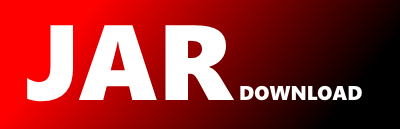
com.litongjava.tio.boot.http.handler.internal.HttpRequestFunctionHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tio-boot Show documentation
Show all versions of tio-boot Show documentation
Java High Performance Web Development Framework
package com.litongjava.tio.boot.http.handler.internal;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import com.litongjava.annotation.EnableCORS;
import com.litongjava.model.type.TioTypeReference;
import com.litongjava.tio.boot.http.utils.RequestActionUtils;
import com.litongjava.tio.http.common.HttpConfig;
import com.litongjava.tio.http.common.HttpRequest;
import com.litongjava.tio.http.common.HttpResponse;
import com.litongjava.tio.http.server.handler.IHttpRequestFunction;
import com.litongjava.tio.http.server.handler.RouteEntry;
import com.litongjava.tio.http.server.model.HttpCors;
import com.litongjava.tio.http.server.util.CORSUtils;
import com.litongjava.tio.utils.json.JsonUtils;
public class HttpRequestFunctionHandler {
@SuppressWarnings("unchecked")
public HttpResponse handleFunction(HttpRequest request, HttpConfig httpConfig, boolean compatibilityAssignment, RouteEntry, ?> routeEntry, String path) {
if (routeEntry == null) {
throw new RuntimeException("No route found for path: " + path);
}
IHttpRequestFunction, ?> function = routeEntry.getFunction();
TioTypeReference> typeReference = routeEntry.getTypeReference();
Type type = typeReference.getType();
Object result = null;
try {
// 处理数组类型
if (type == byte[].class) {
// 如果泛型 T 是 byte[]
byte[] body = request.getBody();
result = ((IHttpRequestFunction
© 2015 - 2024 Weber Informatics LLC | Privacy Policy