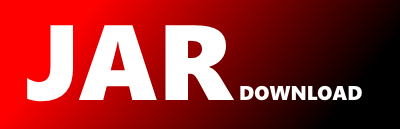
com.litongjava.jfinal.aop.process.ConfigurationAnnotaionProcess Maven / Gradle / Ivy
package com.litongjava.jfinal.aop.process;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Queue;
import com.litongjava.annotation.ABean;
import com.litongjava.annotation.Initialization;
import com.litongjava.jfinal.aop.Aop;
import com.litongjava.jfinal.aop.AopManager;
import com.litongjava.jfinal.model.DestroyableBean;
import com.litongjava.jfinal.model.MultiReturn;
import com.litongjava.jfinal.model.Pair;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class ConfigurationAnnotaionProcess {
/**
* 处理有和@Configuration注解类相似的逻辑
* @param configurationClass
* @param mapping
* @return
*/
public MultiReturn, List, Void> processConfiguration(
Queue> configurationClass, Map, Class extends Object>> mapping) {
// 边界处理
if (configurationClass == null || configurationClass.size() < 1) {
return null;
}
// 用于存储Bean方法及其类的信息
List>> beanMethods = new ArrayList<>();
List>> initializationMethods = new ArrayList<>();
for (Class> clazz : configurationClass) {
for (Method method : clazz.getDeclaredMethods()) {
if (method.isAnnotationPresent(ABean.class)) {
beanMethods.add(new Pair<>(method, clazz));
}
if (method.isAnnotationPresent(Initialization.class)) {
initializationMethods.add(new Pair<>(method, clazz));
}
}
}
// 2. 按照priority对beanMethods排序
beanMethods.sort(Comparator.comparingInt(m -> m.getKey().getAnnotation(ABean.class).priority()));
initializationMethods
.sort(Comparator.comparingInt(m -> m.getKey().getAnnotation(Initialization.class).priority()));
Queue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy