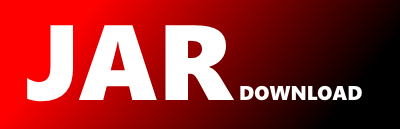
com.mybatis.jpa.statement.DefinitionStatementScanner Maven / Gradle / Ivy
package com.mybatis.jpa.statement;
import com.mybatis.jpa.annotation.InsertDefinition;
import com.mybatis.jpa.annotation.UpdateDefinition;
import org.apache.ibatis.builder.IncompleteElementException;
import org.apache.ibatis.builder.annotation.MethodResolver;
import org.apache.ibatis.session.Configuration;
import org.reflections.Reflections;
import org.reflections.scanners.MethodAnnotationsScanner;
import org.reflections.scanners.SubTypesScanner;
import org.reflections.scanners.TypeAnnotationsScanner;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
/**
* @author svili
**/
public class DefinitionStatementScanner {
private Configuration configuration;
private String[] basePackages;
private StatementBuildable statementBuilder;
private Set> registryAnnotationSet = new HashSet<>();
public void scan() {
for (String basePackage : basePackages) {
Reflections reflections = new Reflections(basePackage, new TypeAnnotationsScanner(), new SubTypesScanner(), new MethodAnnotationsScanner());
Set> registryAnnotation = registryAnnotationSet;
for (Class extends Annotation> annotation : registryAnnotation) {
Set methods = reflections.getMethodsAnnotatedWith(annotation);
for (Method method : methods) {
statementBuilder.parseStatement(method);
}
}
}
parsePendingMethods();
}
private void parsePendingMethods() {
Collection incompleteMethods = configuration.getIncompleteMethods();
synchronized (incompleteMethods) {
Iterator iter = incompleteMethods.iterator();
while (iter.hasNext()) {
try {
iter.next().resolve();
iter.remove();
} catch (IncompleteElementException e) {
// This method is still missing a resource
}
}
}
}
public static class Builder {
private DefinitionStatementScanner instance = new DefinitionStatementScanner();
public Builder() {
instance.registryAnnotationSet.add(InsertDefinition.class);
instance.registryAnnotationSet.add(UpdateDefinition.class);
}
public Builder configuration(Configuration configuration) {
this.instance.configuration = configuration;
return this;
}
public Builder basePackages(String[] basePackages) {
this.instance.basePackages = basePackages;
return this;
}
public Builder statementBuilder(StatementBuildable statementBuilder) {
this.instance.statementBuilder = statementBuilder;
return this;
}
public Builder registryAnnotationSet(Set> registryAnnotationSet) {
this.instance.registryAnnotationSet = registryAnnotationSet;
return this;
}
public DefinitionStatementScanner build() {
return this.instance;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy