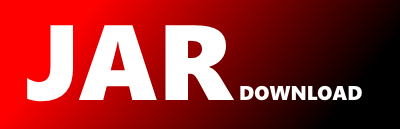
com.livefyre.entity.Subscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
Utilities for assistance with Livefyre features.
package com.livefyre.entity;
import java.util.Date;
import org.json.JSONObject;
public class Subscription {
private String to;
private String by;
private String type;
private Integer createdAt;
public Subscription() {}
public Subscription(String to, String by, Type type, Integer createdAt) {
this.to = to;
this.by = by;
this.type = type.name();
this.createdAt = createdAt;
}
public static Subscription serializeFromJson(JSONObject json) {
return new Subscription(json.getString("to"), json.getString("by"), Type.valueOf(json.getString("type")), json.getInt("createdAt"));
}
public Date createdAtDate() {
return new Date(createdAt.longValue()*1000);
}
/* Getters/Setters */
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
public String getBy() {
return by;
}
public void setBy(String by) {
this.by = by;
}
public String getType() {
return type;
}
public void setType(String type) {
Type.valueOf(type);
this.type = type;
}
public Integer getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Integer createdAt) {
this.createdAt = createdAt;
}
public enum Type {
personalStream;
private Type() {}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy