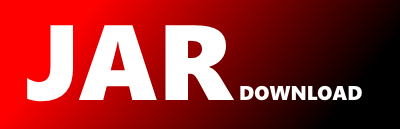
com.litongjava.tio.boot.http.utils.RequestActionUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tio-boot Show documentation
Show all versions of tio-boot Show documentation
Java High Performance Web Development Framework
package com.litongjava.tio.boot.http.utils;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.jfinal.template.Template;
import com.litongjava.model.http.response.ResponseVo;
import com.litongjava.tio.boot.http.TioRequestContext;
import com.litongjava.tio.boot.utils.TioAsmUtils;
import com.litongjava.tio.http.common.HttpConfig;
import com.litongjava.tio.http.common.HttpRequest;
import com.litongjava.tio.http.common.HttpResponse;
import com.litongjava.tio.http.common.session.HttpSession;
import com.litongjava.tio.http.server.util.ClassUtils;
import com.litongjava.tio.http.server.util.Resps;
import com.litongjava.tio.server.ServerChannelContext;
import com.litongjava.tio.utils.hutool.StrUtil;
import com.litongjava.tio.utils.json.JsonUtils;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class RequestActionUtils {
/**
*
* @param request
* @param response
* @param obj
* @return
*/
public static HttpResponse afterExecuteAction(Object actionRetrunValue) {
HttpRequest request = TioRequestContext.getRequest();
HttpResponse response = TioRequestContext.getResponse();
if (actionRetrunValue == null) {
return response;
}
if (actionRetrunValue instanceof HttpResponse) {
// action return http response
response = (HttpResponse) actionRetrunValue;
} else if (actionRetrunValue instanceof String) {
// action return string
response = Resps.txt(response, (String) actionRetrunValue);
} else if (actionRetrunValue instanceof Integer) {
response = Resps.txt(response, actionRetrunValue.toString());
} else if (actionRetrunValue instanceof Long) {
response = Resps.txt(response, actionRetrunValue.toString());
} else if (actionRetrunValue instanceof byte[]) {
response.setBody((byte[]) actionRetrunValue);
} else if (actionRetrunValue instanceof Template) {
// action return Template
Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy