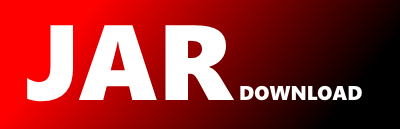
com.litongjava.tio.boot.http.handler.internal.TioBootHttpRequestDispatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tio-boot Show documentation
Show all versions of tio-boot Show documentation
Java High Performance Web Development Framework
package com.litongjava.tio.boot.http.handler.internal;
import java.io.File;
import java.io.FileFilter;
import java.lang.reflect.Method;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import org.apache.commons.io.monitor.FileAlterationMonitor;
import org.apache.commons.io.monitor.FileAlterationObserver;
import com.litongjava.constatns.ServerConfigKeys;
import com.litongjava.model.sys.SysConst;
import com.litongjava.tio.boot.exception.TioBootExceptionHandler;
import com.litongjava.tio.boot.http.TioRequestContext;
import com.litongjava.tio.boot.http.handler.controller.DynamicRequestController;
import com.litongjava.tio.boot.http.handler.controller.TioBootHttpControllerRouter;
import com.litongjava.tio.boot.http.session.SessionLimit;
import com.litongjava.tio.boot.http.utils.TioHttpControllerUtils;
import com.litongjava.tio.boot.server.TioBootServer;
import com.litongjava.tio.core.Tio;
import com.litongjava.tio.http.common.Cookie;
import com.litongjava.tio.http.common.HttpConfig;
import com.litongjava.tio.http.common.HttpMethod;
import com.litongjava.tio.http.common.HttpRequest;
import com.litongjava.tio.http.common.HttpResponse;
import com.litongjava.tio.http.common.RequestLine;
import com.litongjava.tio.http.common.handler.ITioHttpRequestHandler;
import com.litongjava.tio.http.common.session.HttpSession;
import com.litongjava.tio.http.server.handler.HttpRequestHandler;
import com.litongjava.tio.http.server.handler.RouteEntry;
import com.litongjava.tio.http.server.intf.HttpRequestInterceptor;
import com.litongjava.tio.http.server.intf.HttpSessionListener;
import com.litongjava.tio.http.server.intf.ThrowableHandler;
import com.litongjava.tio.http.server.model.HttpCors;
import com.litongjava.tio.http.server.router.HttpReqeustGroovyRouter;
import com.litongjava.tio.http.server.router.HttpRequestFunctionRouter;
import com.litongjava.tio.http.server.router.HttpRequestRouter;
import com.litongjava.tio.http.server.session.HttpSessionUtils;
import com.litongjava.tio.http.server.session.SessionCookieDecorator;
import com.litongjava.tio.http.server.stat.ip.path.IpPathAccessStats;
import com.litongjava.tio.http.server.stat.token.TokenPathAccessStats;
import com.litongjava.tio.http.server.util.CORSUtils;
import com.litongjava.tio.http.server.util.Resps;
import com.litongjava.tio.utils.SystemTimer;
import com.litongjava.tio.utils.cache.AbsCache;
import com.litongjava.tio.utils.cache.CacheFactory;
import com.litongjava.tio.utils.environment.EnvUtils;
import com.litongjava.tio.utils.hutool.ArrayUtil;
import com.litongjava.tio.utils.hutool.StrUtil;
import lombok.extern.slf4j.Slf4j;
/**
* @author litongjava
*/
@Slf4j
public class TioBootHttpRequestDispatcher implements ITioHttpRequestHandler {
protected HttpConfig httpConfig;
protected TioBootHttpControllerRouter httpControllerRouter = null;
private HttpRequestRouter httpRequestRouter;
private HttpReqeustGroovyRouter httpGroovyRouter;
private HttpRequestFunctionRouter httpRequestFunctionRouter;
private HttpRequestInterceptor httpRequestInterceptor;
private HttpSessionListener httpSessionListener;
private ThrowableHandler throwableHandler;
private SessionCookieDecorator sessionCookieDecorator;
private IpPathAccessStats ipPathAccessStats;
private TokenPathAccessStats tokenPathAccessStats;
private RequestStatisticsHandler requestStatisticsHandler;
private ResponseStatisticsHandler responseStatisticsHandler;
private StaticResourceHandler staticResourceHandler;
private HttpRequestHandler forwardHandler;
private HttpRequestHandler notFoundHandler;
private DynamicRequestController dynamicRequestController;
private HttpRequestFunctionHandler httpRequestFunctionHandler;
private AccessStatisticsHandler accessStatisticsHandler = new AccessStatisticsHandler();
private boolean printReport = EnvUtils.getBoolean(ServerConfigKeys.SERVER_HTTP_REQUEST_PRINTREPORT, false);
private boolean corsEnable = EnvUtils.getBoolean(ServerConfigKeys.SERVER_HTTP_RESPONSE_CORS_ENABLE, false);
private boolean printUrl = EnvUtils.getBoolean(ServerConfigKeys.SERVER_HTTP_REQUEST_PRINT_URL, false);
/**
* 静态资源缓存
*/
private AbsCache staticResCache;
/**
* 限流缓存
*/
private AbsCache sessionRateLimiterCache;
private String contextPath;
private int contextPathLength = 0;
private String suffix;
private int suffixLength = 0;
/**
* 赋值兼容处理
*/
private boolean compatibilityAssignment = true;
public void init(HttpConfig httpConfig, CacheFactory cacheFactory,
//
HttpRequestInterceptor defaultHttpServerInterceptorDispather,
//
HttpRequestRouter httpReqeustSimpleHandlerRoute, HttpReqeustGroovyRouter httpReqeustGroovyRoute,
//
HttpRequestFunctionRouter httpRequestFunctionRouter,
//
TioBootHttpControllerRouter tioBootHttpControllerRoutes,
//
HttpRequestHandler forwardHandler, HttpRequestHandler notFoundHandler,
//
RequestStatisticsHandler requestStatisticsHandler, ResponseStatisticsHandler responseStatisticsHandler,
//
StaticResourceHandler staticResourceHandler) {
this.httpControllerRouter = tioBootHttpControllerRoutes;
this.httpRequestInterceptor = defaultHttpServerInterceptorDispather;
this.httpRequestRouter = httpReqeustSimpleHandlerRoute;
this.httpGroovyRouter = httpReqeustGroovyRoute;
this.forwardHandler = forwardHandler;
this.notFoundHandler = notFoundHandler;
this.requestStatisticsHandler = requestStatisticsHandler;
this.responseStatisticsHandler = responseStatisticsHandler;
this.httpRequestFunctionRouter = httpRequestFunctionRouter;
if (httpConfig == null) {
throw new RuntimeException("httpConfig can not be null");
}
this.contextPath = httpConfig.getContextPath();
this.suffix = httpConfig.getSuffix();
if (StrUtil.isNotBlank(contextPath)) {
contextPathLength = contextPath.length();
}
if (StrUtil.isNotBlank(suffix)) {
suffixLength = suffix.length();
}
this.httpConfig = httpConfig;
if (httpConfig.getMaxLiveTimeOfStaticRes() > 0) {
long maxLiveTimeOfStaticRes = (long) httpConfig.getMaxLiveTimeOfStaticRes();
staticResCache = cacheFactory.register(DefaultHttpRequestConstants.STATIC_RES_CONTENT_CACHENAME, maxLiveTimeOfStaticRes, null);
}
sessionRateLimiterCache = cacheFactory.register(DefaultHttpRequestConstants.SESSION_RATE_LIMITER_CACHENAME, 60 * 1L, null);
if (httpConfig.getPageRoot() != null) {
try {
this.monitorFileChanged();
} catch (Exception e) {
e.printStackTrace();
}
}
if (staticResourceHandler == null) {
this.staticResourceHandler = new DefaultStaticResourceHandler();
} else {
this.staticResourceHandler = staticResourceHandler;
}
dynamicRequestController = new DynamicRequestController();
httpRequestFunctionHandler = new HttpRequestFunctionHandler();
}
/**
* 创建httpsession
*
* @return
*/
public HttpSession createSession(HttpRequest request) {
String sessionId = httpConfig.getSessionIdGenerator().sessionId(httpConfig, request);
HttpSession httpSession = new HttpSession(sessionId);
if (httpSessionListener != null) {
httpSessionListener.doAfterCreated(request, httpSession, httpConfig);
}
return httpSession;
}
/**
* @return the httpConfig
*/
public HttpConfig getHttpConfig(HttpRequest request) {
return httpConfig;
}
public HttpRequestInterceptor getHttpServerInterceptor() {
return httpRequestInterceptor;
}
/**
* @return the staticResCache
*/
public AbsCache getStaticResCache() {
return staticResCache;
}
/**
* 检查域名是否可以访问本站
*
* @param request
* @return
*/
private boolean checkDomain(HttpRequest request) {
String[] allowDomains = httpConfig.getAllowDomains();
if (allowDomains == null || allowDomains.length == 0) {
return true;
}
String host = request.getHost();
if (ArrayUtil.contains(allowDomains, host)) {
return true;
}
return false;
}
@Override
public HttpResponse handler(HttpRequest request) throws Exception {
request.setNeedForward(false);
// check domain
if (!checkDomain(request)) {
String remark = "Incorrect domain name" + request.getDomain();
Tio.remove(request.channelContext, remark);
HttpResponse httpResponse = new HttpResponse(request).setString(remark);
httpResponse.setKeepedConnectin(false);
return httpResponse;
}
long start = SystemTimer.currTime;
RequestLine requestLine = request.getRequestLine();
String path = requestLine.path;
if (StrUtil.isNotBlank(contextPath)) {
if (StrUtil.startWith(path, contextPath)) {
path = StrUtil.subSuf(path, contextPathLength);
}
}
if (StrUtil.isNotBlank(suffix)) {
if (StrUtil.endWith(path, suffix)) {
path = StrUtil.sub(path, 0, path.length() - suffixLength);
} else {
}
}
requestLine.setPath(path);
processCookieBeforeHandler(request, requestLine);
HttpResponse httpResponse = null;
// print url
if (printUrl) {
log.info("access:{}", requestLine.toString());
}
// limit
if (httpConfig.isUseSession() && EnvUtils.getBoolean("server.rate.limit.enable", true)) {
httpResponse = SessionLimit.check(request, path, httpConfig, sessionRateLimiterCache);
if (httpResponse != null) {
return httpResponse;
}
}
// options
if (requestLine.method.equals(HttpMethod.OPTIONS)) { // allow all OPTIONS request
httpResponse = new HttpResponse(request);
CORSUtils.enableCORS(httpResponse, new HttpCors());
return httpResponse;
}
// request
if (requestStatisticsHandler != null) {
requestStatisticsHandler.count(request);
}
requestLine = request.getRequestLine();
path = requestLine.path;
try {
TioRequestContext.hold(request);
// Interceptor
httpResponse = httpRequestInterceptor.doBeforeHandler(request, requestLine, httpResponse);
if (httpResponse != null) {
if (printReport) {
if (log.isInfoEnabled()) {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("\n-----------httpRequestInterceptor report---------------------\n");
stringBuffer.append("request:" + requestLine.toString()).append("\n")//
.append("interceptor:" + httpRequestInterceptor).append("\n")//
.append("response:" + httpResponse).append("\n")//
.append("\n");
log.info(stringBuffer.toString());
}
}
}
HttpRequestHandler httpRequestHandler = null;
if (httpResponse == null) {
// simpleHandlerRoute
httpRequestHandler = httpRequestRouter.find(path);
if (httpRequestHandler != null) {
if (printReport) {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("\n-----------httpRequestRouter report---------------------\n");
stringBuffer.append("request:" + requestLine.toString()).append("\n")//
.append("handler:" + httpRequestHandler.toString()).append("\n");
log.info(stringBuffer.toString());
}
httpResponse = httpRequestHandler.handle(request);
}
}
if (httpResponse == null) {
// groovyRoutes
if (httpGroovyRouter != null) {
httpRequestHandler = httpGroovyRouter.find(path);
if (httpRequestHandler != null) {
if (printReport) {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("\n-----------httpGroovyRoutes report---------------------\n");
stringBuffer.append("request:" + requestLine.toString()).append("\n")//
.append("handler:" + httpRequestHandler.toString()).append("\n");
log.info(stringBuffer.toString());
}
httpResponse = httpRequestHandler.handle(request);
}
}
}
if (httpResponse == null) {
// httpRequestFunctionRouter
if (httpRequestFunctionRouter != null) {
RouteEntry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy