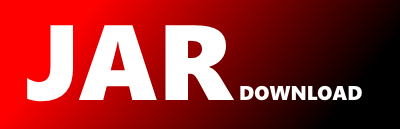
com.lithic.api.services.blocking.AuthStreamEnrollmentServiceImpl.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lithic-kotlin-core Show documentation
Show all versions of lithic-kotlin-core Show documentation
The Lithic Developer API is designed to provide a predictable programmatic
interface for accessing your Lithic account through an API and transaction
webhooks. Note that your API key is a secret and should be treated as such.
Don't share it with anyone, including us. We will never ask you for it.
The newest version!
// File generated from our OpenAPI spec by Stainless.
package com.lithic.api.services.blocking
import com.lithic.api.core.ClientOptions
import com.lithic.api.core.RequestOptions
import com.lithic.api.core.handlers.emptyHandler
import com.lithic.api.core.handlers.errorHandler
import com.lithic.api.core.handlers.jsonHandler
import com.lithic.api.core.handlers.withErrorHandler
import com.lithic.api.core.http.HttpMethod
import com.lithic.api.core.http.HttpRequest
import com.lithic.api.core.http.HttpResponse.Handler
import com.lithic.api.core.json
import com.lithic.api.errors.LithicError
import com.lithic.api.models.AuthStreamEnrollmentRetrieveSecretParams
import com.lithic.api.models.AuthStreamEnrollmentRotateSecretParams
import com.lithic.api.models.AuthStreamSecret
class AuthStreamEnrollmentServiceImpl
constructor(
private val clientOptions: ClientOptions,
) : AuthStreamEnrollmentService {
private val errorHandler: Handler = errorHandler(clientOptions.jsonMapper)
private val retrieveSecretHandler: Handler =
jsonHandler(clientOptions.jsonMapper).withErrorHandler(errorHandler)
/**
* Retrieve the ASA HMAC secret key. If one does not exist for your program yet, calling this
* endpoint will create one for you. The headers (which you can use to verify webhooks) will
* begin appearing shortly after calling this endpoint for the first time. See
* [this page](https://docs.lithic.com/docs/auth-stream-access-asa#asa-webhook-verification) for
* more detail about verifying ASA webhooks.
*/
override fun retrieveSecret(
params: AuthStreamEnrollmentRetrieveSecretParams,
requestOptions: RequestOptions
): AuthStreamSecret {
val request =
HttpRequest.builder()
.method(HttpMethod.GET)
.addPathSegments("v1", "auth_stream", "secret")
.putAllQueryParams(clientOptions.queryParams)
.replaceAllQueryParams(params.getQueryParams())
.putAllHeaders(clientOptions.headers)
.replaceAllHeaders(params.getHeaders())
.build()
return clientOptions.httpClient.execute(request, requestOptions).let { response ->
response
.use { retrieveSecretHandler.handle(it) }
.apply {
if (requestOptions.responseValidation ?: clientOptions.responseValidation) {
validate()
}
}
}
}
private val rotateSecretHandler: Handler = emptyHandler().withErrorHandler(errorHandler)
/**
* Generate a new ASA HMAC secret key. The old ASA HMAC secret key will be deactivated 24 hours
* after a successful request to this endpoint. Make a
* [`GET /auth_stream/secret`](https://docs.lithic.com/reference/getauthstreamsecret) request to
* retrieve the new secret key.
*/
override fun rotateSecret(
params: AuthStreamEnrollmentRotateSecretParams,
requestOptions: RequestOptions
) {
val request =
HttpRequest.builder()
.method(HttpMethod.POST)
.addPathSegments("v1", "auth_stream", "secret", "rotate")
.putAllQueryParams(clientOptions.queryParams)
.replaceAllQueryParams(params.getQueryParams())
.putAllHeaders(clientOptions.headers)
.replaceAllHeaders(params.getHeaders())
.apply { params.getBody()?.also { body(json(clientOptions.jsonMapper, it)) } }
.build()
clientOptions.httpClient.execute(request, requestOptions).let { response ->
response.use { rotateSecretHandler.handle(it) }
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy