecated, use
* `authentication_result`)
*/
fun verificationResult(): VerificationResult =
verificationResult.getRequired("verification_result")
/** The 3DS version used for the authentication */
@JsonProperty("3ds_version") @ExcludeMissing fun __3dsVersion() = _3dsVersion
/** Whether an acquirer exemption applied to the transaction. */
@JsonProperty("acquirer_exemption")
@ExcludeMissing
fun _acquirerExemption() = acquirerExemption
/** Indicates what the outcome of the 3DS authentication process is. */
@JsonProperty("authentication_result")
@ExcludeMissing
fun _authenticationResult() = authenticationResult
/** Indicates which party made the 3DS authentication decision. */
@JsonProperty("decision_made_by") @ExcludeMissing fun _decisionMadeBy() = decisionMadeBy
/**
* Indicates whether chargeback liability shift applies to the transaction. Possible enum
* values:
*
* * `3DS_AUTHENTICATED`: The transaction was fully authenticated through a 3-D Secure flow, chargeback liability shift applies.
* * `ACQUIRER_EXEMPTION`: The acquirer utilised an exemption to bypass Strong Customer Authentication (`transStatus = N`, or `transStatus = I`). Liability remains with the acquirer and in this case the `acquirer_exemption` field is expected to be not `NONE`.
* * `NONE`: Chargeback liability shift has not shifted to the issuer, i.e. the merchant is liable.
* - `TOKEN_AUTHENTICATED`: The transaction was a tokenized payment with validated
* cryptography, possibly recurring. Chargeback liability shift to the issuer applies.
*/
@JsonProperty("liability_shift") @ExcludeMissing fun _liabilityShift() = liabilityShift
/**
* Unique identifier you can use to match a given 3DS authentication (available via the
* three_ds_authentication.created event webhook) and the transaction. Note that in cases
* where liability shift does not occur, this token is matched to the transaction on a
* best-effort basis.
*/
@JsonProperty("three_ds_authentication_token")
@ExcludeMissing
fun _threeDSAuthenticationToken() = threeDSAuthenticationToken
/**
* Indicates whether a 3DS challenge flow was used, and if so, what the verification method
* was. (deprecated, use `authentication_result`)
*/
@JsonProperty("verification_attempted")
@ExcludeMissing
fun _verificationAttempted() = verificationAttempted
/**
* Indicates whether a transaction is considered 3DS authenticated. (deprecated, use
* `authentication_result`)
*/
@JsonProperty("verification_result")
@ExcludeMissing
fun _verificationResult() = verificationResult
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): CardholderAuthentication = apply {
if (!validated) {
_3dsVersion()
acquirerExemption()
authenticationResult()
decisionMadeBy()
liabilityShift()
threeDSAuthenticationToken()
verificationAttempted()
verificationResult()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var _3dsVersion: JsonField = JsonMissing.of()
private var acquirerExemption: JsonField = JsonMissing.of()
private var authenticationResult: JsonField = JsonMissing.of()
private var decisionMadeBy: JsonField = JsonMissing.of()
private var liabilityShift: JsonField = JsonMissing.of()
private var threeDSAuthenticationToken: JsonField = JsonMissing.of()
private var verificationAttempted: JsonField = JsonMissing.of()
private var verificationResult: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(cardholderAuthentication: CardholderAuthentication) = apply {
this._3dsVersion = cardholderAuthentication._3dsVersion
this.acquirerExemption = cardholderAuthentication.acquirerExemption
this.authenticationResult = cardholderAuthentication.authenticationResult
this.decisionMadeBy = cardholderAuthentication.decisionMadeBy
this.liabilityShift = cardholderAuthentication.liabilityShift
this.threeDSAuthenticationToken =
cardholderAuthentication.threeDSAuthenticationToken
this.verificationAttempted = cardholderAuthentication.verificationAttempted
this.verificationResult = cardholderAuthentication.verificationResult
additionalProperties(cardholderAuthentication.additionalProperties)
}
/** The 3DS version used for the authentication */
fun _3dsVersion(_3dsVersion: String) = _3dsVersion(JsonField.of(_3dsVersion))
/** The 3DS version used for the authentication */
@JsonProperty("3ds_version")
@ExcludeMissing
fun _3dsVersion(_3dsVersion: JsonField) = apply {
this._3dsVersion = _3dsVersion
}
/** Whether an acquirer exemption applied to the transaction. */
fun acquirerExemption(acquirerExemption: AcquirerExemption) =
acquirerExemption(JsonField.of(acquirerExemption))
/** Whether an acquirer exemption applied to the transaction. */
@JsonProperty("acquirer_exemption")
@ExcludeMissing
fun acquirerExemption(acquirerExemption: JsonField) = apply {
this.acquirerExemption = acquirerExemption
}
/** Indicates what the outcome of the 3DS authentication process is. */
fun authenticationResult(authenticationResult: AuthenticationResult) =
authenticationResult(JsonField.of(authenticationResult))
/** Indicates what the outcome of the 3DS authentication process is. */
@JsonProperty("authentication_result")
@ExcludeMissing
fun authenticationResult(authenticationResult: JsonField) =
apply {
this.authenticationResult = authenticationResult
}
/** Indicates which party made the 3DS authentication decision. */
fun decisionMadeBy(decisionMadeBy: DecisionMadeBy) =
decisionMadeBy(JsonField.of(decisionMadeBy))
/** Indicates which party made the 3DS authentication decision. */
@JsonProperty("decision_made_by")
@ExcludeMissing
fun decisionMadeBy(decisionMadeBy: JsonField) = apply {
this.decisionMadeBy = decisionMadeBy
}
/**
* Indicates whether chargeback liability shift applies to the transaction. Possible
* enum values:
*
* * `3DS_AUTHENTICATED`: The transaction was fully authenticated through a 3-D Secure flow, chargeback liability shift applies.
* * `ACQUIRER_EXEMPTION`: The acquirer utilised an exemption to bypass Strong Customer Authentication (`transStatus = N`, or `transStatus = I`). Liability remains with the acquirer and in this case the `acquirer_exemption` field is expected to be not `NONE`.
* * `NONE`: Chargeback liability shift has not shifted to the issuer, i.e. the merchant is liable.
* - `TOKEN_AUTHENTICATED`: The transaction was a tokenized payment with validated
* cryptography, possibly recurring. Chargeback liability shift to the issuer applies.
*/
fun liabilityShift(liabilityShift: LiabilityShift) =
liabilityShift(JsonField.of(liabilityShift))
/**
* Indicates whether chargeback liability shift applies to the transaction. Possible
* enum values:
*
* * `3DS_AUTHENTICATED`: The transaction was fully authenticated through a 3-D Secure flow, chargeback liability shift applies.
* * `ACQUIRER_EXEMPTION`: The acquirer utilised an exemption to bypass Strong Customer Authentication (`transStatus = N`, or `transStatus = I`). Liability remains with the acquirer and in this case the `acquirer_exemption` field is expected to be not `NONE`.
* * `NONE`: Chargeback liability shift has not shifted to the issuer, i.e. the merchant is liable.
* - `TOKEN_AUTHENTICATED`: The transaction was a tokenized payment with validated
* cryptography, possibly recurring. Chargeback liability shift to the issuer applies.
*/
@JsonProperty("liability_shift")
@ExcludeMissing
fun liabilityShift(liabilityShift: JsonField) = apply {
this.liabilityShift = liabilityShift
}
/**
* Unique identifier you can use to match a given 3DS authentication (available via the
* three_ds_authentication.created event webhook) and the transaction. Note that in
* cases where liability shift does not occur, this token is matched to the transaction
* on a best-effort basis.
*/
fun threeDSAuthenticationToken(threeDSAuthenticationToken: String) =
threeDSAuthenticationToken(JsonField.of(threeDSAuthenticationToken))
/**
* Unique identifier you can use to match a given 3DS authentication (available via the
* three_ds_authentication.created event webhook) and the transaction. Note that in
* cases where liability shift does not occur, this token is matched to the transaction
* on a best-effort basis.
*/
@JsonProperty("three_ds_authentication_token")
@ExcludeMissing
fun threeDSAuthenticationToken(threeDSAuthenticationToken: JsonField) = apply {
this.threeDSAuthenticationToken = threeDSAuthenticationToken
}
/**
* Indicates whether a 3DS challenge flow was used, and if so, what the verification
* method was. (deprecated, use `authentication_result`)
*/
fun verificationAttempted(verificationAttempted: VerificationAttempted) =
verificationAttempted(JsonField.of(verificationAttempted))
/**
* Indicates whether a 3DS challenge flow was used, and if so, what the verification
* method was. (deprecated, use `authentication_result`)
*/
@JsonProperty("verification_attempted")
@ExcludeMissing
fun verificationAttempted(verificationAttempted: JsonField) =
apply {
this.verificationAttempted = verificationAttempted
}
/**
* Indicates whether a transaction is considered 3DS authenticated. (deprecated, use
* `authentication_result`)
*/
fun verificationResult(verificationResult: VerificationResult) =
verificationResult(JsonField.of(verificationResult))
/**
* Indicates whether a transaction is considered 3DS authenticated. (deprecated, use
* `authentication_result`)
*/
@JsonProperty("verification_result")
@ExcludeMissing
fun verificationResult(verificationResult: JsonField) = apply {
this.verificationResult = verificationResult
}
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): CardholderAuthentication =
CardholderAuthentication(
_3dsVersion,
acquirerExemption,
authenticationResult,
decisionMadeBy,
liabilityShift,
threeDSAuthenticationToken,
verificationAttempted,
verificationResult,
additionalProperties.toImmutable(),
)
}
class AcquirerExemption
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is AcquirerExemption && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val AUTHENTICATION_OUTAGE_EXCEPTION =
AcquirerExemption(JsonField.of("AUTHENTICATION_OUTAGE_EXCEPTION"))
val LOW_VALUE = AcquirerExemption(JsonField.of("LOW_VALUE"))
val MERCHANT_INITIATED_TRANSACTION =
AcquirerExemption(JsonField.of("MERCHANT_INITIATED_TRANSACTION"))
val NONE = AcquirerExemption(JsonField.of("NONE"))
val RECURRING_PAYMENT = AcquirerExemption(JsonField.of("RECURRING_PAYMENT"))
val SECURE_CORPORATE_PAYMENT =
AcquirerExemption(JsonField.of("SECURE_CORPORATE_PAYMENT"))
val STRONG_CUSTOMER_AUTHENTICATION_DELEGATION =
AcquirerExemption(JsonField.of("STRONG_CUSTOMER_AUTHENTICATION_DELEGATION"))
val TRANSACTION_RISK_ANALYSIS =
AcquirerExemption(JsonField.of("TRANSACTION_RISK_ANALYSIS"))
fun of(value: String) = AcquirerExemption(JsonField.of(value))
}
enum class Known {
AUTHENTICATION_OUTAGE_EXCEPTION,
LOW_VALUE,
MERCHANT_INITIATED_TRANSACTION,
NONE,
RECURRING_PAYMENT,
SECURE_CORPORATE_PAYMENT,
STRONG_CUSTOMER_AUTHENTICATION_DELEGATION,
TRANSACTION_RISK_ANALYSIS,
}
enum class Value {
AUTHENTICATION_OUTAGE_EXCEPTION,
LOW_VALUE,
MERCHANT_INITIATED_TRANSACTION,
NONE,
RECURRING_PAYMENT,
SECURE_CORPORATE_PAYMENT,
STRONG_CUSTOMER_AUTHENTICATION_DELEGATION,
TRANSACTION_RISK_ANALYSIS,
_UNKNOWN,
}
fun value(): Value =
when (this) {
AUTHENTICATION_OUTAGE_EXCEPTION -> Value.AUTHENTICATION_OUTAGE_EXCEPTION
LOW_VALUE -> Value.LOW_VALUE
MERCHANT_INITIATED_TRANSACTION -> Value.MERCHANT_INITIATED_TRANSACTION
NONE -> Value.NONE
RECURRING_PAYMENT -> Value.RECURRING_PAYMENT
SECURE_CORPORATE_PAYMENT -> Value.SECURE_CORPORATE_PAYMENT
STRONG_CUSTOMER_AUTHENTICATION_DELEGATION ->
Value.STRONG_CUSTOMER_AUTHENTICATION_DELEGATION
TRANSACTION_RISK_ANALYSIS -> Value.TRANSACTION_RISK_ANALYSIS
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
AUTHENTICATION_OUTAGE_EXCEPTION -> Known.AUTHENTICATION_OUTAGE_EXCEPTION
LOW_VALUE -> Known.LOW_VALUE
MERCHANT_INITIATED_TRANSACTION -> Known.MERCHANT_INITIATED_TRANSACTION
NONE -> Known.NONE
RECURRING_PAYMENT -> Known.RECURRING_PAYMENT
SECURE_CORPORATE_PAYMENT -> Known.SECURE_CORPORATE_PAYMENT
STRONG_CUSTOMER_AUTHENTICATION_DELEGATION ->
Known.STRONG_CUSTOMER_AUTHENTICATION_DELEGATION
TRANSACTION_RISK_ANALYSIS -> Known.TRANSACTION_RISK_ANALYSIS
else -> throw LithicInvalidDataException("Unknown AcquirerExemption: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
class AuthenticationResult
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is AuthenticationResult && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val ATTEMPTS = AuthenticationResult(JsonField.of("ATTEMPTS"))
val DECLINE = AuthenticationResult(JsonField.of("DECLINE"))
val NONE = AuthenticationResult(JsonField.of("NONE"))
val SUCCESS = AuthenticationResult(JsonField.of("SUCCESS"))
fun of(value: String) = AuthenticationResult(JsonField.of(value))
}
enum class Known {
ATTEMPTS,
DECLINE,
NONE,
SUCCESS,
}
enum class Value {
ATTEMPTS,
DECLINE,
NONE,
SUCCESS,
_UNKNOWN,
}
fun value(): Value =
when (this) {
ATTEMPTS -> Value.ATTEMPTS
DECLINE -> Value.DECLINE
NONE -> Value.NONE
SUCCESS -> Value.SUCCESS
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
ATTEMPTS -> Known.ATTEMPTS
DECLINE -> Known.DECLINE
NONE -> Known.NONE
SUCCESS -> Known.SUCCESS
else -> throw LithicInvalidDataException("Unknown AuthenticationResult: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
class DecisionMadeBy
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is DecisionMadeBy && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val CUSTOMER_ENDPOINT = DecisionMadeBy(JsonField.of("CUSTOMER_ENDPOINT"))
val LITHIC_DEFAULT = DecisionMadeBy(JsonField.of("LITHIC_DEFAULT"))
val LITHIC_RULES = DecisionMadeBy(JsonField.of("LITHIC_RULES"))
val NETWORK = DecisionMadeBy(JsonField.of("NETWORK"))
val UNKNOWN = DecisionMadeBy(JsonField.of("UNKNOWN"))
fun of(value: String) = DecisionMadeBy(JsonField.of(value))
}
enum class Known {
CUSTOMER_ENDPOINT,
LITHIC_DEFAULT,
LITHIC_RULES,
NETWORK,
UNKNOWN,
}
enum class Value {
CUSTOMER_ENDPOINT,
LITHIC_DEFAULT,
LITHIC_RULES,
NETWORK,
UNKNOWN,
_UNKNOWN,
}
fun value(): Value =
when (this) {
CUSTOMER_ENDPOINT -> Value.CUSTOMER_ENDPOINT
LITHIC_DEFAULT -> Value.LITHIC_DEFAULT
LITHIC_RULES -> Value.LITHIC_RULES
NETWORK -> Value.NETWORK
UNKNOWN -> Value.UNKNOWN
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
CUSTOMER_ENDPOINT -> Known.CUSTOMER_ENDPOINT
LITHIC_DEFAULT -> Known.LITHIC_DEFAULT
LITHIC_RULES -> Known.LITHIC_RULES
NETWORK -> Known.NETWORK
UNKNOWN -> Known.UNKNOWN
else -> throw LithicInvalidDataException("Unknown DecisionMadeBy: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
class LiabilityShift
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is LiabilityShift && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val _3DS_AUTHENTICATED = LiabilityShift(JsonField.of("3DS_AUTHENTICATED"))
val ACQUIRER_EXEMPTION = LiabilityShift(JsonField.of("ACQUIRER_EXEMPTION"))
val NONE = LiabilityShift(JsonField.of("NONE"))
val TOKEN_AUTHENTICATED = LiabilityShift(JsonField.of("TOKEN_AUTHENTICATED"))
fun of(value: String) = LiabilityShift(JsonField.of(value))
}
enum class Known {
_3DS_AUTHENTICATED,
ACQUIRER_EXEMPTION,
NONE,
TOKEN_AUTHENTICATED,
}
enum class Value {
_3DS_AUTHENTICATED,
ACQUIRER_EXEMPTION,
NONE,
TOKEN_AUTHENTICATED,
_UNKNOWN,
}
fun value(): Value =
when (this) {
_3DS_AUTHENTICATED -> Value._3DS_AUTHENTICATED
ACQUIRER_EXEMPTION -> Value.ACQUIRER_EXEMPTION
NONE -> Value.NONE
TOKEN_AUTHENTICATED -> Value.TOKEN_AUTHENTICATED
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
_3DS_AUTHENTICATED -> Known._3DS_AUTHENTICATED
ACQUIRER_EXEMPTION -> Known.ACQUIRER_EXEMPTION
NONE -> Known.NONE
TOKEN_AUTHENTICATED -> Known.TOKEN_AUTHENTICATED
else -> throw LithicInvalidDataException("Unknown LiabilityShift: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
class VerificationAttempted
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is VerificationAttempted && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val NONE = VerificationAttempted(JsonField.of("NONE"))
val OTHER = VerificationAttempted(JsonField.of("OTHER"))
fun of(value: String) = VerificationAttempted(JsonField.of(value))
}
enum class Known {
NONE,
OTHER,
}
enum class Value {
NONE,
OTHER,
_UNKNOWN,
}
fun value(): Value =
when (this) {
NONE -> Value.NONE
OTHER -> Value.OTHER
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
NONE -> Known.NONE
OTHER -> Known.OTHER
else ->
throw LithicInvalidDataException("Unknown VerificationAttempted: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
class VerificationResult
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is VerificationResult && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val CANCELLED = VerificationResult(JsonField.of("CANCELLED"))
val FAILED = VerificationResult(JsonField.of("FAILED"))
val FRICTIONLESS = VerificationResult(JsonField.of("FRICTIONLESS"))
val NOT_ATTEMPTED = VerificationResult(JsonField.of("NOT_ATTEMPTED"))
val REJECTED = VerificationResult(JsonField.of("REJECTED"))
val SUCCESS = VerificationResult(JsonField.of("SUCCESS"))
fun of(value: String) = VerificationResult(JsonField.of(value))
}
enum class Known {
CANCELLED,
FAILED,
FRICTIONLESS,
NOT_ATTEMPTED,
REJECTED,
SUCCESS,
}
enum class Value {
CANCELLED,
FAILED,
FRICTIONLESS,
NOT_ATTEMPTED,
REJECTED,
SUCCESS,
_UNKNOWN,
}
fun value(): Value =
when (this) {
CANCELLED -> Value.CANCELLED
FAILED -> Value.FAILED
FRICTIONLESS -> Value.FRICTIONLESS
NOT_ATTEMPTED -> Value.NOT_ATTEMPTED
REJECTED -> Value.REJECTED
SUCCESS -> Value.SUCCESS
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
CANCELLED -> Known.CANCELLED
FAILED -> Known.FAILED
FRICTIONLESS -> Known.FRICTIONLESS
NOT_ATTEMPTED -> Known.NOT_ATTEMPTED
REJECTED -> Known.REJECTED
SUCCESS -> Known.SUCCESS
else -> throw LithicInvalidDataException("Unknown VerificationResult: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is CardholderAuthentication && this._3dsVersion == other._3dsVersion && this.acquirerExemption == other.acquirerExemption && this.authenticationResult == other.authenticationResult && this.decisionMadeBy == other.decisionMadeBy && this.liabilityShift == other.liabilityShift && this.threeDSAuthenticationToken == other.threeDSAuthenticationToken && this.verificationAttempted == other.verificationAttempted && this.verificationResult == other.verificationResult && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(_3dsVersion, acquirerExemption, authenticationResult, decisionMadeBy, liabilityShift, threeDSAuthenticationToken, verificationAttempted, verificationResult, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"CardholderAuthentication{_3dsVersion=$_3dsVersion, acquirerExemption=$acquirerExemption, authenticationResult=$authenticationResult, decisionMadeBy=$decisionMadeBy, liabilityShift=$liabilityShift, threeDSAuthenticationToken=$threeDSAuthenticationToken, verificationAttempted=$verificationAttempted, verificationResult=$verificationResult, additionalProperties=$additionalProperties}"
}
@JsonDeserialize(builder = Merchant.Builder::class)
@NoAutoDetect
class Merchant
private constructor(
private val acceptorId: JsonField,
private val acquiringInstitutionId: JsonField,
private val city: JsonField,
private val country: JsonField,
private val descriptor: JsonField,
private val mcc: JsonField,
private val state: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** Unique alphanumeric identifier for the payment card acceptor (merchant). */
fun acceptorId(): String = acceptorId.getRequired("acceptor_id")
/** Unique numeric identifier of the acquiring institution. */
fun acquiringInstitutionId(): String =
acquiringInstitutionId.getRequired("acquiring_institution_id")
/**
* City of card acceptor. Note that in many cases, particularly in card-not-present
* transactions, merchants may send through a phone number or URL in this field.
*/
fun city(): String = city.getRequired("city")
/**
* Country or entity of card acceptor. Possible values are: (1) all ISO 3166-1 alpha-3
* country codes, (2) QZZ for Kosovo, and (3) ANT for Netherlands Antilles.
*/
fun country(): String = country.getRequired("country")
/** Short description of card acceptor. */
fun descriptor(): String = descriptor.getRequired("descriptor")
/**
* Merchant category code (MCC). A four-digit number listed in ISO 18245. An MCC is used to
* classify a business by the types of goods or services it provides.
*/
fun mcc(): String = mcc.getRequired("mcc")
/** Geographic state of card acceptor. */
fun state(): String = state.getRequired("state")
/** Unique alphanumeric identifier for the payment card acceptor (merchant). */
@JsonProperty("acceptor_id") @ExcludeMissing fun _acceptorId() = acceptorId
/** Unique numeric identifier of the acquiring institution. */
@JsonProperty("acquiring_institution_id")
@ExcludeMissing
fun _acquiringInstitutionId() = acquiringInstitutionId
/**
* City of card acceptor. Note that in many cases, particularly in card-not-present
* transactions, merchants may send through a phone number or URL in this field.
*/
@JsonProperty("city") @ExcludeMissing fun _city() = city
/**
* Country or entity of card acceptor. Possible values are: (1) all ISO 3166-1 alpha-3
* country codes, (2) QZZ for Kosovo, and (3) ANT for Netherlands Antilles.
*/
@JsonProperty("country") @ExcludeMissing fun _country() = country
/** Short description of card acceptor. */
@JsonProperty("descriptor") @ExcludeMissing fun _descriptor() = descriptor
/**
* Merchant category code (MCC). A four-digit number listed in ISO 18245. An MCC is used to
* classify a business by the types of goods or services it provides.
*/
@JsonProperty("mcc") @ExcludeMissing fun _mcc() = mcc
/** Geographic state of card acceptor. */
@JsonProperty("state") @ExcludeMissing fun _state() = state
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Merchant = apply {
if (!validated) {
acceptorId()
acquiringInstitutionId()
city()
country()
descriptor()
mcc()
state()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var acceptorId: JsonField = JsonMissing.of()
private var acquiringInstitutionId: JsonField = JsonMissing.of()
private var city: JsonField = JsonMissing.of()
private var country: JsonField = JsonMissing.of()
private var descriptor: JsonField = JsonMissing.of()
private var mcc: JsonField = JsonMissing.of()
private var state: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(merchant: Merchant) = apply {
this.acceptorId = merchant.acceptorId
this.acquiringInstitutionId = merchant.acquiringInstitutionId
this.city = merchant.city
this.country = merchant.country
this.descriptor = merchant.descriptor
this.mcc = merchant.mcc
this.state = merchant.state
additionalProperties(merchant.additionalProperties)
}
/** Unique alphanumeric identifier for the payment card acceptor (merchant). */
fun acceptorId(acceptorId: String) = acceptorId(JsonField.of(acceptorId))
/** Unique alphanumeric identifier for the payment card acceptor (merchant). */
@JsonProperty("acceptor_id")
@ExcludeMissing
fun acceptorId(acceptorId: JsonField) = apply { this.acceptorId = acceptorId }
/** Unique numeric identifier of the acquiring institution. */
fun acquiringInstitutionId(acquiringInstitutionId: String) =
acquiringInstitutionId(JsonField.of(acquiringInstitutionId))
/** Unique numeric identifier of the acquiring institution. */
@JsonProperty("acquiring_institution_id")
@ExcludeMissing
fun acquiringInstitutionId(acquiringInstitutionId: JsonField) = apply {
this.acquiringInstitutionId = acquiringInstitutionId
}
/**
* City of card acceptor. Note that in many cases, particularly in card-not-present
* transactions, merchants may send through a phone number or URL in this field.
*/
fun city(city: String) = city(JsonField.of(city))
/**
* City of card acceptor. Note that in many cases, particularly in card-not-present
* transactions, merchants may send through a phone number or URL in this field.
*/
@JsonProperty("city")
@ExcludeMissing
fun city(city: JsonField) = apply { this.city = city }
/**
* Country or entity of card acceptor. Possible values are: (1) all ISO 3166-1 alpha-3
* country codes, (2) QZZ for Kosovo, and (3) ANT for Netherlands Antilles.
*/
fun country(country: String) = country(JsonField.of(country))
/**
* Country or entity of card acceptor. Possible values are: (1) all ISO 3166-1 alpha-3
* country codes, (2) QZZ for Kosovo, and (3) ANT for Netherlands Antilles.
*/
@JsonProperty("country")
@ExcludeMissing
fun country(country: JsonField) = apply { this.country = country }
/** Short description of card acceptor. */
fun descriptor(descriptor: String) = descriptor(JsonField.of(descriptor))
/** Short description of card acceptor. */
@JsonProperty("descriptor")
@ExcludeMissing
fun descriptor(descriptor: JsonField) = apply { this.descriptor = descriptor }
/**
* Merchant category code (MCC). A four-digit number listed in ISO 18245. An MCC is used
* to classify a business by the types of goods or services it provides.
*/
fun mcc(mcc: String) = mcc(JsonField.of(mcc))
/**
* Merchant category code (MCC). A four-digit number listed in ISO 18245. An MCC is used
* to classify a business by the types of goods or services it provides.
*/
@JsonProperty("mcc")
@ExcludeMissing
fun mcc(mcc: JsonField) = apply { this.mcc = mcc }
/** Geographic state of card acceptor. */
fun state(state: String) = state(JsonField.of(state))
/** Geographic state of card acceptor. */
@JsonProperty("state")
@ExcludeMissing
fun state(state: JsonField) = apply { this.state = state }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Merchant =
Merchant(
acceptorId,
acquiringInstitutionId,
city,
country,
descriptor,
mcc,
state,
additionalProperties.toImmutable(),
)
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Merchant && this.acceptorId == other.acceptorId && this.acquiringInstitutionId == other.acquiringInstitutionId && this.city == other.city && this.country == other.country && this.descriptor == other.descriptor && this.mcc == other.mcc && this.state == other.state && this.additionalProperties == other.additionalProperties /* spotless:on */
}
private var hashCode: Int = 0
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode = /* spotless:off */ Objects.hash(acceptorId, acquiringInstitutionId, city, country, descriptor, mcc, state, additionalProperties) /* spotless:on */
}
return hashCode
}
override fun toString() =
"Merchant{acceptorId=$acceptorId, acquiringInstitutionId=$acquiringInstitutionId, city=$city, country=$country, descriptor=$descriptor, mcc=$mcc, state=$state, additionalProperties=$additionalProperties}"
}
class Network
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Network && this.value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val INTERLINK = Network(JsonField.of("INTERLINK"))
val MAESTRO = Network(JsonField.of("MAESTRO"))
val MASTERCARD = Network(JsonField.of("MASTERCARD"))
val UNKNOWN = Network(JsonField.of("UNKNOWN"))
val VISA = Network(JsonField.of("VISA"))
fun of(value: String) = Network(JsonField.of(value))
}
enum class Known {
INTERLINK,
MAESTRO,
MASTERCARD,
UNKNOWN,
VISA,
}
enum class Value {
INTERLINK,
MAESTRO,
MASTERCARD,
UNKNOWN,
VISA,
_UNKNOWN,
}
fun value(): Value =
when (this) {
INTERLINK -> Value.INTERLINK
MAESTRO -> Value.MAESTRO
MASTERCARD -> Value.MASTERCARD
UNKNOWN -> Value.UNKNOWN
VISA -> Value.VISA
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
INTERLINK -> Known.INTERLINK
MAESTRO -> Known.MAESTRO
MASTERCARD -> Known.MASTERCARD
UNKNOWN -> Known.UNKNOWN
VISA -> Known.VISA
else -> throw LithicInvalidDataException("Unknown Network: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
@JsonDeserialize(builder = Pos.Builder::class)
@NoAutoDetect
class Pos
private constructor(
private val entryMode: JsonField,
private val terminal: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
fun entryMode(): PosEntryMode = entryMode.getRequired("entry_mode")
fun terminal(): PosTerminal = terminal.getRequired("terminal")
@JsonProperty("entry_mode") @ExcludeMissing fun _entryMode() = entryMode
@JsonProperty("terminal") @ExcludeMissing fun _terminal() = terminal
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Pos = apply {
if (!validated) {
entryMode().validate()
terminal().validate()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var entryMode: JsonField = JsonMissing.of()
private var terminal: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(pos: Pos) = apply {
this.entryMode = pos.entryMode
this.terminal = pos.terminal
additionalProperties(pos.additionalProperties)
}
fun entryMode(entryMode: PosEntryMode) = entryMode(JsonField.of(entryMode))
@JsonProperty("entry_mode")
@ExcludeMissing
fun entryMode(entryMode: JsonField) = apply { this.entryMode = entryMode }
fun terminal(terminal: PosTerminal) = terminal(JsonField.of(terminal))
@JsonProperty("terminal")
@ExcludeMissing
fun terminal(terminal: JsonField) = apply { this.terminal = terminal }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Pos =
Pos(
entryMode,
terminal,
additionalProperties.toImmutable(),
)
}
@JsonDeserialize(builder = PosEntryMode.Builder::class)
@NoAutoDetect
class PosEntryMode
private constructor(
private val card: JsonField,
private val cardholder: JsonField,
private val pan: JsonField,
private val pinEntered: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** Card presence indicator */
fun card(): Card = card.getRequired("card")
/** Cardholder presence indicator */
fun cardholder(): Cardholder = cardholder.getRequired("cardholder")
/** Method of entry for the PAN */
fun pan(): Pan = pan.getRequired("pan")
/** Indicates whether the cardholder entered the PIN. True if the PIN was entered. */
fun pinEntered(): Boolean = pinEntered.getRequired("pin_entered")
/** Card presence indicator */
@JsonProperty("card") @ExcludeMissing fun _card() = card
/** Cardholder presence indicator */
@JsonProperty("cardholder") @ExcludeMissing fun _cardholder() = cardholder
/** Method of entry for the PAN */
@JsonProperty("pan") @ExcludeMissing fun _pan() = pan
/** Indicates whether the cardholder entered the PIN. True if the PIN was entered. */
@JsonProperty("pin_entered") @ExcludeMissing fun _pinEntered() = pinEntered
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): PosEntryMode = apply {
if (!validated) {
card()
cardholder()
pan()
pinEntered()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var card: JsonField = JsonMissing.of()
private var cardholder: JsonField = JsonMissing.of()
private var pan: JsonField = JsonMissing.of()
private var pinEntered: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(posEntryMode: PosEntryMode) = apply {
this.card = posEntryMode.card
this.cardholder = posEntryMode.cardholder
this.pan = posEntryMode.pan
this.pinEntered = posEntryMode.pinEntered
additionalProperties(posEntryMode.additionalProperties)
}
/** Card presence indicator */
fun card(card: Card) = card(JsonField.of(card))
/** Card presence indicator */
@JsonProperty("card")
@ExcludeMissing
fun card(card: JsonField) = apply { this.card = card }
/** Cardholder presence indicator */
fun cardholder(cardholder: Cardholder) = cardholder(JsonField.of(cardholder))
/** Cardholder presence indicator */
@JsonProperty("cardholder")
@ExcludeMissing
fun cardholder(cardholder: JsonField) = apply {
this.cardholder = cardholder
}
/** Method of entry for the PAN */
fun pan(pan: Pan) = pan(JsonField.of(pan))
/** Method of entry for the PAN */
@JsonProperty("pan")
@ExcludeMissing
fun pan(pan: JsonField