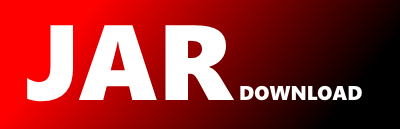
com.rapleaf.jack.store.field.JsEnumValueField Maven / Gradle / Ivy
package com.rapleaf.jack.store.field;
import java.util.function.BiFunction;
import java.util.function.Function;
import com.rapleaf.jack.store.JsRecord;
import com.rapleaf.jack.store.iface.InsertValue;
/**
* Convert enum to value using {@link Function} Enum -> Integer
* Convert value to enum using {@link Function} Integer -> Enum
*
* Value CAN be different from {@link Enum#ordinal}, e.g. thrift TEnum
*/
public class JsEnumValueField> extends AbstractJsField {
JsEnumValueField(String key, Function enumToValueFunction, Function valueToEnumFunction, InsertValue putMethod, BiFunction getMethod) {
super(key, convertPutMethod(enumToValueFunction, putMethod), convertGetMethod(valueToEnumFunction, getMethod));
}
private static InsertValue convertPutMethod(Function enumToValueFunction, InsertValue putMethod) {
return (executor, key, e) -> putMethod.apply(executor, key, enumToValueFunction.apply(e));
}
private static > BiFunction convertGetMethod(Function enumFunction, BiFunction getMethod) {
return (record, key) -> {
int enumValue = getMethod.apply(record, key);
return enumFunction.apply(enumValue);
};
}
}