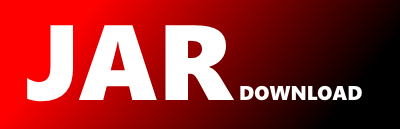
rb.templates.db_impl.erb Maven / Gradle / Ivy
<%#
# Copyright 2011 Rapleaf
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
%>
<%= autogenerated %>
package <%= root_package %>.impl;
import java.io.IOException;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Set;
import java.util.Collection;
import java.util.List;
import <%= root_package %>.I<%= db_name%>;
import <%= JACK_NAMESPACE %>.LazyLoadPersistence;
import <%= JACK_NAMESPACE %>.queries.GenericInsertion;
import <%= JACK_NAMESPACE %>.queries.GenericQuery;
import <%= JACK_NAMESPACE %>.queries.GenericUpdate;
import <%= JACK_NAMESPACE %>.queries.GenericDeletion;
import <%= JACK_NAMESPACE %>.BaseDatabaseConnection;
import <%= JACK_NAMESPACE %>.queries.Records;
import <%= JACK_NAMESPACE %>.queries.Column;
import <%= JACK_NAMESPACE %>.queries.QueryFetcher;
<% model_defns.each do |model_defn| %>
import <%= root_package %>.iface.<%= model_defn.iface_name %>;
<% end%>
import <%= project_defn.databases_namespace %>.IDatabases;
import com.rapleaf.jack.tracking.PostQueryAction;
public class <%=db_name%>Impl implements I<%=db_name%> {
private final BaseDatabaseConnection conn;
private final IDatabases databases;
private final PostQueryAction postQueryAction;
<% model_defns.each do |model_defn| %>
private final LazyLoadPersistence<<%= model_defn.iface_name %>, IDatabases> <%= model_defn.table_name %>;
<% end %>
private boolean allowBulkOperation = false;
public <%=db_name%>Impl(BaseDatabaseConnection conn, IDatabases databases, PostQueryAction postQueryAction) {
this.conn = conn;
this.databases = databases;
this.postQueryAction = postQueryAction;
<% model_defns.each do |model_defn| %>
this.<%= model_defn.table_name %> = new LazyLoadPersistence<>(conn, databases, <%= model_defn.impl_name %>::new);
<% end %>
}
public GenericInsertion.Builder createInsertion() {
return GenericInsertion.create(conn);
}
public GenericQuery.Builder createQuery() {
final GenericQuery.Builder builder = GenericQuery.create(conn);
builder.setPostQueryAction(postQueryAction);
return builder;
}
public GenericUpdate.Builder createUpdate() {
return GenericUpdate.create(conn, allowBulkOperation);
}
public GenericDeletion.Builder createDeletion() {
return GenericDeletion.create(conn, allowBulkOperation);
}
@Override
public Records findBySql(String statement, List> params, Collection columns) throws IOException {
final PreparedStatement preparedStatement = conn.getPreparedStatement(statement);
try {
for (int i = 0; i < params.size(); i++) {
final Object param = params.get(i);
final int paramIdx = i+1;
preparedStatement.setObject(paramIdx, param);
}
return QueryFetcher.getQueryResults(preparedStatement, columns, conn);
} catch (SQLException e) {
throw new IOException(e);
}
}
<% model_defns.each do |model_defn| %>
public <%= model_defn.iface_name %> <%= model_defn.persistence_getter %>{
return <%= model_defn.table_name %>.get();
}
<% end %>
public boolean deleteAll() throws IOException {
boolean success = true;
<% model_defns.each do |model_defn| %>
success &= <%= model_defn.persistence_getter %>.isEmpty() || <%= model_defn.persistence_getter %>.deleteAll();
<% end %>
return success;
}
public void disableCaching() {
<% model_defns.each do |model_defn| %>
<%= model_defn.table_name %>.disableCaching();
<% end %>
}
public void setAutoCommit(boolean autoCommit) {
conn.setAutoCommit(autoCommit);
}
public boolean getAutoCommit() {
return conn.getAutoCommit();
}
public void commit() {
conn.commit();
}
public void rollback() {
conn.rollback();
}
public void resetConnection() {
conn.resetConnection();
}
@Override
public void close() throws IOException {
conn.close();
}
@Override
public void setBulkOperation(boolean isAllowBulkOperation) {
this.allowBulkOperation = isAllowBulkOperation;
}
@Override
public boolean getBulkOperation() {
return allowBulkOperation;
}
public IDatabases getDatabases() {
return databases;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy