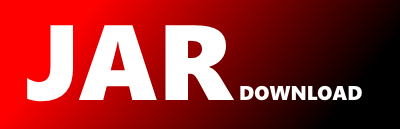
com.rapleaf.jack.queries.AbstractTable Maven / Gradle / Ivy
package com.rapleaf.jack.queries;
import java.util.Arrays;
import java.util.Set;
import com.google.common.base.Preconditions;
import com.google.common.base.Strings;
import com.google.common.collect.Sets;
import com.rapleaf.jack.AttributesWithId;
import com.rapleaf.jack.ModelWithId;
public class AbstractTable implements Table {
protected final String name;
protected final String alias;
protected final Class attributesType;
protected final Class modelType;
protected final Set allColumns;
protected AbstractTable(String name, String alias, Class attributesType, Class modelType) {
Preconditions.checkArgument(!Strings.isNullOrEmpty(name), "Table name cannot be null or empty.");
Preconditions.checkArgument(!Strings.isNullOrEmpty(alias), "Table alias cannot be null or empty.");
this.name = name;
this.alias = alias;
this.attributesType = attributesType;
this.modelType = modelType;
this.allColumns = Sets.newHashSet();
}
protected AbstractTable(AbstractTable table) {
this.name = table.name;
this.alias = table.alias;
this.attributesType = table.attributesType;
this.modelType = table.modelType;
this.allColumns = table.allColumns;
}
/**
* This method is used internally only. Users should call {@code Tbl#as}
* on each respective table to get an aliased reference.
*/
AbstractTable alias(String alias) {
return new AbstractTable<>(name, alias, attributesType, modelType);
}
@Override
public String getName() {
return name;
}
@Override
public String getAlias() {
return alias;
}
@Override
public Set getAllColumns() {
return allColumns;
}
@Override
public String getSqlKeyword() {
return name + " AS " + alias;
}
public Class getAttributesType() {
return attributesType;
}
public Class getModelType() {
return modelType;
}
public IndexedTable with(final IndexHint indexHint, final IndexHint... indexHints) {
Set indexHintList = Sets.newHashSet(indexHint);
indexHintList.addAll(Arrays.asList(indexHints));
return new IndexedTable<>(this, IndexHint.validate(indexHintList));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy